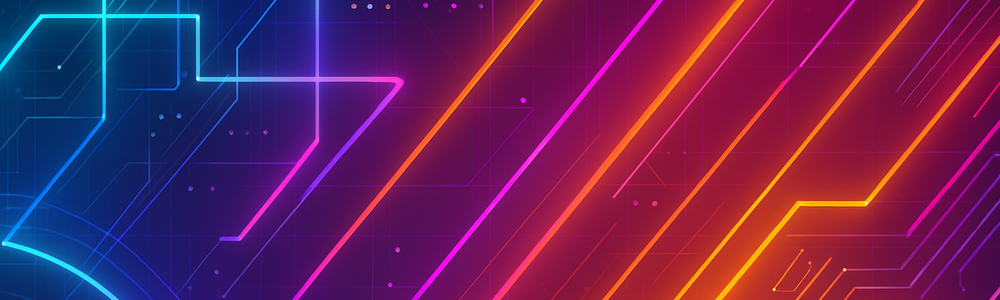
Operators are the unsung heroes of programming languages. They quietly power much of what happens behind the scenes in our code, performing essential tasks. In this post, we’ll take a closer look at Rust’s core operators. While many of them behave similarly to what you might be used to in other languages, it’s important to understand their nuances as you move toward Rust mastery.
In this post we’ll cover the following categories of Rust operators:
- arithmetic
- assignment
- equality
- comparison
- logical
- bitwise
- bitwise assignment
The following is the complete list of operators we’ll cover in this post. Some of them will receive more attention, particularly when introducing a new category. Once a category is introduced, the remaining operators in that group will be covered more briefly, since their general purpose and functionality should already be clear.
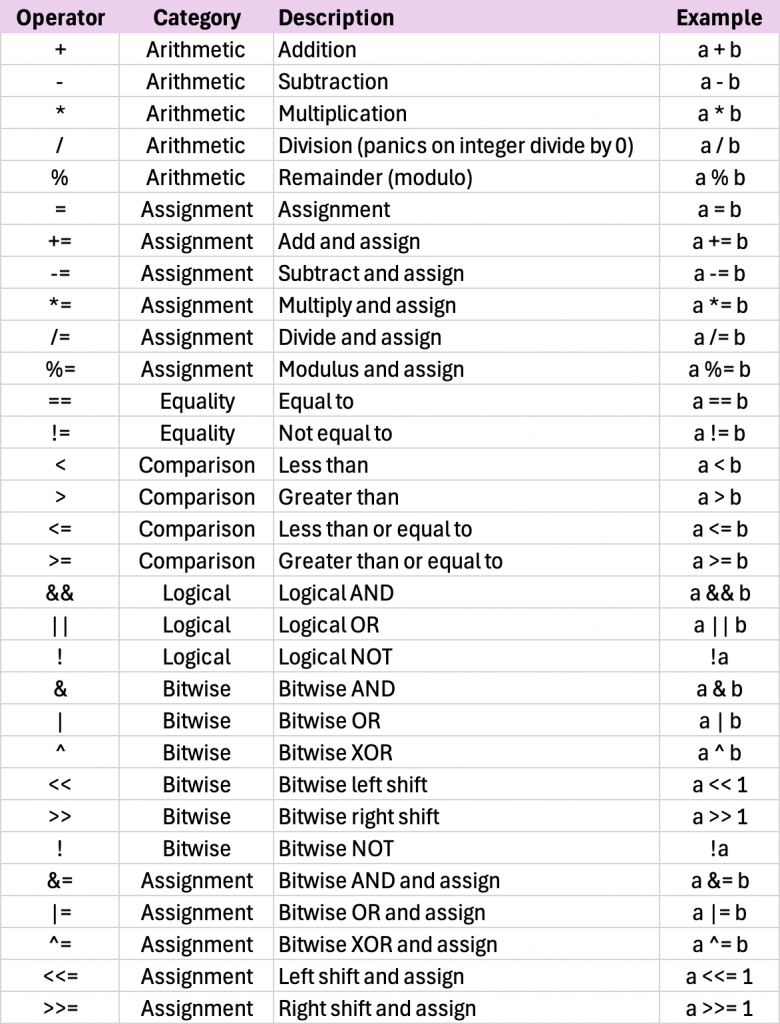
Arithmetic Operators
Arithmetic operators perform basic mathematical operations:
Addition (+
) – Adds two numbers.
Subtraction (-
) – Subtracts the right number from the left.
Multiplication (*
) – Multiplies two numbers.
Division (/
) – Divides the left number by the right.
Modulus (%
) – Returns the remainder of the division. For example:
10 % 3
equals 1
because 10 divided by 3 equals 3 with a remainder of 1.
10 % 4
equals 2
because 10 divided by 4 equals 2 with a remainder of 2.
Arithmetic operators work with all integer types (isize
, usize
, etc.) and floating-point types (f32
, f64
), but not with bool
or char
.
Operands must be of the same type, or you’ll encounter a type mismatch error. Use explicit conversions to fix this. The most common method is casting with as
, but be aware that it performs unchecked conversions that may truncate or wrap values.
Rust’s arithmetic operators (+
, -
, *
, /
, %
) may seem simple, but they hide some nuanced behavior. This post explores the types they work with, how conversions affect them, and how to handle overflow and division gracefully.
For floating-point types, %
performs truncating division, which may produce unexpected results when working with negative numbers. Use alternative methods if you need a true modulo for floats.
Casting with as
Casting with as
is the most common way to convert between types, but it can be unsafe. It may silently truncate or wrap around values, so use it with caution.
let x:i32 = 5;
let y: u32 = 10;
let z = x + y; // mismatched types error
let z = x + y as i32; // works fine
Supported conversions include:
- Integer ↔ Integer (
usize
,isize
, etc.) - Float ↔ Float
- Integer → Float
- Float → Integer (truncates decimal part)
When converting between signed and unsigned types or floating-point and integer types, as
may result in unexpected truncation, wraparound, or loss of precision. Prefer TryInto
or TryFrom
when possible.
Alternatives to Casting with as
TryInto
and TryFrom
return a Result
, allowing you to handle conversion errors gracefully and avoid silent truncation. These are fallible conversions.
Into
and From
are for infallible conversions when precision and safety are guaranteed.
Using as
for conversion can be unsafe and can silently truncate, so understand the implications of what you are dealing with, and consider the alternatives.
Integer Overflow
Integer overflow can lead to unexpected behavior, but Rust offers multiple strategies to handle it effectively:
Checked arithmetic returns None
if an overflow occurs:
let x: u8 = 255;
let result = x.checked_add(1); // None, as 255 + 1 overflows
Wrapping arithmetic wraps on overflow:
let x: u8 = 255;
let result = x.wrapping_add(1); // 0, wraps around
Saturating arithmetic saturates at the type’s maximum or minimum value on overflow.
let x: u8 = 255;
let result = x.saturating_add(1); // 255, saturated
Division by Zero
Integer division by zero panics, while floating-point division by zero returns NaN
or Infinity
.
is_nan()
→ Checks for NaN
is_infinite()
→ Checks for infinity (+∞ or -∞)
is_finite()
→ Checks for a finite value
Modulus Operator ( % )
The modulus operator (%
) behaves differently for integers and floats:
For integers, %
returns the remainder.
For floats, %
returns the remainder of division but not true modulo.
let x: i32 = 10;
let y: i32 = 3;
let remainder = x % y; // 1
let a: f64 = 10.5;
let b: f64 = 4.2;
let remainder_float = a % b; // 2.1
Floating Point Constants
Rust provides useful floating-point constants:
f32::NAN
, f64::NAN
f32::INFINITY
, f64::INFINITY
f32::NEG_INFINITY
, f64::NEG_INFINITY
Assignment Operators
Assignment operators are used to assign values to variables:
=
Assigns a value.
x = 5
assigns 5 to x
.
+=
Adds the right value to the left and assigns the result.
x += 3
is the same as x = x + 3
.
-=
Subtracts the right value from the left and assigns the result.
x -= 2
is the same as x = x - 2
.
*=
Multiplies the left value by the right and assigns the result.
x *= 4
is the same as x = x * 4
.
/=
Divides the left value by the right and assigns the result.
x /= 2
is the same as x = x / 2
.
%=
Takes the modulus of the left value with the right and assigns the result.
x %= 3
is the same as x = x % 3
.
The Rust assignment operators behave similarly to the arithmetic operators, with a few nuances.
Mutability
The left-hand side must be mutable.
Overflow Behavior
- For integer types, overflow occurs in debug mode, where Rust panics on overflow.
- In release mode, the behavior wraps around using two’s complement arithmetic.
To explicitly control overflow behavior, use #![deny(overflowing_literals)]
to prevent overflow-related errors in release mode.
Division and Modulo Edge Cases
Division by zero with /= or %= will panic at runtime.
Equality, Inequality, and Comparison Operators
In Rust, equality operators are used to compare two values and return a bool
(true
or false
).
Equal to ( ==
)
Checks if two values are equal.
fn main() {
println!("{}", 5 == 5); // true
println!("{}", 5 == 3); // false
}
Returns true
if the values are the same.
Returns false
if the values are different.
Not equal to (!=
)
Checks if two values are not equal.
fn main() {
println!("{}", 5 != 3); // true
println!("{}", 5 != 5); // false
}
Returns true
if the values are different.
Returns false
if the values are the same.
In Rust, both ==
and !=
require the types of the compared values to implement the PartialEq
trait. If the types don’t support comparison, the code won’t compile.
// Valid comparison
println!("{}", "hello" == "hello"); // true
// Invalid comparison - will not compile
// println!("{}", 5 == "5");
// Error: mismatched types
If you define custom structs or enums, you can implement the PartialEq
trait to enable comparison.
#[derive(PartialEq)]
struct Point {
x: i32,
y: i32,
}
fn main() {
let p1 = Point { x: 3, y: 4 };
let p2 = Point { x: 3, y: 4 };
println!("{}", p1 == p2); // true
}
When comparing references or pointers, ==
and !=
compare the values they point to.
fn main() {
let a = 5;
let b = &a;
println!("{}", a == *b); // true
}
In Rust, comparison operators compare two values and return a bool
(true
or false
).
Greater than ( >
)
Checks if the left value is greater than the right value.
fn main() {
println!("{}", 5 > 3); // true
println!("{}", 3 > 5); // false
}
Less than ( <
)
Checks if the left value is less than the right value.
fn main() {
println!("{}", 3 < 5); // true
println!("{}", 5 < 3); // false
}
Greater than or equal to ( >=
)
Checks if the left value is greater than or equal to the right value.
fn main() {
println!("{}", 5 >= 5); // true
println!("{}", 5 >= 3); // true
println!("{}", 3 >= 5); // false
}
Less than or equal to ( <=
)
Checks if the left value is less than or equal to the right value.
fn main() {
println!("{}", 5 <= 5); // true
println!("{}", 3 <= 5); // true
println!("{}", 5 <= 3); // false
}
Rust enforces type safety for comparisons. Both values must be of the same type or implement the PartialOrd
trait, which enables ordering comparisons.
// Valid comparison
println!("{}", 3.5 < 5.0); // true
// Invalid comparison - will not compile
// println!("{}", 5 < "5");
// Error: mismatched types
If you define custom structs or enums, you can implement the PartialOrd
and PartialEq
traits to allow comparisons.
#[derive(PartialEq, PartialOrd)]
struct Point {
x: i32,
y: i32,
}
fn main() {
let p1 = Point { x: 3, y: 4 };
let p2 = Point { x: 5, y: 6 };
println!("{}", p1 < p2); // true
}
References and Pointers:
When comparing references or pointers, Rust compares the values they point to.
fn main() {
let a = 5;
let b = &a;
println!("{}", a >= *b); // true
}
Logical Operators
In Rust, logical operators are used to combine or invert boolean expressions, returning a bool
(true
or false
).
Logical AND ( &&
)
Returns true
if both expressions are true
.
fn main() {
println!("{}", true && true); // true
println!("{}", true && false); // false
println!("{}", false && true); // false
println!("{}", false && false); // false
}
If either condition is false
, the result is false
.
Short-circuits: If the first condition is false
, the second condition is not evaluated.
Logical OR ( ||
)
Returns true
if at least one expression is true
.
fn main() {
println!("{}", true || false); // true
println!("{}", false || true); // true
println!("{}", false || false); // false
println!("{}", true || true); // true
}
Only returns false
if both conditions are false
.
Short-circuits: If the first condition is true
, the second condition is not evaluated.
Logical NOT ( !
)
Inverts the boolean value.
Converts true
to false
and false
to true
.
fn main() {
println!("{}", !true); // false
println!("{}", !false); // true
}
Short-Circuiting Behavior
AND (&&
) Short-circuits: If the first condition is false
, the second condition is not evaluated.
fn main() {
let x = false;
let result = x && {
println!("This won't print.");
true
};
println!("{}", result); // false
}
OR (||
) Short-circuits: If the first condition is true
, the second condition is not evaluated.
fn main() {
let x = true;
let result = x || {
println!("This won't print.");
false
};
println!("{}", result); // true
}
Logical Operators in if
Statements
Logical operators are often used to control program flow.
fn main() {
let x = 10;
let y = 5;
if x > 5 && y < 10 {
println!("Both conditions are true.");
}
if x < 5 || y < 10 {
println!("At least one condition is true.");
}
if !(x == 10) {
println!("x is not 10.");
} else {
println!("x is 10.");
}
}
Logical operators only work with boolean values (bool
). You cannot use non-boolean types in logical expressions.
// This will not compile
// let x = 5 && 10;
// Error: expected `bool`, found integer
Bitwise Operators
Bitwise operators work at the bit level and perform operations on individual bits of integer types (i32
, u32
, etc.). These operators return a modified integer after applying the operation.
Bitwise AND ( &
)
Performs a bitwise AND operation between two integers.
Each bit is set to 1
only if both corresponding bits in the operands are 1
.
fn main() {
let a = 5; // 0101 in binary
let b = 3; // 0011 in binary
println!("{}", a & b); // 1 (0001 in binary)
}
5 (0101)
3 (0011)
Result: 0001
(which is 1 in decimal).
Bitwise OR ( |
)
Performs a bitwise OR operation.
Each bit is set to 1
if either of the corresponding bits in the operands is 1
.
fn main() {
let a = 5; // 0101 in binary
let b = 3; // 0011 in binary
println!("{}", a | b); // 7 (0111 in binary)
}
5 (0101)
3 (0011)
Result: 0111
(which is 7 in decimal).
Bitwise XOR ( ^
)
Performs a bitwise XOR (exclusive OR).
Each bit is set to 1
if only one of the corresponding bits is 1
, but not both.
fn main() {
let a = 5; // 0101 in binary
let b = 3; // 0011 in binary
println!("{}", a ^ b); // 6 (0110 in binary)
}
5 (0101)
3 (0011)
Result: 0110
(which is 6 in decimal).
Bitwise NOT ( !
)
Inverts all bits of an integer.
Converts 1
to 0
and 0
to 1
.
Applies two’s complement for signed integers, which results in -(n + 1)
.
fn main() {
let a = 5; // 0101 in binary
println!("{}", !a); // -6
}
!5
inverts 0101
to 1010
(two’s complement gives -6
).
Bitwise NOT inverts all bits, producing a two’s complement result for signed types. For unsigned types, it flips the bits without affecting the sign.
Left Shift ( <<
)
Shifts the bits to the left by a specified number of positions.
Each left shift multiplies the number by 2
to the power of the shift amount.
fn main() {
let a = 5; // 0101 in binary
println!("{}", a << 1); // 10 (1010 in binary)
println!("{}", a << 2); // 20 (10100 in binary)
}
5 << 1
shifts the bits to the left: 0101
→ 1010
→ 10.
5 << 2
shifts the bits again: 10100
→ 20.
Shifting by an amount equal to or greater than the bit-width of the type (e.g., 32
for u32
) will panic in debug mode and result in undefined behavior in release mode.
Right Shift ( >>
)
Shifts the bits to the right by a specified number of positions.
Zero-fill shift (>>
) for unsigned types (u32
), filling with 0
s.
Sign-extended shift (>>
) for signed types (i32
), preserving the sign bit.
fn main() {
let a = 5; // 0101 in binary
println!("{}", a >> 1); // 2 (0010 in binary)
let b: i32 = -5;
println!("{}", b >> 1); // -3 (sign-extended)
}
5 >> 1
shifts 0101
to 0010
→ 2.
-5
(in binary) is represented using two’s complement, so shifting right preserves the sign.
Right shifts for signed integers use arithmetic shifting, preserving the sign bit. Unsigned types use logical shifting, filling with zeros.
Bitwise operations are extremely fast and are often used for low-level system programming, cryptography, and graphics processing.
Bitwise Assignment Operators
You can also combine bitwise operators with assignment.
Bitwise AND and assign ( &=
)
Bitwise OR and assign ( |=
)
Bitwise XOR and assign ( ^=
)
Left shift and assign ( <<=
)
Right shift and assign ( >>=
)
fn main() {
let mut a = 5;
a &= 3; // a = a & 3
println!("{}", a); // 1
a |= 2; // a = a | 2
println!("{}", a); // 3
a ^= 1; // a = a ^ 1
println!("{}", a); // 2
a <<= 1; // a = a << 1
println!("{}", a); // 4
a >>= 1; // a = a >> 1
println!("{}", a); // 2
}
Bitwise operations can only be performed on integer types. They won’t work with floating-point numbers or non-integer types.
Left shifts (<<
) and right shifts (>>
) behave differently for signed and unsigned types.
Right shifts preserve the sign for signed integers, filling with the sign bit.
Bitwise operations are extremely fast and are often used for low-level system programming, cryptography, and graphics processing.
Operators Missing in Rust
Note that Rust does not have the following commonly found in some programming languages:
- increment ( ++ ) and decrement ( — ) operators
- ternary expression:
condition ? expr1 : expr2
These features are intentionally omitted in Rust. The increment and decrement operators are potentially confusing because they offer prefix and postfix versions and can introduce subtle bugs. Rust prefers explicitness and omitting ++ and — forces developers to be more explicit.
Also, variables in Rust are immutable by default, and the ++ and — operators are inherently mutation operators. Allowing them would violate a core principle in Rust.
Rust omits the ternary expression, preferring the a concise version of if - else
for readability.
Operators are a standard feature of every programming language. This post may serve as a handy reference on the subtleties of the operators Rust offers.
Thanks for stopping by, and for including ByteMagma in your Rust mastery journey!
Leave a Reply