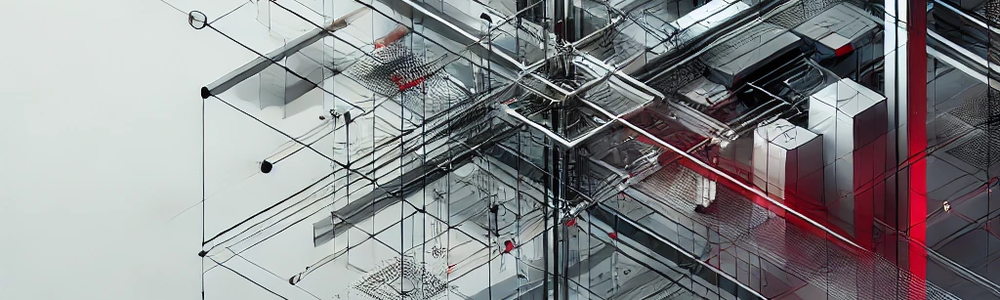
When your program outgrows simple variables and needs to represent real-world things — like users, products, or game characters — it’s time to reach for structs.
In this post, we’ll explore how Rust’s structs let you bundle related data together, define custom types, and even attach behavior with methods and associated functions.
If you’re just getting started with Rust, these posts earlier in this series on Rust programming may be helpful:
Setting Up Your Rust Development Environment
Mutability, Copying, and Why Some Things Just Vanish
Functions in Rust – The Building Blocks of Reusable Code
Ownership, Moving, and Borrowing in Rust
Introduction
A struct in Rust lets you group related data into a single, custom data type — a key tool for modeling real-world entities. Beyond just storing data, Rust also allows you to define methods and associated functions that operate on these structs, giving them behavior as well as structure.
Rust structs can be loosely compared to C++ classes, but with some important differences. For example, Rust does not support inheritance in the traditional object-oriented sense. Instead, Rust encourages composition over inheritance — combining fields and traits to build flexible, reusable abstractions.
Defining a Struct
Structs are similar to tuples, which we touched on in our post about Rust variables and data types. Both can hold multiple, related values of different types.
However, a key difference is that structs name each piece of data (called a field), whereas tuple elements are accessed only by their position. This naming makes structs much easier to understand and work with.
Instead of remembering that .0 is a name and .1 is an age in a tuple, you can just write person.name and person.age. It makes your code easier to read and reason about.
To define a struct, we start with the struct keyword, followed by the name (identifier). The struct’s name should describe the significance or purpose of the struct, what its data fields will represent. Examples include:
- User
- Vehicle
- Shape
- Request
Following the struct’s name are curly braces, which enclose the body of the struct, where the names and types of the data fields are defined. Specifying the data types of a struct’s fields is required, as it helps the compiler generate efficient and safe code.
Here is an example of a Customer struct and its fields:
struct Customer {
first_name: String,
last_name: String,
email: String,
phone: String,
address_1: String,
address_2: String,
city: String,
state: String,
zip_code: String,
}
In this example, each field is a String — an owned string type. This is preferred because the Customer struct owns the data.
If the data came from somewhere else and was only temporarily borrowed, we might use &str instead. But for struct fields that are part of the struct’s long-term data, String is the right choice.
Struct names in Rust use PascalCase, where each word is capitalized with no underscores (e.g., Customer, UserAccount).
Field names use snake_case, which means all lowercase letters with underscores between words (e.g., first_name, zip_code). This helps distinguish struct types from fields and follows Rust’s style guide.
Notice the comma after the final field zip_code. This is idiomatic in Rust and applies to many situations:
- struct definitions
- function arguments
- match arms
- array literals
- enum variants
Reasons to use a trailing comma:
- Cleaner version control diffs (only the new line changes)
- Avoids syntax errors when adding new items
- Automatically added by rustfmt, Rust’s formatting tool
Creating Struct Instances
Let’s get started writing some code.
Open a shell window (Terminal in Mac/Linux, Cmd or PowerShell in Windows).Then navigate to the directory where you store Rust packages
for this blog, and run the following command:
cargo new structs
Then change into the newly created structs
directory, and open that directory in VS Code or your favorite IDE.
Note: Using VS Code will make it easier to follow along with this blog series. Installing the Rust Analyzer extension is also highly recommended.
Also, opening the directory containing your Rust package
allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the structs directory in VS Code.
Open the file src/main.rs and replace its contents entirely with this code:
#[derive(Debug)]
struct Customer {
first_name: String,
last_name: String,
email: String,
phone: String,
address_1: String,
address_2: String,
city: String,
state: String,
zip_code: String,
}
fn main() {
let customer_one = Customer {
first_name: String::from("Frank"),
last_name: String::from("Smith"),
email: String::from("frank@xyz.com"),
phone: String::from("(123) 456-7890"),
address_1: String::from("376 Main Street"),
address_2: String::from("Apt 302"),
city: String::from("West Springfield"),
state: String::from("MA"),
zip_code: String::from("01089"),
};
println!("{}", customer_one);
}
Note that struct definitions must be outside all functions. They can be above or below the main() function, but not inside it.
First we define the Customer struct, with the fields we saw before. We define the struct above function main(), but you could define it below main() as well. If the struct definition is in the same file as main() either location is fine.
Then in main() we define an instance of the Customer struct. Defining the struct and its fields is like a blueprint. It doesn’t have data, it just describes what data a Customer data item will have.
To create an instance of the Customer struct we use the let keyword, followed by the name of the instance, in this case customer_one.
After the assignment operator ( = ), we have the struct name, Customer, and then inside curly braces we specify the struct field names one by one, a colon after the field name, and then the field value. For example:
first_name: String::from("Frank")
Note that when creating a struct instance you must provide data for all the struct fields. None of the fields are optional. There are techniques for providing default values for struct fields, but that will be covered in a future post.
We add the line deriving Debug so we can print Customer, which is a custom data type. More on this later.
#[derive(Debug)]
Printing our Customer struct Instance
After defining this Customer struct instance, we output it to the shell window with the println!() macro. But if you execute cargo run
at this point, you’ll get a compile error.
You can also see this error in VS Code if you have the Rust Analyzer extension installed, by hovering over the red squiggly line under customer_one.
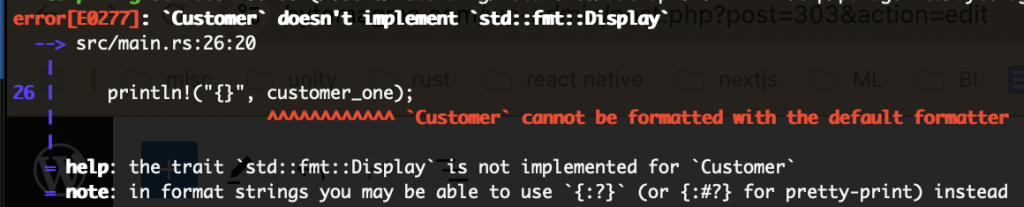
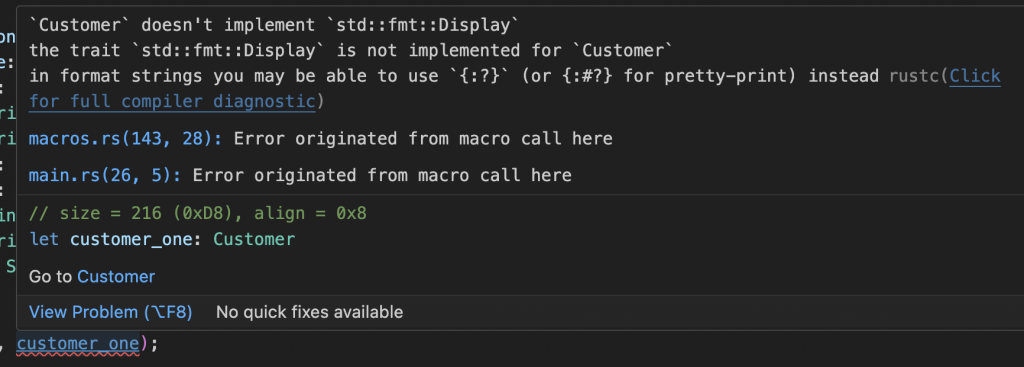
Up to now, when we wanted to print a value with println!() we did something like this:
let x = 5;
println!("{x}");
or this
let x = 5;
println!("{}", x);
These are equivalent. You can place the variable x directly inside the placeholder {} or after it, separated with a comma.
But the placeholder {} can only be used with data types that implement the Display trait.
We’ll discuss traits more deeply in a future post, but in short, a trait is like a shared set of behaviors or capabilities that types can implement.
The Display trait provides a user-friendly representation of a value. The Display trait is automatically implemented for the primitive data types: the integer types, float types, bool, char, the string types String and &str.
The Display trait is also implemented for some other types that are part of the Rust standard library, a library included with Rust to provide additional functionality out of the box.
But complex types such as Vec, HashMap, HashSet, etc. do not implement the Display trait, so we can’t use the {} placeholder with them.
Our Customer type is a custom struct and, by default, doesn’t implement the Display trait, so we can’t use the {} placeholder with it.
Rust provides two other placeholders that can be used with complex and custom types: {:?} and {:#?}
The {:?} placeholder outputs data like this:
Customer { first_name: "Alice", last_name: "Anderson", email: "alice@example.com", phone: "555-1234" }
The {:#?} (pretty print) placeholder outputs data like this:
Customer {
first_name: "Alice",
last_name: "Anderson",
email: "alice@example.com",
phone: "555-1234",
}
Usually for complex types and custom types we use the {:?} placeholder, but that requires the type to implement the Debug trait, and our Customer struct does not implement the Debug trait.
At this point, you might be thinking: “Wait, what? I just want to print this thing!”
Rather than manually implementing the Display
or Debug
traits (which you could do, but that’s beyond the scope of this post), we can derive default functionality from the Debug trait like this:
#[derive(Debug)]
struct Customer {
first_name: String,
last_name: String,
email: String,
phone: String,
address_1: String,
address_2: String,
city: String,
state: String,
zip_code: String,
}
Rust uses the word “derive” because it’s automatically generating (deriving) the code you would otherwise have to write by hand.
Now we can use the {:?} placeholder, execute cargo run, and our Customer instance is output to the shell window:
println!("{:?}", customer_one);
Customer { first_name: "Frank", last_name: "Smith", email: "frank@xyz.com", phone: "(123) 456-7890", address_1: "376 Main Street", address_2: "Apt 302", city: "West Springfield", state: "MA", zip_code: "01089" }
Now that we’ve got our Customer instance printing properly, let’s explore how to access the data inside its fields.
Accessing Fields
Accessing the fields of a struct instance is easy using dot notation. For example:
println!("{}", customer_one.first_name); // Frank
You might want to combine fields like first_name and last_name to construct a full name:
let full_name = customer_one.first_name + &customer_one.last_name;
However, if you add that line before this one:
println!("{:?}", customer_one);
you’ll get a partial move error.
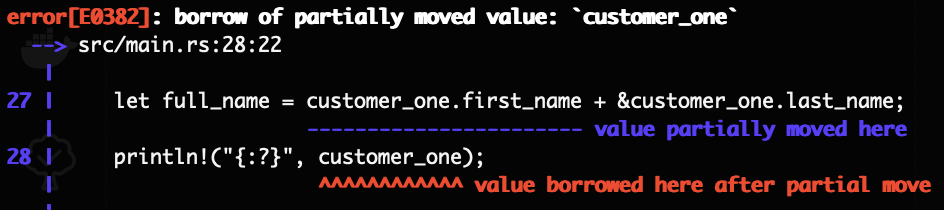
Why? Because in Rust, String
is not a “copy” type — it gets moved when used by value. The +
operator takes ownership of the left-hand String
, so customer_one.first_name
is moved into full_name
.
Since only one field (first_name
) is moved, the rest of customer_one
is still intact — but Rust won’t let you use the partially invalid customer_one
afterward. This is called a partial move, and it makes customer_one
unusable until the moved field is replaced.
In contrast, &customer_one.last_name
is a reference — a borrow, not a move—so that doesn’t cause any issues.
🛠 Fixing the error
One common way to fix this is to clone the field:
let full_name = customer_one.first_name.clone() + &customer_one.last_name;
This creates a copy of the string data on the heap and avoids moving the original first_name
.
You can enable full cloning capabilities, for example cloning entire Customer instances, by also deriving Clone for the struct.
#[derive(Debug, Clone)]
struct Customer {
first_name: String,
last_name: String,
email: String,
phone: String,
address_1: String,
address_2: String,
city: String,
state: String,
zip_code: String,
}
Ownership move errors like this are fairly common in Rust, especially when working with String
, but over time, you’ll become comfortable handling them.
👉 For a deeper dive, check out our post on:
Ownership, Moving, and Borrowing in Rust
Let’s see how we can update data in a struct, as well as creating new struct instances from existing instances.
Updating Structs
Next, lets consider how to update the data in a struct instance.
We’ll use a more simple struct with fewer fields to keep our code more concise. Delete our current code in main.rs (or use a multi-line comment /* CODE TO COMMENT OUT */ to keep it).
Now add this code to main.rs:
#[derive(Debug)]
struct Student {
active: bool,
name: String,
email: String,
age: u8,
}
fn main() {
let mut student_one = Student {
active: true,
name: String::from("Susan Carson"),
email: String::from("susan_carson@xyzcompany.com"),
age: 24,
};
println!("{:?}", student_one);
}
Note that struct definitions must be outside the main() function. They can be above or below the main() function, but not inside it.
We’ve defined a Student struct with four fields. We create an instance of the struct and we print it out. Note that we used the mut keyword to ensure that our struct instance can be changed.
If we discovered that we made a mistake entering Susan’s age, and that she is in fact 23 years old, we could use dot notation to change her age in our struct instance:
student_one.age = 23;
println!("{:?}", student_one);
Running the code with cargo run we see Susan’s age before and after the correction:
Student { active: true, name: "Susan Carson", email: "susan_carson@xyzcompany.com", age: 24 }
Student { active: true, name: "Susan Carson", email: "susan_carson@xyzcompany.com", age: 23 }
Note that the entire struct instance must be mutable. We cannot make individual struct fields mutable and leave other fields immutable.
Field Init Shorthand Syntax
Add this function below main() that creates a Student instance:
fn build_student(active: bool, name: String, email: String, age: u8) -> Student {
Student {
active: active,
name: name,
email: email,
age: age,
}
}
Now at the bottom of main() create a new Student instance.
let student_two = build_student(true, String::from("Tim Weston"), String::from("tweston@greatmail.com"), 32);
Note that in function build_student() because the expression creating the new Student instance does not end with a semicolon, the instance is implicitly returned from the function, as if we had explicitly returned the instance:
return Student {
active: active,
name: name,
email: email,
age: age,
};
Because the function parameter names are the same as the Student struct field names, we can use the field init shorthand syntax when creating the instance:
fn build_student(active: bool, name: String, email: String, age: u8) -> Student {
Student {
active,
name,
email,
age,
}
}
Creating Instances from Other Instances
Sometimes it can be useful to create a new struct instance that includes some fields of an another instance, but has new values for other fields.
let student_three = Student {
active: student_two.active,
name: String::from("Ronald Jones"),
email: String::from("ronald@small_company.com"),
age: 45,
};
We can use the more concise struct update syntax.
let student_three = Student {
name: String::from("Ronald Jones"),
email: String::from("ronald@small_company.com"),
age: 45,
..student_two
};
At the end of the new Student struct body we have the struct update syntax:
..student_two
This copies or moves all remaining fields from student_two
that are not explicitly set in the new instance.
Because fields name, email and age have already been set, only the active field will be copied.
This can cause problems if the original instance is used after the move. Rust will throw a compile-time error if you try to access fields that were moved.
If you use the struct update syntax to bring over all fields from student_two:
let student_three = Student {
..student_two
};
Because the name and email fields are Strings, which are not Copy
types, ownership is moved when passed by value.those fields will now be invalid in student_two.
// This will cause a compile error: value borrowed here after move
// println!("student_two's name: {}", student_two.name);
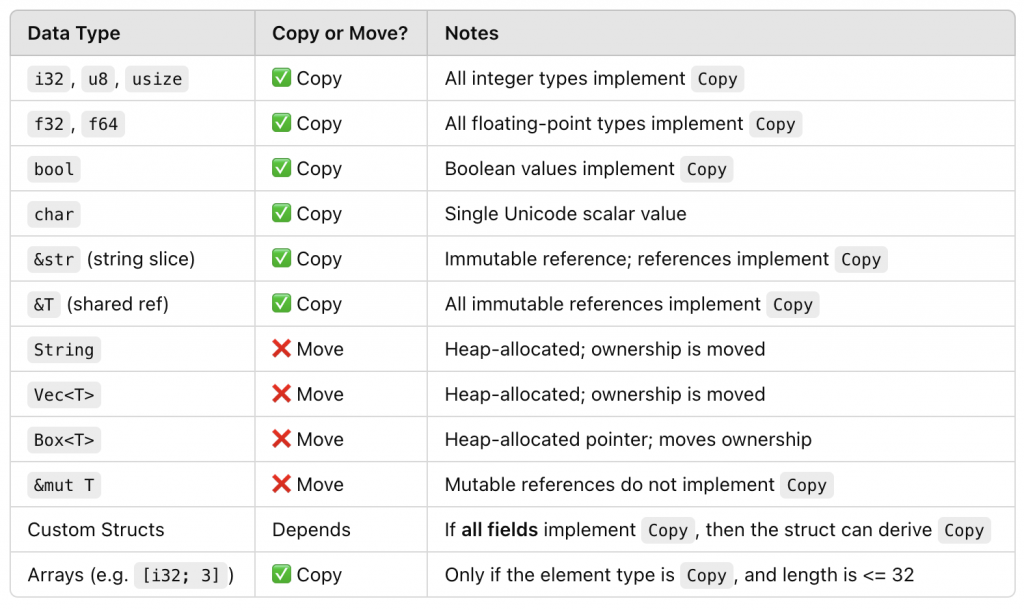
🔑 Tip: Most primitive types are Copy, and most heap-allocated types (like String, Vec, and Box) are Move. If in doubt, try assigning or passing the value — Rust will tell you at compile time whether it was moved or copied.
So understand the implications of using the struct update syntax.
Destructuring Struct Fields
Using the following syntax you can destructure a struct, using its field values to create new variables.
let Student {active, name, email, age} = (student_one);
println!("{active}, {name}, {email}, {age}");
This creates four new variables, active, name, email and age, whose values are populated from the student_one Student instance.
Note that move fields like name and email (Strings) in the student_one instance will be invalid after this destructuring.
You can even create variables with different names:
let Student {active: student_is_active, name: student_name, email: student_email, age: student_age} = (student_one);
println!("{student_is_active}, {student_name}, {student_email}, {student_age}");
We destructure data from the student_one instance, but we place the values in new variables student_is_active, student_name, student_email, and student_age.
Tuple Structs and Unit-Like Structs
Now we’ll look at two interesting uses of structs, tuple structs and unit-like structs.
Tuple Structs
Tuple structs resemble regular tuples, but they have a distinct type name, giving them semantic meaning. Unlike regular structs, their fields are not named — they are accessed by position.
To define a tuple struct, we have the struct keyword, the name of the struct, and the field types in parentheses.
Comment out or replace the code in main.rs with the following:
fn main() {
let red = Color(255, 0, 0);
let green = Color(0, 255, 0);
let blue = Color(0, 0, 255);
println!("{}, {}, {}", red.0, red.1, red.2);
// Output: 255, 0, 0
let origin = Coords(0, 0, 0);
let dest = Coords(100, 200, 50);
println!("{}, {}, {}", dest.0, dest.1, dest.2);
// Output: 100, 200, 50
}
struct Color(u8, u8, u8); // Represents RGB values
struct Coords(u8, u8, u8); // Represents 3D coordinates
Note that struct definitions must be outside the main() function. They can be above or below the main() function, but not inside it.
We define a tuple struct with the name Color that has three u8 fields. In main() we create three instances, with the field values specifying RGB values for red, green and blue.
As the struct fields are unnamed, we access them by position index, with indexes beginning with zero.
println!("{}, {}, {}", red.0, red.1, red.2);
// Output: 255, 0, 0
We also create a Coords tuple struct whose fields have the same types. Even though the types of the Color and Coords tuple structs are the same, with the same number of fields, they are distinct and separate tuple structs.
This ensures type safety—Rust won’t let you assign a Color to a variable expecting a Coords, even though their internal structure is the same.
Similar to tuples, you can destructure tuple structs, but you need to specify the name of the tuple struct:
let Color(r, g, b) = red;
println!("{}, {}, {}", r, g, b);
// Output: 255, 0, 0
Tuple structs are useful when a tuple needs a meaningful type name, but naming each field would be unnecessary or overly verbose.
Unit-Like Structs with no Fields!
Unit-like structs are structs with a name but no fields. They are a bit abstract but have many uses. They behave similar to the unit type ().
To define a unit-like struct, you have the struct keyword and the struct name, with no curly braces or parentheses.
In the following example, we define three unit-like structs representing the current login state for a user.
struct LoggedOut;
struct LoggingIn;
struct LoggedIn;
As we build out our system, we can check a user’s login state to see which of these states it matches. So in this use case, unit-like structs are being used like state flags.
Now it’s time to see how we can add behavior to structs with methods and associated functions.
Adding Behavior with impl
You can define methods and associated functions for a struct. The key difference between them is that methods are relevant within the context of a struct instance, and associated functions do not need a struct instance. This will become more clear as we look at methods and associated functions.
Note that struct methods and associated functions are named in snake_case.
To get started, comment out or delete the code in main.rs and replace it with this struct definition:
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
fn main() {}
This is a simple struct representing a rectangle shape. It has two fields, width and height, representing the dimensions of the rectangle.
Struct Methods
Struct methods can be called on a struct instance.
We define struct methods in an impl (implementation) block:
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// Method that takes &self
fn area(&self) -> u32 {
self.width * self.height
}
// Method that compares with another Rectangle
fn can_hold(&self, other: &Self) -> bool {
self.width >= other.width && self.height >= other.height
}
}
fn main() {}
Add this implementation block in main.rs.
The implementation block starts with the impl keyword, then the name of the struct for which we will implement methods (Rectangle), then curly braces for the implementation body.
Here we’ve defined two methods for the Rectangle struct, area() and can_hold().
The first argument of a struct method is always self, a variable that refers to the instance on which the method is being called.
Note that this first argument could be any of these three, depending on whether or not you need the method to take ownership of the instance, or if it should be borrowed, immutably or mutably.
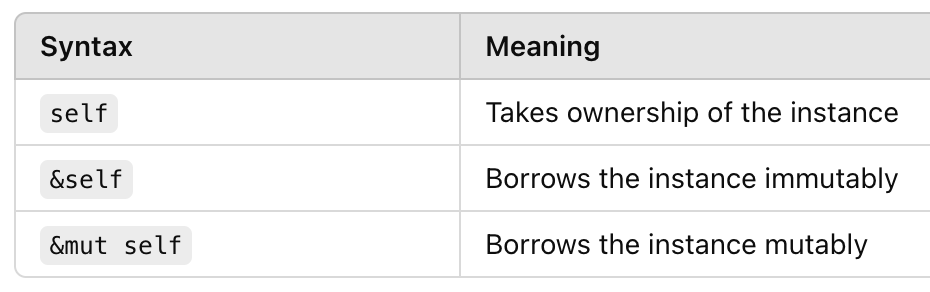
When calling a struct method, this first parameter self will be passed in automatically by Rust, you do not need to pass it explicitly.
The area(&self) method takes a reference to self because we want to borrow the instance immutably. The function doesn’t change anything, it just uses the width and height fields’ data.
fn area(&self) -> u32 {
self.width * self.height
}
Note how we refer to the instance’s width and height fields using dot notation and the self parameter. Also notice that this method returns a value, the calculated area of the rectangle instance.
Add this code to main():
let rect1 = Rectangle {width: 30, height: 50};
println!("Area of rect1: {} square pixels", rect1.area());
// Output: Area of rect1: 1500 square pixels
Notice how we call the area() method on instance rect1.
rect1.area()
The can_hold(&self, other: &Self) takes a reference to self as the first parameter, but it also takes a reference to another Rectangle struct instance.
// Method that compares with another Rectangle
fn can_hold(&self, other: &Self) -> bool {
self.width >= other.width && self.height >= other.height
}
This method returns true if the “other” rectangle fits inside the “self” rectangle. If it doesn’t fit the method returns false. So this method returns true if self
can completely contain other
.
So if our method will use custom parameters, such as other in this case, they come after the default self parameter.
Note the type of the other parameter is &Self (capital S). This is an idiomatic way of saying that the type of the other parameter is &Rectangle. You could change the method definition to explicitly use the &Rectangle type, but &Self is more idiomatic.
fn can_hold(&self, other: &Rectangle) -> bool {
self.width >= other.width && self.height >= other.height
}
The other parameter is a reference to the Rectangle instance being compared because we don’t want this method taking ownership. If the other parameter were Rectangle (no &), then the instance being compared would become invalid and unusable.
Add this code to main() to create two more instances of the Rectangle struct and to call the can_hold() method to compare instances.
let rect2 = Rectangle {width: 10, height: 40};
let rect3 = Rectangle {width: 60, height: 45};
println!("rect1 can hold rect2: {}", rect1.can_hold(&rect2));
println!("rect1 can hold rect3: {}", rect1.can_hold(&rect3));
// Output: rect1 can hold rect2: true
// Output: rect1 can hold rect3: false
Examine the length and height of each rectangle.
let rect1 = Rectangle {width: 30, height: 50};
let rect2 = Rectangle {width: 10, height: 40};
let rect3 = Rectangle {width: 60, height: 45};
It’s clear that rect2 would fit inside rect1, but that rect3 would not fit inside rect1.
Notice how we call the can_hold() method on the rect1 instance, passing a reference to the rect2 and rect3 instances:
rect1.can_hold(&rect2));
rect1.can_hold(&rect3));
This is what distinguishes struct methods from struct associated methods, which we will discuss next.
Struct Associated Functions
Struct methods are called on an instance of the struct:
rect1.area()
Struct associated functions are called on the struct type itself:
Rectangle::new(30, 50)
Methods operate on an instance (self
) and are called with dot notation.
Associated functions don’t take self
and are called with ::
, like Rectangle::new()
.
One common use of associated functions is to define a “constructor-like” associated function. Add this code to the Rectangle struct impl block:
// Associated function - constructor
fn new(width: u32, height: u32) -> Self {
Self { width, height }
}
This associated function does not take the self parameter. It takes width and height parameters and returns a new Rectangle instance, setting the instance width and height field values to the provided parameters.
The function return type is Self (capital S), which is an idiomatic way of specifying Rectangle. You could change the function like this and it would be equivalent:
fn new(width: u32, height: u32) -> Rectangle {
Rectangle { width, height }
}
But using Self is considered more idiomatic.
Now that we have a constructor associated function we can replace these lines in main:
let rect1 = Rectangle {width: 30, height: 50};
let rect2 = Rectangle {width: 10, height: 40};
let rect3 = Rectangle {width: 60, height: 45};
with these lines:
let rect1 = Rectangle::new(30, 50);
let rect2 = Rectangle::new(10, 40);
let rect3 = Rectangle::new(60, 45);
We call the associated function on the type, Rectangle, passing it width and height values, and it returns an instance of Rectangle.
Now add this code for an associated function that takes a single parameter and returns an instance of Rectangle. Add it to the Rectangle impl block.
// New associated function - creates a square
fn square(size: u32) -> Self {
Self {
width: size,
height: size,
}
}
A square is simply a rectangle whose width and height are the same, so this is just a convenience function to create a square.
Multiple impl Blocks
The impl block is separate from the struct definition. We can have multiple impl blocks, and define methods and associated functions in them.
This can be good for code organization. You might put all struct methods for a particular struct in one impl block, and its associated functions in another impl block. Or you might use multiple impl blocks to organize methods and associated functions with related functionality.
Wrapping up…
That wraps up our discussion of Rust structs. We’ll learn more about structs and see more examples of real-world use cases for them in future posts.
Thank you so much for stopping by, and including ByteMagma in your Rust programming mastery journey!
Leave a Reply