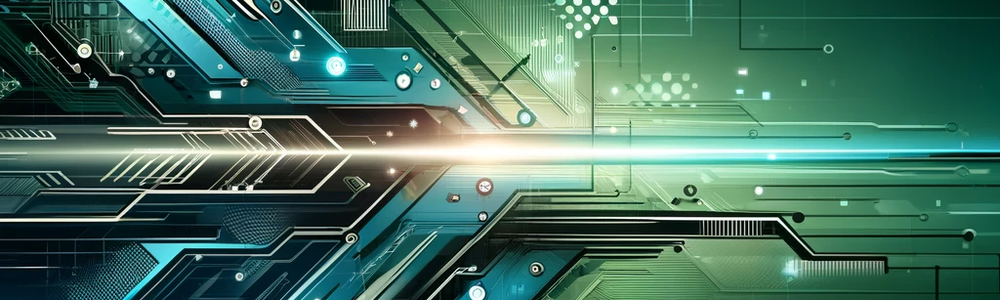
Our first two posts described what Rust is and how to set up the development environment. In this post we start learning Rust.
If you need to set up your development environment you can refer to this post:
Setting Up Your Rust Development Environment
Introduction
Pretty much anytime you start learning a new programming language, you start with variables, as they are so basic to programming.
I assume most readers know what a variable is, but just in case, a variable is a place to store a value. The value might be a number, such as your age, or the price of a cup of coffee.
Or the value might be a word, such as your name, or your favorite fruit. The value might also be what is known as a boolean, with values true or false. This will become clear as we examine Rust variables.
Defining Variables in Rust
Here is an example of declaring a Rust variable:
let my_age: u8;
In Rust, you start a variable declaration with the “let” keyword. Next comes the variable name (identifier), in this case my_age. The naming convention for Rust variables is lowercase snake case. That means all lowercase letters, with words separated by underscores.
Following the variable name we have a colon, and then the data type of the variable. We’ll discuss Rust data types later in this post, but when you specify the data type of a variable, you’re telling the Rust compiler the type of data that will be stored in the variable.
Finally, the variable declaration is terminated with a semicolon. The semicolon is required when declaring variables.
We’ve specified that variable my_age will store an unsigned integer of 8 bits (one byte). This means our variable can store the numbers 0 – 255. The “u” in u8 means this variable can only store “unsigned” integers, so no negative numbers.
Also, you cannot store a floating point number in a u8, such as 2.5. So a u8 can store the numbers 0 – 255 with no numbers having decimal points.
In the example above we have declared the variable but we have not assigned a value to it. It stores no data yet.
You can, and often will, assign a value to a variable when you declare it:
let my_age: u8 = 18;
So now we have a variable whose name is my_age that stores the value 18, a u8 value.
Let’s see some more Rust variable definitions.
let my_age: u8 = 10;
let price = 5.99;
let choice = 'Y';
let rust_is_good: bool = true;
Take a close look at these variable definitions. Do you have some questions?
The definition of the price variable did not specify the data type! This is because the Rust compiler is able to infer (guess) the data type based on the data being stored in the variable. The same goes for the choice variable, which stores a single character ‘Y’.
We could have defined price and choice like this, explicitly specifying the data type:
let price: f32 = 5.99;
let choice: char = 'Y';
As you learn more about Rust and the many things you can do with it, you’ll see many situations where you must specify the data type, or you’ll get a error from the compiler. But often you can omit the data type and let the Rust compiler infer the type.
It’s important as you’re learning Rust to actually write some code, so let’s create a new Rust package
you can use to learn more about variables, and so you can see examples of compiler errors.
I encourage you to create a directory somewhere named byte_magma_packages where you can put all your packages
created while following out Byte Magma posts. But you can put these packages
anywhere.
Open a shell window (Terminal on Mac/Linux, Cmd or Powershell on Windows) and CD to the byte_magma_packages directory or whatever directory you will use to hold Rust packages
.
Now type this command:
cargo new variables_data_types

cargo is a tool that was installed with Rust. It is the package manager and build system for Rust. It creates new packages
, build and runs them, manages dependencies, runs tests, builds documentation, and more.
Now CD into the variables_data_types directory that was created when you executed cargo new. Also, in VS Code (or whatever IDE you use), open that directory.
Open the file src/main.rs. It should contain this default Rust package
code:
fn main() {
println!("Hello, world!");
}
Note that if you get this Rust Analyzer extension error, you can probably ignore it:
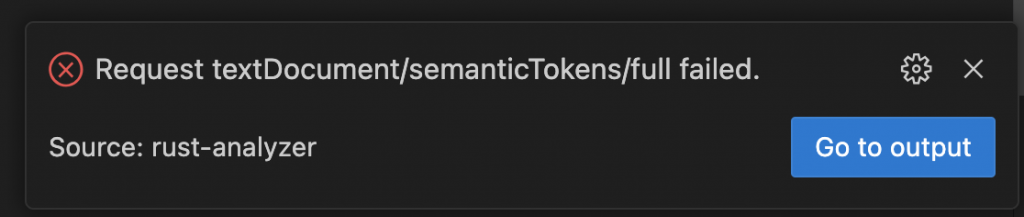
I hope you are using VS Code as your IDE (Integrated Development Environment), and I hope you have installed the Rust Analyzer extension for VS Code, as it will be easier to follow along in our blog posts. If you don’t have it you can refer to our post on setting up the Rust Development Environment.
You can run our new Rust package
by executing this inside the variables_data_types directory:
cargo run

You should see Hello, world! printed to the screen.
Rust Data Types
It’s time to discuss Rust data types. To get started, replace the contents of file main.rs in the variables_data_types package
we just created with the following code. main.rs is the main code file for the package
, and is located in the src directory.
Note that some lines below wrap but in VS Code in main.rs it is best if they do not wrap for clarity.
fn main() {
// booleans
let active: bool = true;
let expired: bool = false;
// character
let choice: char = 'Y';
// signed integers
let signed_small_number: i8 = 125;
let signed_medium_number: i16 = -12_300;
let signed_larger_number: i32 = 1_147_483_647;
let signed_even_larger_number: i64 = 3_223_372_036_854_775_807;
let signed_very_large_number: i128 = 100141183460469231731687303715884105727;
let signed_pointer_size: isize = -500_000_000_000;
// unsigned integers
let unsigned_small_number: u8 = 200;
let unsigned_medium_number: u16 = 45_339;
let unsigned_larger_number: u32 = 3_294_967_295;
let unsigned_even_larger_number: u64 = 12_446_744_073_709_551_615;
let unsigned_very_large_number: u128 = 300282366920938463463374607431768211455;
let unsigned_pointer_size: usize = 15_446_744_073_709_551_615;
// floating point numbers
let float_number: f32 = 3.1415926;
let higher_precision_float_number: f64 = 3.141592653589793;
// unit type
let initialized: () = ();
// string slice type
let name: &str = "Bob";
// compound types
let name_age_tuple: (&str, u8) = ("Bob", 34);
let scores: [u8; 20] = [0; 20];
}
One thing to notice is that you can use an underscore ( _ ) to make larger numbers easier to read. It’s kind of like the comma you might use in a number like 250,220,883. You can place the underscores anywhere you want, but convention has them after every 3rd digit.
After placing this code in main.rs, execute cargo run in the shell window. You will get a lot of warnings about these variables not being used, which you can ignore. But you should get no errors.
Now lets discuss these variables and their data types. In this post we’ll discuss the primitive data types. Within the primitive data types there are scalar types and compound types, and a few special types.
Scalar types are single values like numbers, booleans, and characters. We’ll cover these one group at a time.
Integer Types
Integer types are scalars that are numbers that do not have a fractional part, so no decimal point. Examples include:
25
376
2,601
346,204,550
Rust has twelve integer types, and they can be categorized into signed integers (positive and negative values) and unsigned integers (only positive values).
Your age cannot be a negative number. The miles to your home from work cannot be a negative number.
Here are the Rust integer types:
Type | Size (bits) | Min Value | Max Value |
i8 | 8 | −1.28 × 10² | 1.27 × 10² |
u8 | 8 | 0 | 2.55 × 10² |
i16 | 16 | −3.28 × 10⁴ | 3.27 × 10⁴ |
u16 | 16 | 0 | 6.55 × 10⁴ |
i32 | 32 | −2.14 × 10⁹ | 2.14 × 10⁹ |
u32 | 32 | 0 | 4.29 × 10⁹ |
i64 | 64 | −9.22 × 10¹⁸ | 9.22 × 10¹⁸ |
u64 | 64 | 0 | 1.84 × 10¹⁹ |
i128 | 128 | −1.70 × 10³⁸ | 1.70 × 10³⁸ |
u128 | 128 | 0 | 3.40 × 10³⁸ |
isize | depends | depends | depends |
usize | depends | depends | depends |
Signed integers (positive and negative value) are represented by the letter “i” followed by the number of bits the type can hold.
Unsigned integers (positive values only) are represented by the letter “u” followed by the number of bits the type can hold.
So i8 is a signed 8-bit integer that can hold values from -128 to 127.
u8 is an unsigned 8-bit integer that can hold values from 0 to 255.
You specify a negative number in Rust by prefixing it with the minus sign (-), which is the unary negation operator.
If you do not specify a type for a variable, the Rust compiler defaults to i32 for integers. So if you intend to use a number outside the range that can be stored in an i32 integer (less than -2,147,483,648 or greater than 2,147,483,647), you’ll need to explicitly specify the type (i64, u32, u64, etc).
isize and usize
Note that the isize and usize integer types are platform-specific, at compile-time, not at run-time. That means that if the program is compiled on a 32-bit architecture computer, then the size of the isize type is i32 and the usize type is u32, and if compiled on a 64-bit architecture computer, then the size of the isize type is i64 and the usize type is u64.
isize and usize types are primarily used for pointer-sized values — that is, values related to memory addressing and indexing. Don’t worry about exactly what that means, in this post you don’t need to know.
Floating Point Types
Floating point types are scalars that are numbers that do have a fractional part, a decimal point and one or more numbers after the decimal point. Examples include:
25.64
376.20487
2,601.5
346,204,550.330040
Rust has only two floating point types, and they are both signed types.
Here are the Rust floating point types:
Type | Size (bits) | Approx. Range | Precision (decimal digits) |
f32 | 32 | ±1.18 × 10⁻³⁸ to ±3.4 × 10³⁸ | ~6–7 digits |
f64 | 64 | ±2.23 × 10⁻³⁰⁸ to ±1.80 × 10³⁰⁸ | ~15–17 digits |
Floating point types are represented by the letter “f” followed by the number of bits the type can hold.
If you do not specify a type for a variable, the Rust compiler defaults to f64 for floating point numbers. So if you know the floating point numbers you will be using in a situation is within the range of an f32, you can save memory by explicitly specifying the type as f32.
Note that f32 is called single precision (IEEE 754), and f64 is called double precision.
Boolean Type
The Rust boolean type, bool, is a scalar type that represents a value of either true or false. It’s typically used to indicate whether a condition is met or not, and is commonly used in control flow statements like if, while, and logical expressions.
Examples include:
let active: bool = true;
let expired: bool = false;
In future posts we’ll see many examples of booleans in action.
Character Type
The Rust char
type is a scalar type that represents a single Unicode scalar value. This includes not only ASCII characters like 'A'
or '9'
, but also symbols, emojis, and characters from other languages, such as 'ß'
, '中'
, or '🚀'
.
Examples include:
let choice: char = 'Y';
let currency: char = '€';
let smiley: char = '😊';
let letter: char = '中';
Unit Type
The Rust unit type () is a scalar type that represents the absence of a meaningful value. It has exactly one value: (). It’s often used as the return type for functions that don’t return anything, or as a placeholder when a value is required but no data needs to be stored.
Think of ()
as a type-safe version of “nothing” — it’s not null
, and it’s not undefined. It’s used when the type system expects something, but you don’t have anything meaningful to return or store.
Example:
let mut flags: HashMap<&str, ()> = HashMap::new();
flags.insert("loaded", ());
flags.insert("cached", ());
if flags.contains_key("cached") {
println!("Item is cached.");
}
Don’t worry about what this code does for now, just know that we are using the unit type here as a type of flag to indicate if something has been loaded and / or cached.
&str The String Slice Type
The &str type in Rust is a borrowed string slice — a lightweight, read-only reference to a sequence of UTF-8 characters. It’s ideal for efficiently working with text without owning or duplicating it. String literals are &str by default, and slicing a String returns a &str that avoids extra allocations.
Examples include:
let greeting: &str = "Hello, world!";
let s = String::from("Rustacean");
let slice: &str = &s[0..4]; // "Rust"
Don’t worry too much about the string slice type for now. In a future post we’ll have an indepth discussion of the &str string slice type and the String type, and there are definitely some tricky aspects of that discussion.
Compound Types – Tuples and Arrays
In Rust, the only two primitive compound types are tuples and arrays. Tuples can hold values of different types, while arrays store a fixed number of values of the same type.
Examples include:
let name_age_tuple: (&str, u8) = ("Bob", 34);
let scores: [u8; 20] = [0; 20];
Notice the name_age tuple is defined using parentheses, and in this case it is defined to hold a string slice and a u8. You might define a tuple to hold three or five or more values. A key point is that the items in the tuple can be of different types.
scores on the other hand is an array, perhaps representing students scores on a test. Here we declare that scores is an array of 20 u8 numbers, and we initialize the array to contain 20 items whose initial values are 0.
We’ll get deeper into tuples and arrays in future posts, but for now know you can access the items in a tuple with this syntax:
let age = name_age_tuple.1; // access second item
And you can access array items with this syntax:
let bobs_score = scores[4] // access fifth item
Items in tuples and arrays are accessed using zero-based indexes. So above .1 means access the second item, and [4] means access the fifth item. We use “dot notation” to access tuple items, and square bracket notation to access array items. The 1 and the 4 are referred to as indexes.
A Note on Inferred Types
Note that in the provided code showing examples of all the data types we have covered we specify the data type for each variable. We could omit the type specifiers, but for some of the variables, the type inferred by the compiler might not be what you expect or want.
The only ones you need to be concerned with are integers and floating point numbers. The Rust compiler defaults to i32 for integers and f64 for floating point numbers.
If your variable is for people’s ages, you should use a u8 because it saves space and people’s ages cannot be negative. So it’s okay to rely on the Rust compiler to infer data types, but be aware that in some situations you might want to specify the type.
Example of Compiler Errors
Before we wrap up this post, I’d like to show you an example of a compiler error you might get. If you’re using VS Code as your IDE and if you have the Rust Analyzer VS Code extension installed, then you can follow along, otherwise this will be for future reference.
In the example code, go ahead and remove the type specification for this line:
let unsigned_even_larger_number: u64 = 12_446_744_073_709_551_615;
So it looks like this:
let unsigned_even_larger_number = 12_446_744_073_709_551_615;
You should see this squiggly red line indicating there is an error:

If you hover over the error you see this:

Because the Rust compiler defaults to i32 when inferring integer types, the value being assigned to the variable will not fit in an i32 data type. It suggests using i128, though before we made this change the type we were using was u64 which is fine too.
With the change above removing the u64 type specification, you can execute a cargo run and see the error in the terminal as well.

So feel free to play around with this code, perhaps observing error messages, and trying to figure out how to fix the errors.
Wrapping Up…
So this rather long post introduced the topic of Rust variables and data types. We’ve only scratched the surface on these topics, so look forward to upcoming posts where we continue our journey toward Rust mastery.
Thanks for stopping by!
Leave a Reply