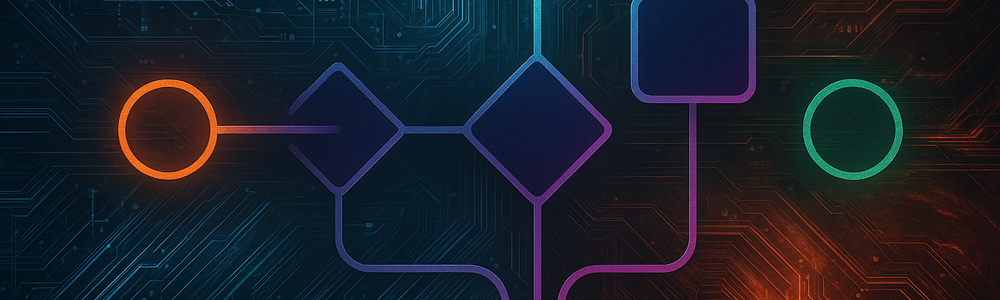
Computer programs often need to check conditions and make decisions, then follow different code flows based on the results. Rust has several constructs for controlling code flow that are similar to other languages. Rust also has some features that are unique.
In this post, we’ll examine the Rust features enabling developers to create complex programs allowing code to branch and execute alternate code flows.
The equality, inequality, comparison and logical operators are used extensively in conditional code. If you need a refresher, check out the ByteMagma blog post on Operators in Rust.
Conditional Code Flow
We begin our examination of code flow in Rust with conditionals. Like most programming languages, Rust has the if else
construct as a basic conditional pattern.
Let’s jump right in and get started writing some code.
Open a shell window (Terminal on Mac/Linux, Command Prompt or PowerShell on Windows). Then navigate to the directory where you store Rust packages
for this blog series, and run the following command:
cargo new conditionals
Next, change into the newly created conditionals directory and open it in VS Code (or your favorite IDE).
Note: Using VS Code is highly recommended for following along with this blog series. Be sure to install the Rust Analyzer extension — it offers powerful features like code completion, inline type hints, and quick fixes.
Also, make sure you’re opening the conditionals directory itself in VS Code. If you open a parent folder instead, the Rust Analyzer extension might not work properly — or at all.
Now, open the file src/main.rs and replace its contents entirely with the following code.
fn main() {
let x = 10;
if x < 5 {
println!("It's true! {} is less than 5!", x);
} else {
println!("It's false! {} is NOT less than 5!", x);
}
}
Now execute cargo run and you should see this output in the shell window:
It's false! 10 is NOT less than 5!
The if
construct starts with the if
keyword, then an expression that evaluates to true or false (a boolean expression), and then curly braces surrounding the code that should execute if the expression evaluates to true.
If the boolean condition evaluates to false, the code block does not execute.
The construct can have an else
as well, and its code block will be executed if the boolean expression evaluates to false.
Change the assignment to x:
let x = 3;
Execute cargo run again and you should see this:
It's true! 3 is less than 5!
This is your basic if else
conditional in Rust. It operates the same as many other programming languages.
One difference is that enclosing the conditional expression in parentheses is optional in Rust:
if (x < 5) {
println!("It's true! {} is less than 5!", x);
} else {
println!("It's false! {} is NOT less than 5!", x);
}
If you make this change and run the code again you’ll get a compiler warning.
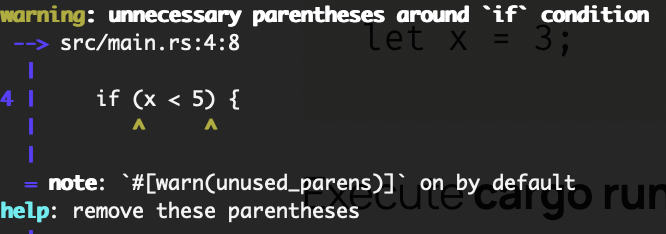
It’s a best practice in Rust to omit parentheses around if
conditions. Adding them is valid but will result in a warning about unnecessary parentheses.
Rust conditionals also have the if else if else
construct, like other languages:
fn main() {
let x = 100;
if x >= 90 {
println!("Great score, you got a {}", x);
} else if x >= 80 && x < 90 {
println!("Pretty good, you got a {}", x);
} else if x >= 70 && x < 80 {
println!("Hmm... looks like you need to study more, you got a {}", x);
} else if x >= 60 && x < 70 {
println!("Ouch, you should get a tutor, you got a {}", x);
} else {
println!("Yo, stop bingeing on Netflix, you got a {}", x);
}
}
Run this code several times, substituting these values for x each time:
100 89 79 69 59
You should see the various conditional branches being executed:
Great score, you got a 100
Pretty good, you got a 89
Hmm... looks like you need to study more, you got a 79
Ouch, you should get a tutor, you got a 69
Yo, stop bingeing on Netflix, you got a 59
The first condition in this complex conditional that evaluates to true will have its code block executed, and if none of the conditions evaluate to true, then the else
code block will execute.
Note that the conditional expression can be quite complex depending on the situation, or it could be the result of a function call, etc. If however the logic in the conditional gets too complex, you might want to take a moment to consider if your overall code could be designed differently.
Ternary-Like Expressions
Some language have a concise ternary expression that goes something like this JavaScript code:
var discount = isWeekend() ? .20 : 0;
In this ternary expression, if the isWeekend() function returns true, then the discount variable is assigned a value of .20, which could be the 20% discount applied to a purchase made on the weekend. If it’s a weekday then there is no discount.
Rust has a ternary-like expression that operates similar to the above ternary expression.
let result = if condition { value_if_true } else { value_if_false };
Here’s an example:
fn main() {
let x = 10;
let is_positive = if x > 0 { "Positive" } else { "Non-positive" };
println!("{}", is_positive);
}
The output of this code is:
Positive
Since if
is an expression in Rust, it evaluates to a value that can be directly assigned to a variable or returned from a function. This makes it just as concise and effective as a ternary operator in other languages.
Pattern Matching
Rust has a match
construct that is very similar to the switch construct found in other languages. However the Rust match construct is much more flexible and powerful.
Basic Match
Here is a basic match expression. Replace the code in main.rs and execute cargo run:
enum Direction {
North,
South,
East,
West,
}
fn describe_direction(dir: Direction) {
match dir {
Direction::North => println!("Heading North"),
Direction::South => println!("Going South"),
Direction::East => println!("Moving toward East"),
Direction::West => println!("Going West!!!"),
}
}
fn main() {
describe_direction(Direction::West);
describe_direction(Direction::South);
describe_direction(Direction::North);
describe_direction(Direction::East);
}
If you’re not familiar with Rust enums, check out the ByteMagma post on enums.
Here’s the output of the above code.
Going West!!!
Going South
Heading North
Moving toward East
You start with the match keyword, followed by the expression to match. In the above code we match on the dir parameter passed into the describe_direction() function. Then the body of the match expression is enclosed in curly braces.
Inside the match code block are arms. Each match arm begins with a pattern to match, followed by a fat arrow => and then an expression, the result returned by the match expression. You can optionally have curly braces for a code block to execute for a match on that arm’s pattern.
In the code above the match arm patterns are the Direction enum variants, North, South, East and West. Each arm has a println!() expression that executes for its pattern. The arms will compare their pattern with the match expression dir.
Match Arms must be Exhaustive
An important requirement of the match construct is that your match arms must be exhaustive, unless you use a catch-all placeholder, which we’ll see in a moment. The compiler knows the Direction enum has four variants, so your match expression, which matches on a Direction instance, must have arms for all the Direction variants, otherwise you’ll get an error.
Remove the last match arm so your match expression looks like this and execute cargo run:
match dir {
Direction::North => println!("Heading North"),
Direction::South => println!("Going South"),
Direction::East => println!("Moving toward East"),
}
You should get a compiler error similar to this:

The _
catch-all pattern is used to match any values that are not explicitly handled, ensuring that the match arms remain exhaustive:
match dir {
Direction::North => println!("Heading North"),
Direction::South => println!("Going South"),
Direction::East => println!("Moving toward East"),
_ => println!("Moving somewhere else"),
}
Now if dir matches anything other than North, South or East the catch-all arm will handle the match.
Matching Multiple Values
Match patterns are highly flexible. Replace the code in main.rs with the following and execute cargo run:
fn numbers(x: i32) {
match x {
1 | 2 => println!("One or Two"),
3..6 => println!("Three to Five"),
6..=8 => println!("Six to Eight"),
_ => println!("Something else"),
}
}
fn main() {
numbers(1);
numbers(2);
numbers(4);
numbers(7);
numbers(10);
}
Output:
One or Two
One or Two
Three to Five
Six to Eight
Something else
The match arms will compare their patterns with the match expression x.
In the above code, the first arm makes use of the OR operator to match on 1 or 2. The second and third arms use Rust ranges to match on numbers 3 – 5 or 6 – 8.
Range 3..6 matches numbers 3 – 5 but doesn’t include the ending number 6. Range 6..=8 matches numbers 6 – 8 and because we use the = symbol it matches 8 as well.
Destructuring a Struct
Here’s an example of destructuring a struct, and matching on the struct fields. If you haven’t learned about Rust structs yet, you can refer to our ByteMagma post Structs in Rust: Modeling Real-World Data.
struct Point {
x: i32,
y: i32,
}
fn main() {
let p = Point { x: 3, y: 7 };
match p {
Point { x, y: 0 } => println!("On the x-axis at {}", x),
Point { x: 0, y } => println!("On the y-axis at {}", y),
Point { x, y } => println!("Point at ({}, {})", x, y),
}
}
Output:
Point at (3, 7)
In this code we define a struct Point having two i32 fields, x and y. We create an instance of the Point struct named p, giving the x field a value of 3 and the y field a value of 7. Then we match on p, the instance of the Point struct.
The match arms will compare their patterns with the match expression p. Here is the first match arm:
Point { x, y: 0 } => println!("On the x-axis at {}", x),
The left of the fat arrow =>
is the pattern used to destructure and match the fields of the Point
struct. If the pattern matches, the associated code block executes.
Then the code to the right of the fat arrow => uses the extracted values. In this case it uses the extracted x value and ignores the extracted y value in a println!() expression.
Point instance p has an x value of 3 and a y value of 7.
The first match arm pattern expects any x value and a y value of 0. Because the pattern y value 0 does not match the p y value 7, that arm is ignored and the next match arm is evaluated.
The second match arm pattern expects a x value of 0 and any y value. Because the pattern x value 0 does not match the p x value 3, that arm is ignored and the next match arm is evaluated.
The third match arm pattern expects any x value and any y value. So this arm’s pattern matches and the output is printed.
Note that because this third match arm pattern matches any value for x and for y, this is the same as the _ catch all pattern. Because match arms must be exhaustive, the compiler is happy.
Destructuring a Tuple
Here’s an example of destructuring a tuple, and matching on the tuple elements. If you haven’t learned about Rust tuples yet, you can refer to our ByteMagma post Rust Variables and Data Types.
fn main() {
let point = (3, 5, 8);
match point {
(0, y, z) => println!("Point on the x-axis at y = {}, z = {}", y, z),
(x, 0, z) => println!("Point on the y-axis at x = {}, z = {}", x, z),
(x, y, 0) => println!("Point on the z-axis at x = {}, y = {}", x, y),
(x, y, z) => println!("Point at ({}, {}, {})", x, y, z),
}
}
Output:
Point at (3, 5, 8)
In this code, rather than representing a point with a struct, we use a tuple with three elements for the three coordinates of a point. We create a tuple point with values 3, 5, and 8.
Then we match on the tuple point. All four arms of the match expression destructure the match expression point into variables x, y, and z. Note that in the previous example, the match arm patterns must use the field names x and y, because we destructured a struct that has fields of x and y.
Since tuple elements are unnamed, you can choose any name when destructuring them in a match arm. These names are temporary bindings for use within the pattern.
fn main() {
let point = (3, 5, 8);
match point {
(0, my_y, and_z) => println!("Point on the x-axis at y = {}, z = {}", my_y, and_z),
(x_elem, 0, z_elem) => println!("Point on the y-axis at x = {}, z = {}", x_elem, z_elem),
(x, y, 0) => println!("Point on the z-axis at x = {}, y = {}", x, y),
(x_data, y_elem, z_last) => println!("Point at ({}, {}, {})", x_data, y_elem, z_last),
}
}
You can name them whatever you want as long the names match in the pattern to the left of the fat arrow => and in the code to the right of the fat arrow.
Destructuring an Enum
Here’s an example of destructuring an enum, and matching on the enum variants. If you haven’t learned about Rust enums yet, you can refer to our ByteMagma post Rust Enums: Close Cousins of Structs.
enum Shape {
Circle { radius: f64 },
Rectangle { width: f64, height: f64 },
Triangle(f64, f64, f64),
}
fn main() {
let shape = Shape::Rectangle { width: 10.0, height: 5.0 };
match shape {
Shape::Circle { radius } => println!("Circle with radius {}", radius),
Shape::Rectangle { width, height } => println!("Rectangle with width {} and height {}", width, height),
Shape::Triangle(a, b, c) => println!("Triangle with sides {}, {}, and {}", a, b, c),
}
}
Output:
Rectangle with width 10 and height 5
In this code we create a Shape enum with three variants:
- Circle – struct-like value, holds one field, the radius of the circle
- Rectangle – struct-like value, holds two values, the width and height of the rectangle
- Triangle – tuple-like value, holds three unnamed values for the sides of the triangle
Note that the Circle and Rectangle variants hold struct-like data. Struct-like data have named fields, but it is not a struct. The Triangle variant holds tuple-like data. Tuple-like data have one or more elements that are unnamed.
We create an instance of the Rectangle variant, shape, and then we match on it. The match arms check if shape is an instance of the Circle enum variant, the Rectangle variant, or the Triangle variant.
Once again, in the match arm pattern we extract the data. For the Circle and Rectangle variants we need to use the field names, radius, width and height, but for Triangle we could name the variables anything.
Match with Guards (using if in patterns)
A match guard is an optional if
condition that adds extra criteria to a match
arm. It allows you to refine a pattern match by adding logic that must evaluate to true
for the arm to execute.
fn main() {
let age = 18;
match age {
n if n < 18 => println!("Underage"),
n if n == 18 => println!("Just turned 18!"),
_ => println!("Adult"),
}
}
Output:
Just turned 18!
Here we define a variable age and initialize it with a value of 18. Then we match on age.
The first two match arms are examples of match guards. Then declare a variable n, and then use an if
to check the value of n against a condition. If the value of n is less than 18, we print Underage, if equal to 18, we print Just turned 18!. For all other values of age we print Adult.
Match guards are another example of the awesome flexibility of match arm patterns!
Matching References with &
Sometimes we need to be careful not to take ownership of a value we are matching on, but to instead borrow the value. If you are new to ownership, move and borrow in Rust, check out our ByteMagma post Ownership, Moving, and Borrowing in Rust.
fn main() {
let x = Some(5);
// Matching against a reference to x
match &x {
Some(val) => println!("Value is {}", val),
None => println!("No value"),
}
}
Output:
Value is 5
In this code, we define the variable x
, which is an instance of the Some
variant of the Option
enum.” If you haven’t learned about Rust enums yet, you can refer to our ByteMagma post Rust Enums: Close Cousins of Structs.
To avoid moving the value, we match against a reference using &x
. This ensures that x
remains available for use after the match expression.
So in the first match arm, Some(val) is &x which is a reference to Some(5). We could have named the variable anything, such as the_passed_in_value, but we named it val here.
Some people get confused when the println!() outputs Value is 5 and not Value is &Some(5). This is because the println!() macro dereferences the &Some(5) reference and gets the 5. It can do this because here the 5 is an i32 primitive value, which implements the Display trait. We’ll discuss traits in detail in a future post.
The match expression has a second arm that handles the Option None variant. Remember, the match arms must be exhaustive, and because the Option enum only has the two variants Some() and None, our match arms handle both.
Pattern Matching an Array
You can also match patterns on an array:
fn main() {
let arr = [1, 2, 3];
match arr {
[1, _, 3] => println!("Pattern matched"),
_ => println!("Different array"),
}
}
Output:
Pattern matched
Here we have an array of three elements. Our first match arm checks for a pattern of an array of three elements whose first element is a 1 and whose third element is a 3. The _ (underscore) in the pattern allows us to ignore the second element, so the second element could be 100, or 8, or 2 and because we ignore it, it won’t affect our matching the pattern.
The second arm is our catch-all that matches all other arrays, allowing our match arms to be exhaustive.
Matching on Array Slices
We can also match on array slices:
fn main() {
let nums = [1, 2, 3, 4, 5];
match nums {
[1, 2, .., 5] => println!("Starts with 1, 2 and ends with 5"),
_ => println!("No match"),
}
}
Output:
Starts with 1, 2 and ends with 5
This code has an array of five elements. Our first match arm checks that the first two array elements are 1 and 2, and the last array element is a 5. This pattern works with slices, where the length may be variable. For arrays of a known length, the pattern must match exactly.
Using @
Bindings in Patterns
@
is part of pattern matching syntax and is referred to as a binding pattern.
fn main() {
let num = Some(5);
match num {
Some(n @ 1..=10) => println!("Matched a small number: {}", n),
Some(n) => println!("Matched something else: {}", n),
None => println!("No match"),
}
}
The first match arm is a concise way of saying “if num a Some() with a value within the range 1 to 10 (including 10), then assign the value of num to variable n”.
If the Some() value of num is not within that range then that arm does not match and the next arm is checked.
The second arm matches other values of num for Some() that actually have a value, and the third arm matches cases where num does not have a value, so our match is exhaustive.
matches!
Macro for Simple Pattern Checks
Rust also includes the matches!() macro for simple pattern checks.
fn main() {
let x = Some(5);
if matches!(x, Some(5)) {
println!("Matched 5!");
}
}
Output:
Matched 5!
We have only one thing to check, does is x a Some(5) value.
Return Values from match Expressions
The match
expression returns a value that can either be assigned to a variable or returned from a function if it’s the last expression.
This post introduced the Rust if else and match constructs for conditional code flow.
if else is similar to implementations in other languages, and match is similar to switch constructs in other languages. We also saw a Rust if else construct similar to the ternary common to other languages.
We also saw the many variations of match expressions for pattern matching, illustrating how flexible and powerful Rust’s pattern matching is.
Thank you very much for stopping by and learning more about Rust conditionals and pattern matching!
Leave a Reply