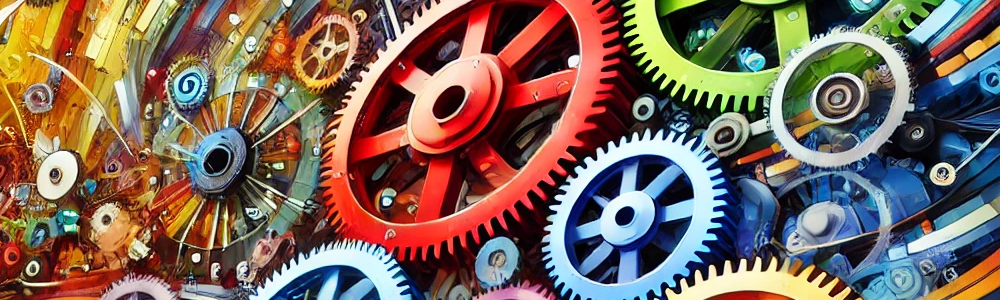
Functions are code blocks designed to perform a task. Functions help with code reuse, readability, and organization. In this post we’ll learn about functions in Rust.
If you’re just getting started with Rust, these posts earlier in this series on Rust programming may be helpful:
Setting Up Your Rust Development Environment
Mutability, Copying, and Why Some Things Just Vanish
Introduction
Functions play a central role in any programming language that supports them. Functions allow you to write a block of code, give it an identifier (a name), optionally pass data to that code block, and optionally return data from that code block.
With functions, we can create code blocks that can be used throughout our Rust packages
. Think of an operating system written in Rust that needs to make various file manipulation functionality available throughout the system.
Various components of the operating system may need to create and edit files, delete files, and modify the contents of files. If you needed to write code for these actions in multiple places in the system, it would waste developer time and could lead to inconsistencies.
So functions help with code reuse, readability, and organization.
Functions in Rust – The Basics
To get started, let’s generate a new Rust package
to begin our discussion of Rust functions. Open a new shell window (Terminal on Mac/Linux, Cmd or Power shell on Windows). Now CD to the folder you’re using for Rust packages
as you follow this blog and execute this command:
cargo new functions
Now CD into the functions directory and open that directory in VS Code or your favorite IDE.
Note: using VS Code will make it easier to follow along with our posts. And installing the Rust Analyzer extension is also beneficial.
Also, opening the directory containing your Rust package
allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the functions directory in VS Code.
Note that if you get this Rust Analyzer extension error, you can probably ignore it:
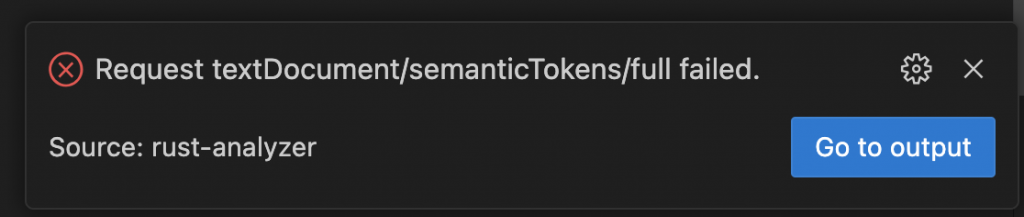
Open the file src/main.rs. The main() function is the entry point to your Rust package
. It gets executed when you run your package
.
Most Rust packages
you create as you go through our posts must have a main.rs file and a main() function. When this is not the case we’ll let you know.
Now execute this command in the shell window:
cargo run
You should be no errors and the text Hello, world! should be output to the shell window.
Let’s analyze the default main() function created when you generated this package
.
fn main() {
println!("Hello, world!");
}
You start Rust functions with the fn keyword. Then you have an identifier (a name), that we use to identify this function, in this case that identifier is main.
By convention, function names in Rust are in snake case, which means all lowercase letters with words separated by an underscore ( _ ).
Examples of Rust function identifiers (names):
- calculate_tax
- uppercase_first_letter
- clear_buffer
Please name your functions with identifiers that clearly indicate what the function does. It doesn’t need to be super long, but other developers (and you six months from now), will appreciate a function name that gives a good indication of what the function does.
After the identifier comes opening and closing parentheses (), and then opening and closing curly braces { }.
Later in this post we’ll learn how to pass data into the function that the function can use to do its work, and how to return data from a function after it has done its work. For now we have a simple function taking no data and returning no data.
The fn keyword, the identifier, the parenthesis (and parameters if the function takes any), and the return type (if the function returns data) is known as the function signature:
fn main()
The curly braces form a code block, and together with the statements and expressions inside the curly braces, they make up the body of the function. The work done by the function happens in the body.
{
println!("Hello, world!");
}
The body of our main() function contains a single statement, where we print the text Hello, world! to the shell window. We do this with println!(), a Rust macro that prints to the shell window.
We’ll cover macros in detail in a future post, and we’ll see other Rust macro used in future posts as well.
For now understand that In Rust, when you use a macro, the compiler expands it into code during an early phase of compilation. This generated code becomes part of your program, even though you don’t see it directly.
Calling Functions
Functions do nothing on their own. Functions need to be called. The main() function in main.rs is called automatically as the entry point to your Rust package
.
Let’s define our own function and call it from main. Go ahead and replace the entire contents of the main.rs file with this code.
fn main() {
greeting();
}
fn greeting() {
println!("Hello from the greeting function!");
}
Save the file and execute cargo run in the shell window. You should see the text Hello from the greeting function! output to the shell window.
If you get errors or you don’t see that text output, please verify the code in your main.rs file is identical to the above code, and that you have saved the changes.
Here we see the power of functions. We defined a function, added a statement in the body that does something, and we called our function within the main() function code block.
Notice when we call our function we have the function identifier greeting, followed by opening and closing parentheses, and then a semicolon to complete the statement.
The semicolon is optional, and in that case we have an expression, not a statement. See the last section of this post for a discussion of the difference between an expression and a statement.
The code flow proceeds like this:
- enter the main() function when the
package
is run - execute the greeting function call
- enter the greeting() function
- execute the println! macro
- return from the greeting() function
- return from the main() function
- the
package
exits
Notice that we return from the greeting and main functions. Because we did not explicitly return a value, our functions implicitly return the unit value ().
The Rust unit type () is a scalar type that represents the absence of a meaningful value. It has exactly one value: (). We’re not explicitly returning anything so by default the unit value is returned.
The unit type was described in our post on Rust Variables and Data Types.
Passing Parameters to Functions
Functions can optionally take inputs, officially called parameters. Parameters are data you pass into the function, and the function can use that data to do its work.
Let’s modify our greeting() function to take two parameters, name and age. Replace the entire code in main.rs with the following code:
fn main() {
greeting("Steve", 32);
}
fn greeting(name: &str, age: u8) {
println!("Hello {}, you are {} years old!", name, age);
}
Note: if you want to keep the current code for your reference, you can enclose it in a multi-line comment like this:
/*
fn main() {
greeting();
}
fn greeting() {
println!("Hello from the greeting function!");
}
*/
Save the file, execute cargo run and you should see this output:
Hello Steve, you are 32 years old!
Let’s examine the new function signature:
fn greeting(name: &str, age: u8)
Inside the parentheses we’ve added two parameters, name and age. We’ve also specified the data types of the parameters. name is a string slice &str, and age is a u8, an unsigned integer.
Function parameters are separated by a comma.
Specifying the name and data type of function parameters is required in Rust. Note that this is not true of closures, but we won’t be covering closures until a post some time in the future.
&str is a string slice. A string slice is a reference to a sequence of UTF-8 encoded characters in memory.
That’s the official definition. For now just understand that &str is something that points to the text Steve in memory. We’ll discuss string slices and their close cousin, Strings, in a post in the near future.
We chose u8 as the data type for age because a u8 can hold values between 0 and 255, and humans typically don’t live to be older than 255 years old.
Choosing u8 as a data type is a good practice in Rust. You should think about the data you’ll be working with and choose an appropriate data type. This helps with efficient memory management.
In general functions can take as many parameters as you feel they need. Having said that, if you find your functions taking very large numbers of parameters, you might need to rethink your design, and create one or more structs or enums to represent data. structs and enums will be discussed in an upcoming post.
Look at how the greeting() function makes use of the two parameters inside the code block:
println!("Hello {}, you are {} years old!", name, age);
Here we are using {} placeholders in the call to the println!() macro. We do this so the data values in the name and age parameters can be inserted into our message string.
We’ll see more examples and variations of placeholders and additional macros that use them in future posts.
You’ll use these placeholders and their variations often when you print to the shell or for other purposes.
Parameters and Arguments
A note on terminology. Strictly speaking, when we define function signatures we are specifying parameters the function takes. When we actually call functions, we put data values in the parentheses, and these are called arguments.
But the terms parameters and arguments are used loosely by many people to refer to both, so don’t worry about this.
Returning Data from Functions
Functions are great for doing work for us, but often you want the function to return data back to the calling code block. Let’s modify our code again to see returning data in action.
Replace the code in main.rs with the following code, or optionally comment out the current code as described above:
fn main() {
let message = greeting("Frank", 28);
println!("{message}");
}
fn greeting(name: &str, age: u8) -> String {
let msg = format!("Yo {}, you are {} years old!", name, age);
return msg;
}
First let’s examine the greeting() function signature:
fn greeting(name: &str, age: u8) -> String
We’ve added a return type specification:
-> String
This means our function will return a String. We use a hyphen (dash) and the greater than symbol, and then the data type of the value that will be returned, in this case String.
We’ll discuss Strings, and their cousins string slices &str in a post coming soon. For now just understand that a String also represents some text.
If you plan to return data from a function you must add this return type specifier to the function signature. And you must return a value of that type.
Go ahead and save main.rs and execute cargo run. You should see this output in the shell window:
Yo Frank, you are 28 years old!
I’ve changed the message a bit so you see our code has actually changed.
Just a quick note on what’s going on in our greeting() function now. We define a variable msg, and we set the value of that variable to what is returned by the format!() macro.
println!() prints formatted text to the shell window, and format!() returns the formatted text as a String, which you can use later in your code. So msg will contain our message, with the placeholders replaced with name and age.
let msg = format!("Yo {}, you are {} years old!", name, age);
return msg;
Finally, we return the msg variable value using the return keyword. The format!() macro returns a formatted String, so the compiler is satisfied.
Back in the main() function, we’ve assigned the return value of greeting() to a variable message and we’re printing message to the shell. We also see another way to insert variable values into placeholders.
Let’s look at what happens when we don’t return the value we’ve specified in our function signature.
Comment out the line returning the data with a single line comment. A single line comment starts with two forward slashes // and the compiler will ignore everything after the //.
//return msg;
Save the file and execute cargo run again, and you get an error message. If you have the Rust Analyzer VS Code extension installed you can also see a red squiggly line which on hover reveals the error.


Because we’ve added the return type specifier of -> String to our function signature, the compiler expects a String to be returned from the function, but we commented out that line.
Earlier we learned that Rust functions return the unit type () by default when we do not return a value in our function. But we’ve said we return a String, so the compiler complains.
Now uncomment that line by removing the // from the start of the line. Also remove the return keyword and the ending semicolon, so your greeting() function looks like this:
fn greeting(name: &str, age: u8) -> String {
let msg = format!("Yo {}, you are {} years old!", name, age);
msg
}
Implicit Returns from Functions
This is a very super duper important concept in Rust. In a function, the last expression is implicitly returned — but only if no earlier return has already happened and the expression is not terminated with a semicolon.
That might sound like a lot, but here’s the key takeaway: in Rust, it’s very common to see (and write!) functions where the last line has no semicolon. That’s because it’s an expression, and whatever value it evaluates to will be returned automatically.
Note that you will also get a compiler error if you return a type that differs from the type specified in the function signature. So for the greeting() function, if we return a u16, or a bool, or a char, or a string slice &str instead of returning a String, we’ll get a compiler error.
Sometimes return types can use coercion but often you must specify concrete types.
Expressions and Statements
Moving forward, it’s important to understand the difference between an expression and a statement.
In Rust, expressions produce a value, while statements do something but don’t return a value.
Expression
Evaluates to a value, and can be used as part of other expressions. An expression does not end in a semicolon.
Examples:
5 // evaluates to 5
x + 1 // evaluates to x + 1
some_func() // evaluates to the function's return value
if x > 0 { 1 } else { -1 } // expression that returns a value
Some of these expressions might not be clear, but just understand that an expression does not end with a semicolon, and it evaluates to some value.
Statement
Performs an action, like declaring a variable or calling a macro. Does not evaluate to a value. Ends in a semicolon ( ; ).
Examples:
let x = 5; // statement (declares a variable)
println!("Hi"); // statement (side effect, no value returned)
So a statement ends with a semicolon and performs an action, such as assigning the value 5 to variable x, or printing the value Hi to the shell window.
This is important because if you want to return a value from a function with a statement (ends with a semicolon), you need the return keyword and a semicolon at the end.
If you want to implicitly return a value from a function with an expression, have a valid expression as the last line of the function body and do not end the expression with a semicolon.
Wrapping up…
So we’ve seen how to define functions, how to specify parameters, how to return values from functions and how to make use of those returned values.
We also touched upon several related topics.
Functions make your code cleaner and modular. Explicit types in parameters and return values make behavior predictable. Functions are a key concept in Rust and other programming languages.
We’ll see much, much, much, so much more about functions as we move forward in your quest for Rust mastery.
Thank you so much for allowing ByteMagma to be a part of your journey. Come back soon for more posts!
Leave a Reply