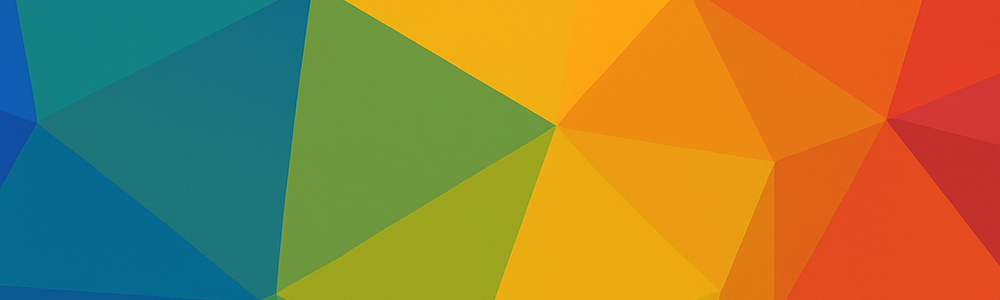
In Rust, traits
are the idiomatic way to define shared behavior—think interfaces with superpowers. Whether you’re building web apps, embedded systems, or anything in between, traits give you the tools to design clean, reusable abstractions. They’re conceptually similar to interfaces
in languages like Java or TypeScript, but with some important differences.
While interfaces in many languages allow you to declare methods and properties that implementing types must provide, Rust traits allow you to define only methods and associated functions, but not fields. Rust doesn’t support defining variables or data members in traits.
Traits in Rust act like a contract: if a type, such as a struct
or enum
, claims to implement a trait, it must provide implementations for the required methods. If it doesn’t, the code won’t compile.
One feature that sets Rust traits apart is default method implementations. When you define a trait, you can provide a default implementation for any method or associated function (which are like static methods). Then, when a type implements that trait, it can either use the default or override it with its own version.
To be clear, traits can be implemented on the following:
Structs | Enums | Unions (with some restrictions) | Tuples | Arrays and slices |
Primitive types (like i32 , f64 , etc.) | References (e.g., &T , &mut T ) | Function pointers (e.g., types like fn(i32) -> i32 ) | Generic types and type parameters | Your custom types |
Topics We’ll Cover
- Declaring traits, and default implementations
- Implementing traits on types
- Deriving traits
- Marker traits and auto traits, a quick detour
- Trait bounds
- Traits as function parameters
- Returning types that implement traits
- Associated types
- Trait objects and dynamic dispatch
- Super traits
- Advanced trait features
Declaring traits, and default implementations
Let’s get started with traits. Often your traits, (and structs, enums, etc.) will be declared in a library crate, so let’s create one.
Open a shell window (Terminal in Mac/Linux, Cmd or PowerShell in Windows).Then navigate to the directory where you store Rust packages
for this blog, and run the following command:
cargo new traits_demo --lib
Then change into the newly created traits_demo
directory, and open that directory in VS Code or your favorite IDE.
Note: Using VS Code will make it easier to follow along with this blog series. Installing the Rust Analyzer extension is also highly recommended.
Also, opening the traits_demo
directory allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the traits_demo
directory in VS Code.
Open the file src/lib.rs and replace its contents entirely with this code:
pub trait Payable {
fn get_salary(&self) -> f32; // Required method
fn pay(&self) { // Default method
println!("It's payday! You got paid: {}", self.get_salary() / 52.0);
}
}
Here we declare a trait named Payable
. The purpose of this trait is to define methods used to pay employees. Note that we use the pub
keyword before the trait keyword, so our trait will be public. This makes it visible to other modules and crates, which is necessary when using the trait in your main.rs
binary crate.
You declare a trait with the trait
keyword, the name of the trait, and then curly braces. In the body of the trait declaration you declare your trait’s methods. Here we’ve declared a get_salary() method that takes a reference to self
, and returns an f32
.
Notice that we do not provide an implementation for the get_salary() method, just the method signature followed by a semicolon. This is referred to as a method stub. Types implementing the Payable trait will need to implement the get_salary() method, otherwise a compilation error will occur.
Our trait also declares a pay() method, but this time we provide a default implementation
. Types implementing our trait can define their own implementation for the pay() method, or they can simply use this default implementation. We’ll see examples of both in a moment.
Traits you define can have as many methods as you want, some can simply be stubs, others can have default implementations. It will depend on the purpose and use of the trait.
Our two trait methods automatically receive the &self
reference. &self
is a reference to the instance of the type for which this trait will be implemented. We use a reference because we don’t want our trait methods to take ownership of the type instance, we just want to borrow it.
If you need a refresher on ownership, this ByteMagma blog post might help: Ownership, Moving, and Borrowing in Rust
You might ask, why doesn’t the pay() method simply do something like this:
fn pay(&self) {
println!("It's payday! You got paid: {}", self.salary / 52.0);
}
Types that implement this trait will have a salary field, but the trait doesn’t know this. Traits can’t assume the presence of fields in the implementing type. So defining a get_salary() method in the trait allows each type to provide its own logic to access the appropriate field, regardless of its name.
Implementing traits on types
Now we’ll define two structs, Employee
and Manager
that will implement this Payable
trait. Employees are paid just a salary and managers are paid their salary and a bonus.
Add the following code in lib.rs for our Employee and Manager structs, below the code for our trait. Note that these structs could be defined above our trait, but placing them below the trait they implement makes logical sense.
pub struct Employee {
pub name: String,
pub salary: f32,
}
pub struct Manager {
pub name: String,
pub salary: f32,
pub bonus: f32,
}
Both these structs have a salary field, but only the manager has the bonus field, as Employees don’t receive bonuses.
Now let’s implement the Payable trait for these two structs.
Add the following code to lib.rs below these struct definitions:
impl Payable for Employee {
fn get_salary(&self) -> f32 {
self.salary
}
// uses default pay()
}
impl Payable for Manager {
fn get_salary(&self) -> f32 {
self.salary
}
// custom implementation of the pay() method
fn pay(&self) {
let total = (self.get_salary() / 52.0) + (self.bonus / 52.0);
println!("Manager {} got paid (with bonus): {}", self.name, total);
}
}
Note: If you still want to use the trait’s default method inside a custom one, you can use fully qualified syntax like Payable::pay(self);
.
fn pay(&self) {
println!("Custom pay logic");
Payable::pay(self); // Calls the default implementation
}
To implement a trait for a type, we start with the impl
(implementation) keyword, then the name of the trait to implement, then for TYPE
, where TYPE is the type for which the trait will be implemented:
impl Payable for Employee {
impl Payable for Manager {
We’re implementing the Payable
trait for the Employee
and Manager
structs.
Then we have curly braces that enclose the implementation block.
We implement the get_salary() method for the Employee and Manager structs so the trait has access to the salary
field.
But we only implement the pay() method for the Manager struct. The Employee struct will use the Payable
trait default implementation of the pay() method.
fn pay(&self) {
let total = (self.get_salary() / 52.0) + (self.bonus / 52.0);
println!("Manager {} got paid (with bonus): {}", self.name, total);
}
The custom implementation of the pay() method for the Manager struct calculates the total amount paid (salary + bonus), and then prints out a custom message that includes the manager’s name. We divide by 52.0 to get the weekly salary and weekly bonus amounts.
You might be thinking, why can’t we print out the Employee’s name as well? We could add a get_name() method to the trait to make that possible, but for now, we’re keeping our focus on salary logic.
// compilation error, trait cannot access name field on type instance
fn pay(&self) {
println!("Employee {} got paid: {}", self.name, self.get_salary() / 52);
}
Remember, the trait default implementation can’t access the struct fields (self.name). We could add a get_name() method to the trait but for now we’ll leave it as it.
Let’s now create a binary crate to simulate creating type instances and calling the pay() method on each.
This should be your current folder structure for the package:
traits_demo/
├── Cargo.toml
└── src/
└── lib.rs
Inside the src
directory, add a file main.rs.
your_project_name/
├── Cargo.toml
└── src/
├── lib.rs
└── main.rs
Add this code to main.rs:
// bring in Employee, Manager and Payable from lib.rs
use traits_demo::{Employee, Manager, Payable};
fn main() {
let emp = Employee {
name: "Alice".to_string(),
salary: 52_000.00,
};
let mgr = Manager {
name: "Bob".to_string(),
salary: 68_000.00,
bonus: 10_000.00,
};
emp.pay();
mgr.pay();
}
Execute cargo run and you should get this output:
It's payday! You got paid: 1000
Manager Bob got paid (with bonus): 1500
Congratulations! You created your first trait, implemented it for two types, and saw it in action!
Deriving traits
You can “derive” some traits implemented in the Rust standard library with the following syntax:
#[derive(Debug, Clone, PartialEq)] // derive three traits
#[derive(Debug)] // derive one trait
derive
is a Rust attribute
. Rust attributes are metadata applied to structs, enums, functions, modules, etc. Attributes influence how the Rust compiler “handles” the item.
Attributes start with an # and are enclosed in square brackets. Attributes instruct the compiler to do something special, enabling conditional compilation, tweaking lint behavior, automatically implementing common traits
, etc.
Here are some common built-in attributes:
#[cfg(...)]
— for conditional compilation
#[allow(...)]
/ #[warn(...)]
— for linting control
#[derive(...)]
— for automatic trait implementation (our current topic!)
The #[derive(...)]
attribute tells the compiler to automatically implement one or more traits in the Rust standard library for a struct, enum, etc. for things like comparisons, cloning or debugging.
Example:
#[derive(Debug, Clone, PartialEq)]
struct Point {
x: i32,
y: i32,
}
The compiler will generate implementations for the Debug
, Clone
, and PartialEq
traits behind the scenes. This means you can:
- Print a
Point
usingprintln!("{:?}", point)
- Clone a
Point
withlet p2 = p1.clone();
- Compare points with
==
or!=
⚠️ Note: Some traits have extra requirements. For example, Copy
can only be derived if all fields in the type also implement Copy
. If even one field doesn’t (like a String
or Vec<T>
), the compiler will raise an error.
Traits like Clone
and Debug
are more forgiving — they can be derived as long as their required behavior is defined for all fields, and most standard types already support them.
This explains why you might sometimes get compiler errors when trying to #[derive(Copy)]
but not with #[derive(Clone)]
. Rust is keeping you safe by ensuring bitwise copying is only done when truly valid for all fields.
If the default implementation these traits provide are not sufficient, you can always implement them for your type.
Example:
use std::fmt;
struct Point {
x: i32,
y: i32,
}
impl fmt::Debug for Point {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
f.debug_struct("Point")
.field("x", &self.x)
.field("y", &self.y)
.finish()
}
}
impl fmt::Display for Point {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
write!(f, "({}, {})", self.x, self.y)
}
}
fn main() {
let p = Point { x: 3, y: 7 };
println!("Display: {}", p); // Uses Display
println!("Debug: {:?}", p); // Uses Debug
println!("Pretty Debug:\n{:#?}", p); // Pretty Debug output
}
/*
Output:
Display: (3, 7)
Debug: Point { x: 3, y: 7 }
Pretty Debug:
Point {
x: 3,
y: 7,
}
*/
The above code shows how you might implement the fmt()
method of the Display
and Debug
standard library traits to customize how data is printed.
If you want to run this code, add a bin directory in the src directory of the current package we’ve been working with, create a file src/bin/main2.rs, copy/paste the above code to main2.rs and execute cargo run --bin main2
Marker Traits and Auto Traits: A Quick Detour
While discussing #[derive(...)]
, it’s helpful to know that not all traits exist to provide methods. Some traits act as flags or indicators, and these fall into two interesting categories: marker traits and auto traits.
Marker Traits
Marker traits are traits with no methods. They simply mark a type with a particular capability. For example:
Copy
: The type can be duplicated with a simple memory copy (no deep clone needed).Clone
: The type can be explicitly cloned with.clone()
.Eq
andPartialEq
: The type can be compared for equality.Default
: The type has a default value viaT::default()
.
Many of these can be derived, which makes them super convenient:
#[derive(Copy, Clone, Debug, PartialEq)]
struct Point {
x: i32,
y: i32,
}
Now you can:
- Copy
Point
instances withlet p2 = p1;
- Clone explicitly with
p1.clone()
- Compare with
==
and!=
- Print for debugging with
{:?}
All of this with zero manual implementation!
Auto Traits
Auto traits are traits that the Rust compiler automatically applies to your types based on their fields. You don’t implement or derive them—they’re applied behind the scenes if your type qualifies.
Examples of auto traits:
Send
: Safe to transfer between threads.Sync
: Safe to share across threads.Unpin
: Safe to move after being pinned (used in async code).
You’ll typically encounter these traits in multithreading or async programming. For example:
use std::thread;
fn is_send<T: Send>() {}
fn main() {
// i32 is Send, so this compiles fine
is_send::<i32>();
// Rc<T> is NOT Send — you'd get a compile-time error if you try this:
// is_send::<std::rc::Rc<i32>>();
}
This shows that the compiler enforces safety by automatically applying or rejecting auto traits based on your type’s contents.
Knowing about marker and auto traits gives you a deeper appreciation for how Rust handles type safety, memory, and concurrency under the hood—all while still being ergonomic to use via #[derive(...)]
.
Trait bounds
In Rust, trait bounds are all about placing constraints on generics. We’ll explore generics more thoroughly in a future post, but for now, just know that generics allow you to use a placeholder for a type—one that gets filled in when you actually use a struct, enum, trait, or function in your code.
You’ll get a light introduction to generics in this section, especially in the context of trait bounds. If you’re curious how generics are used in common types like Option
and Result
, check out this ByteMagma post: Error Handling in Rust: Panics, Result, and Option
Why Trait Bounds Matter
Rust lets us write generic functions and generic types that work with any type, which is incredibly powerful. Even without any constraints, generics allow us to write flexible, reusable code that avoids duplication.
But sometimes you want to write code that only works with generic types that implement specific traits. Perhaps your code needs to ensure the generic type can be printed (Debug trait), cloned (Clone), or compared (PartialEq).
Rust needs proof that the type can actually do those things.
That’s where trait bounds come in. They let you say:
“This type can be anything — as long as it implements a specific trait.”
Trait bounds make generic code more than just flexible, they make your code truly functional — giving you the power to write logic that works across types while still ensuring the capabilities you need.
Motivation: Generic Functions Needing Certain Capabilities
Imagine we want to write a function that prints anything. You might try:
// without a trait bound
fn print_item<T>(item: T) {
println!("{}", item);
}
But this won’t compile. Why? Because Rust doesn’t know whether T
implements the Display
trait — the one required for println!
with {}
formatting.
To fix it, we constrain T
with a trait bound:
// with a trait bound
use std::fmt::Display;
fn print_item<T: Display>(item: T) {
println!("{}", item);
}
Now Rust knows that T
must implement the Display
trait — so printing works just fine.
Syntax for Trait Bounds
Single Trait Bound
The most basic form of a trait bound applies a single constraint to a generic type.
Here’s a simple example using PartialEq
to compare two values:
fn are_equal<T: PartialEq>(a: T, b: T) -> bool {
a == b
}
This tells Rust that T
must implement the PartialEq
trait so it can be compared using ==
.
Here’s another simple example using the Debug
trait:
use std::fmt::Debug;
fn print_debug<T: Debug>(item: T) {
println!("{:?}", item);
}
This works with any type that implements the Debug
trait, allowing it to be formatted with the {:?}
placeholder.
Multiple Trait Bounds
Use the +
operator to specify multiple trait bounds:
fn process<T: Display + Clone>(item: T) {
println!("{}", item.clone());
}
Here, T
must implement both Display
and Clone
.
Here’s another example using the +
operator to combine multiple traits:
fn describe<T: Display + PartialEq>(a: T, b: T) {
println!("a: {}, b: {}", a, b);
if a == b {
println!("They’re equal!");
}
}
This function works with any type that can be printed and compared for equality.
Where Clauses for Readability
Long lists of multiple trait bounds using the + operator can make the function signature complex and messy.
The where
clause improves readability of your code.
// using the + operator
fn process<T: Display + Clone, U: Debug + Default>(t: T, u: U) {
// ...
}
// using a where clause
fn process<T, U>(t: T, u: U)
where
T: Display + Clone,
U: Debug,
{
println!("{}, {:?}", t.clone(), u);
}
Same logic, cleaner layout — especially helpful when using multiple generic types.
Trait Bounds with Structs and Enums
Trait bounds aren’t just for functions — they also apply to generic types like structs and enums.
Generic Struct with a Trait Bound
struct Wrapper<T: Display> {
value: T,
}
impl<T: Display> Wrapper<T> {
fn print(&self) {
println!("{}", self.value);
}
}
This enforces that Wrapper<T>
can only be created with types that implement Display
.
Enums Work Similarly
enum ResultWrapper<T: Debug> {
Success(T),
Error(String),
}
Using impl Trait
Syntax
The impl Trait
syntax is a shortcut — it lets you skip the generic type parameter entirely.
Instead of:
fn print_item<T: Display>(item: T) {
println!("{}", item);
}
You can write:
fn print_item(item: impl Display) {
println!("{}", item);
}
So instead of specifying a generic type with a trait bound, you add impl Display as the function parameter type.
The impl Trait syntax is cleaner and easier to read when you only use the type once.
So if you would do this with generics:
fn print_item<T: Display>(item: T) {
println!("{}", item);
}
Instead you can do this with the impl Trait syntax:
fn print_item(item: impl Display) {
println!("{}", item);
}
When to Use It
Use impl Trait
when:
- You don’t need to refer to the generic type again
- You want more concise function signatures
Stick with explicit T: Trait
syntax if:
- You need to refer to the generic type multiple times (e.g., return values)
- You have multiple generic parameters and want flexibility
// referring to the generic type multiple times (function parameter and return type)
fn identity<T: Clone>(item: T) -> T {
item
}
// multiple generic parameters (T and U)
fn convert<T, U>(input: U) -> T
where
T: From<U>,
{
T::from(input)
}
We’ll explore impl Trait
more in the next two sections on function parameters and return types.
Traits as function parameters
In the previous section, we introduced trait bounds and how they constrain generic types. One of the most common (and powerful) ways to use trait bounds in real Rust code is in function parameters. This section focuses entirely on that use case — and dives into when to use impl Trait
vs. T: Trait
.
Why Use Traits as Function Parameters?
Functions often need to work with values that exhibit certain behavior — such as being printable, clonable, or serializable. Instead of specifying a concrete type, we can accept any type that implements a specific trait.
This enables:
- Polymorphism (behavior-based flexibility)
- Generic, reusable code
- Cleaner APIs
Option 1: Using impl Trait in Parameters
The simplest and most readable way to accept a value that implements a trait is with impl Trait:
use std::fmt::Debug;
fn print_debug(item: impl Debug) {
println!("{:?}", item);
}
This means: “Accept any type that implements the Debug trait.”
Advantages:
- Easy to read
- No need to name a generic type
- Great when the trait is only used in the parameter list
Option 2: Using a Named Generic with a Trait Bound
You can also use a named generic and constrain it with a trait:
use std::fmt::Debug;
fn print_debug<T: Debug>(item: T) {
println!("{:?}", item);
}
This does the same thing as the impl Trait version above — but gives you a named type (T) that you can refer to elsewhere.
When to use:
- You want to use the type more than once
- You’re also returning the type
- You want to relate multiple types
When impl Trait and T: Trait Are Interchangeable
If the trait only appears once (usually just in a parameter), then both styles are equivalent in function and performance:
fn greet(name: impl AsRef) {
println!("Hello, {}!", name.as_ref());
}
Equivalent to:
fn greet<T: AsRef>(name: T) {
println!("Hello, {}!", name.as_ref());
}
In these cases, pick whichever style is cleaner or matches your team’s conventions.
When You Must Use a Named Generic
You’ll need a named generic (T) when:
You refer to the type in more than one place:
fn identity<T: Clone>(value: T) -> T {
value.clone()
}
Using impl Trait here wouldn’t work, because you need the same type for both the input and output.
Or when you need to relate multiple types:
fn convert<T, U>(input: U) -> T
where
T: From,
{
T::from(input)
}
This expresses a relationship between two generic types — something impl Trait can’t do.
Multiple Trait Bounds in Parameters
You can still use multiple traits with either syntax:
With impl Trait:
use std::fmt::Debug;
fn log_info(item: impl Debug + Clone) {
println!("{:?}", item.clone());
}
With T: Trait1 + Trait2:
use std::fmt::Debug;
fn log_info<T: Debug + Clone>(item: T) {
println!("{:?}", item.clone());
}
Trait Bounds vs Trait Objects
This section is about static dispatch
using trait bounds. Later in this post, we’ll explore trait objects (e.g., Box) and dynamic dispatch
, which gives even more flexibility — at a small runtime cost.
So when accepting trait-based parameters:
Use impl Trait for simplicity and readability — especially when the trait is used once.
Use T: Trait when you need flexibility, multiple usages, or type relationships.
Both forms give you zero-cost abstractions via static dispatch.
Returning types that implement traits
You can also specify a function return type as a type that implements a trait.
But returning a type that implements a trait has some constraints and rules that differ from using traits as function parameters. In this section, we’ll explore two primary approaches:
impl Trait
in return position (static dispatch)Box<dyn Trait>
(dynamic dispatch)
We’ll also cover when each approach is appropriate, with clear examples.
The Problem: You Can’t Return a trait
by Itself
Let’s say you write a function like this:
use std::fmt::Display;
fn get_printable() -> Display {
// This won't compile!
}
Rust will complain, because you’re trying to return a trait type directly. But a trait is not a concrete type — it’s just a contract. So Rust needs more context.
That leads us to our first solution…
Returning impl Trait
(Static Dispatch)
The most common and idiomatic way to return a value that implements a trait is using impl Trait
in the return type.
use std::fmt::Display;
fn get_message() -> impl Display {
"This is a message."
}
This works perfectly because Rust can see at compile time that the function returns a string slice (&'static str
), which implements the Display
trait.
Key Points:
- The actual return type is known at compile time.
- The trait is used as a bound type, not an actual type.
- This is called static dispatch — no runtime cost.
You can think of it like this:
“The caller doesn’t need to know the exact type — just that it implements
Display
.”
You Can’t Return Multiple Concrete Types with impl Trait
Here’s a common mistake:
fn random_message(condition: bool) -> impl Display {
if condition {
"Hello"
} else {
42 // won't compile — different types!
}
}
Rust won’t allow this, even though both "Hello"
and 42
implement Display
, because the compiler needs to know the return type is the same type in all branches.
If you need to return one of several types that all implement a trait, use a trait object
.
Returning Box<dyn Trait>
(Dynamic Dispatch)
When you need to return values of different types that all implement the same trait, you can use trait objects and heap allocation:
use std::fmt::Display;
fn get_dynamic_message(condition: bool) -> Box<dyn Display> {
if condition {
Box::new("Hello")
} else {
Box::new(42)
}
}
How this works:
Box<dyn Display>
is a trait object.- The actual value is stored on the heap, and accessed via a fat pointer.
- Rust uses dynamic dispatch at runtime to call the correct method.
Box<dyn Display>
is a smart pointer that stores the value on the heap. Internally, it uses a fat pointer — a special kind of pointer that includes both a reference to the value and a reference to a vtable for dynamic dispatch.
Think of it like:
“I’m returning something that implements
Display
, but I don’t know what it is until runtime.”
We’ll discuss vtables in the section discussing Trait Objects in detail.
Static vs Dynamic Dispatch Summary
Feature | impl Trait | Box<dyn Trait> |
---|---|---|
Dispatch Type | Static (compile-time) | Dynamic (runtime) |
Performance | Zero-cost | Slight runtime overhead |
Return Type Flexibility | Must be a single concrete type | Can be multiple types |
Ownership | Stored on the stack | Heap-allocated via Box |
Trait Requirements | Trait must be Sized | Trait must be object-safe |
When to Use Which?
- Use
impl Trait
if:- You always return the same type
- You care about performance
- You want simplicity
- Use
Box<dyn Trait>
if:- You need to return multiple types that implement the same trait
- You’re writing plugin-style or runtime-determined logic
- You’re okay with heap allocation and slight overhead
Real-World Style Example
Let’s say you’re building a logger. You might want to return a log writer:
trait LogWriter {
fn write(&self, msg: &str);
}
struct Console;
impl LogWriter for Console {
fn write(&self, msg: &str) {
println!("Console: {}", msg);
}
}
struct FileLogger;
impl LogWriter for FileLogger {
fn write(&self, msg: &str) {
println!("(pretend this is writing to a file): {}", msg);
}
}
// Return a trait object
fn get_logger(use_file: bool) -> Box<dyn LogWriter> {
if use_file {
Box::new(FileLogger)
} else {
Box::new(Console)
}
}
Now you can call:
let logger = get_logger(true);
logger.write("Hello!");
This is dynamic dispatch in action. get_logger() returns a Box<dyn LogWriter>. Because of the if-else
the compiler doesn’t know which type (FileLogger or Console) will be returned at runtime, but its okay, the compiler will use the vtable to know which write() method implementation to use.
To summarize, you can return trait-constrained values in two main ways:
impl Trait
(static dispatch):
Great when the return type is known and consistent — fast and ergonomic.Box<dyn Trait>
(dynamic dispatch):
Use when flexibility is required and types may vary.
Both are powerful tools in Rust’s trait system. Choose based on your use case — compile-time simplicity or runtime flexibility.
Associated types
When designing traits, it’s common to have a method that returns or uses a related type — something that’s not generic in the usual way, but still needs to vary per implementation. Rust gives us a way to express this kind of relationship using associated types — named placeholders within a trait that get filled in by each implementation.
This concept can feel a little abstract at first — especially if you’re used to plain generics. But don’t worry — the next few examples will walk you through it to make things more clear. Once it clicks, you’ll see how associated types make traits more expressive and your type signatures much cleaner.
What Are Associated Types?
Associated types are type placeholders defined within traits. Unlike generic type parameters (<T>
), associated types are bound to the trait itself, not to individual methods.
Think of it like this:
“This trait defines a type called
Item
, and each implementation will decide whatItem
actually is.”
A Basic Example: An Iterator Trait
Rust’s built-in Iterator
trait is a perfect example:
pub trait Iterator {
type Item;
fn next(&mut self) -> Option<Self::Item>;
}
Here:
Item
is an associated typeSelf::Item
is used to define the return type ofnext()
Implementing a Trait with an Associated Type
Let’s implement a simplified version of Iterator
:
struct Counter {
current: usize,
max: usize,
}
impl Iterator for Counter {
type Item = usize;
fn next(&mut self) -> Option<Self::Item> {
if self.current < self.max {
let result = self.current;
self.current += 1;
Some(result)
} else {
None
}
}
}
Here, in the implementation of Iterator for our struct Counter, type Item = usize;
tells Rust that for this implementation, Self::Item
will be usize
.
Why Use Associated Types?
You could write this trait using generics instead:
trait Iterator<T> {
fn next(&mut self) -> Option<T>;
}
But this gets messy when:
- You want to use the trait object (
dyn Iterator
) — generic parameters don’t work here - You want to constrain or relate associated types across multiple traits
So Rust prefers the associated type form for clarity and object safety.
Example: A Key-Value Store
Associated types are really useful when one trait needs to define related types that aren’t interchangeable across implementations.
trait KeyValueStore {
type Key;
type Value;
fn set(&mut self, key: Self::Key, value: Self::Value);
fn get(&self, key: &Self::Key) -> Option<&Self::Value>;
}
Now implement it for a simple in-memory map:
use std::collections::HashMap;
struct MemoryStore {
map: HashMap<String, String>,
}
impl KeyValueStore for MemoryStore {
type Key = String;
type Value = String;
fn set(&mut self, key: Self::Key, value: Self::Value) {
self.map.insert(key, value);
}
fn get(&self, key: &Self::Key) -> Option<&Self::Value> {
self.map.get(key)
}
}
This version is clean, intuitive, and flexible.
Associated Types vs Generics
Let’s compare:
Using Generics (less ergonomic for traits):
trait Store<K, V> {
fn insert(&mut self, key: K, value: V);
}
Using Associated Types:
trait Store {
type Key;
type Value;
fn insert(&mut self, key: Self::Key, value: Self::Value);
}
Associated types:
- Make trait objects possible (
dyn Store
) - Avoid polluting method signatures with
<K, V>
- Are easier to constrain and relate across traits
Constraining Associated Types with Trait Bounds
You can constrain associated types just like regular ones:
trait PrintableIterator {
type Item: std::fmt::Display;
fn next(&mut self) -> Option<Self::Item>;
}
This enforces that Item
must implement Display
.
Associated Types and Trait Objects
Associated types allow you to create trait objects (e.g., Box<dyn Iterator<Item = T>>
), which isn’t possible with generic parameters:
This works:
fn use_iterator(it: &mut dyn Iterator<Item = i32>) {
while let Some(val) = it.next() {
println!("Got: {}", val);
}
}
But this would fail with Iterator<T>
— trait objects can’t have generic parameters.
When to Use Associated Types
Use associated types when:
- You’re defining a trait that will always return or use a specific type per implementation
- You want cleaner method signatures
- You want to use trait objects (
dyn Trait
) - You want to constrain the associated type with bounds (
type Item: Display
)
They’re a key part of idiomatic trait design in Rust — and once you understand them, other powerful traits like Iterator
, IntoIterator
, Deref
, Fn
, etc. become a lot more clear.
Trait objects and dynamic dispatch
Earlier sections in this post showed how to return values that implement traits — and how to use Box<dyn Trait>
when the return type might vary. That was your first encounter with trait objects and dynamic dispatch.
In this section, we’ll learn more about these concepts and gain a deeper understanding of how they work.
Trait objects are a powerful part of Rust — enabling runtime polymorphism for flexible designs like plugin systems, collections whose items are of different types, and behavior-driven APIs.
What Is a Trait Object?
A trait object is a value of type &dyn Trait
, Box<dyn Trait>
, or Rc<dyn Trait>
, etc.
- It holds a pointer to some value, and a pointer to a vtable (virtual method table).
- That vtable is what enables dynamic dispatch — Rust looks up the method implementation at runtime.
A trait object lets you work with values of different types that implement the same trait — even when you don’t know the concrete types at compile time.
use std::fmt::Display;
let item: Box<dyn Display> = Box::new("Hello");
Here:
dyn Display
is the trait object.Box
handles heap allocation and dynamic dispatch via a vtable.- You can call any method defined in the
Display
trait onitem
, even though the exact type (&str
) is erased at runtime.
Rust “erases” the specific type (&str
) and instead stores:
- A pointer to the value (heap-allocated, thanks to
Box
) - A pointer to a vtable that knows how to call
Display::fmt
for this type
Box<dyn Display>
can contain values of different types, such as an earlier example with an if-else
where the return type could not be known at compile time.
So Rust creates a vtable (virtual method table) — a small lookup table of function pointers to the Display
trait’s method implementations for each type stored via the box (&str
in this case).
Note: This vtable-based dynamic dispatch only occurs when you use trait objects like Box<dyn Trait>
, &dyn Trait
, or Rc<dyn Trait>
. If you’re using generics or impl Trait
, Rust uses static dispatch, which resolves the method calls at compile time — no vtable, no runtime cost.
When you call a method like:
println!("{}", item);
Rust:
- Looks in the vtable to find the right
Display::fmt
function for the type of item - Calls that function on the value
What Is Dynamic Dispatch?
Dispatch refers to how a method call is resolved. Rust has two main types:
- Static dispatch:
The compiler knows the exact type and generates direct function calls. This is whatimpl Trait
and generic functions use — it’s fast and inlined. - Dynamic dispatch:
The compiler doesn’t know the exact type. It uses a vtable (virtual method table) to look up the correct method at runtime.
Example: Static vs Dynamic Dispatch
trait Speak {
fn speak(&self);
}
struct Dog;
struct Cat;
impl Speak for Dog {
fn speak(&self) { println!("Woof!"); }
}
impl Speak for Cat {
fn speak(&self) { println!("Meow!"); }
}
Static dispatch:
// compiler knows animal is of type T
fn call_speak<T: Speak>(animal: T) {
animal.speak(); // resolved at compile time as the type is known
}
Dynamic dispatch with trait object:
// compiler doesn't know type of animal, just that it implements the Speak trait
fn call_speak_dyn(animal: &dyn Speak) {
animal.speak(); // resolved at runtime via vtable as type is not known
}
What Makes a Trait Object-Safe?
Not every trait can be turned into a trait object. Rust requires object safety, which ensures the compiler can safely use a trait at runtime without knowing the concrete type.
A trait is object-safe if:
- All methods use
&self
,&mut self
, orself
(noSelf
in return types). - It doesn’t use generic methods.
Not object-safe:
trait NotSafe {
fn make() -> Self; // uses Self in return type
}
Object-safe version:
trait Safe {
fn do_it(&self); // fine
}
This ensures trait objects like Box<dyn Safe>
can actually work.
Using Trait Objects in Structs and Collections
You can store trait objects in struct fields, boxes, and even collections — as long as you use indirection like Box
or &dyn Trait
. Why do we need indirection for trait objects?
Trait objects like dyn Trait
are unsized types — the compiler doesn’t know their size at compile time. That means:
But if you wrap them in something that has a known size — like a Box
, &
, or Rc
— you’re now dealing with a pointer, which does have a known size.
You can’t store them directly in structs, variables, or collections that require a known, fixed size.
Example: Trait object as struct field
struct Logger {
sink: Box<dyn std::io::Write>,
}
impl Logger {
fn log(&mut self, msg: &str) {
writeln!(self.sink, "{}", msg).unwrap();
}
}
This allows Logger
to log to a file, stdout, or any writer — all at runtime.
Example: collection of different types
trait Plugin {
fn name(&self) -> &str;
fn run(&self);
}
struct PluginA;
impl Plugin for PluginA {
fn name(&self) -> &str { "Plugin A" }
fn run(&self) { println!("Running A"); }
}
struct PluginB;
impl Plugin for PluginB {
fn name(&self) -> &str { "Plugin B" }
fn run(&self) { println!("Running B"); }
}
fn run_all(plugins: Vec<Box<dyn Plugin>>) {
for plugin in plugins {
println!("Plugin: {}", plugin.name());
plugin.run();
}
}
let plugins: Vec<Box<dyn Plugin>> = vec![Box::new(PluginA), Box::new(PluginB)];
run_all(plugins);
Now you’re working with multiple concrete types behind the same trait — a classic use case for dynamic dispatch.
Limitations of Trait Objects
Trait objects are powerful — but they come with trade-offs.
You can’t:
- Use generic methods in trait objects
- Return
Self
from trait methods - Call associated functions (no receiver like
self
) - Use trait objects for traits that require
Sized
You can’t call associated functions (those without a self
parameter) on trait objects, because the compiler doesn’t know which implementation to invoke. Trait objects require methods to use self
, &self
, or &mut self
.
These features require compile-time type knowledge — but trait objects erase the type.
Trait Objects vs Generics (impl Trait)
Feature | Trait Objects (dyn Trait ) | Generics / impl Trait |
---|---|---|
Dispatch | Dynamic (runtime) | Static (compile-time) |
Performance | Slight runtime cost | Zero-cost abstraction |
Flexibility | Return or store multiple types | One known concrete type |
Can be used in collections? | Yes, heterogeneous types | No, must be same concrete type |
Requires object-safe trait? | Yes | No |
Can use Self / generics? | No | Yes |
When to Use Trait Objects
Use trait objects when:
- You need runtime polymorphism
- You want to store different types behind the same interface
- You’re designing plugin systems, event handlers, or behavior graphs
- You need flexible APIs over performance-critical paths
Avoid them when:
- Performance is key (prefer
impl Trait
) - You don’t need the indirection
- You can solve the problem with static dispatch
Trait objects let you:
- Erase type information
- Work with values through a shared interface
- Call methods dynamically at runtime via a vtable
They’re essential for dynamic, extensible systems — especially when concrete types vary or are determined at runtime.
While they come with restrictions and a small performance cost, they give you an incredibly powerful tool for flexible design in Rust.
Trait objects can be difficult to understand, as they involve more advanced concepts, but with practice they become more clear and you will see their value and utility.
Coming up next: we’ll dive into supertraits, which allow traits to build on other traits — and help organize your trait hierarchies cleanly.
Super traits
In Rust, super traits allow you to require that a type implementing one trait must also implement another. This creates a form of trait inheritance—without the downsides of class-based inheritance. Super traits are particularly useful when you want to extend functionality while ensuring a common behavioral contract across types.
Let’s look into:
- What super traits are and why they’re useful
- How to define and implement them
- Real-world-inspired example:
Display
as a super trait - Using super traits with generics and trait bounds
What Are Super Traits?
A super trait is a trait that another trait depends on. When you define a trait as a super trait of another, you’re stating that any type implementing the child trait must also implement the super trait(s).
This allows the child trait to reuse functionality defined in the super trait without additional constraints.
use std::fmt::Display;
trait Printable: Display {
fn print(&self) {
println!("{}", self);
}
}
In this example, Printable
is a child trait of Display
, making Display
a super trait. That means any type implementing Printable
must implement Display
as well.
Defining and Using Super Traits
Let’s walk through how to use super traits in practice.
Step 1: Define a Super Trait
trait Identifiable {
fn id(&self) -> u32;
}
Step 2: Define a Trait That Depends on It
trait Loggable: Identifiable {
fn log(&self) {
println!("Logging item with ID: {}", self.id());
}
}
The Loggable
trait now requires the Identifiable
trait, allowing it to safely call .id()
within its methods.
Step 3: Implement for a Struct
struct User {
id: u32,
name: String,
}
impl Identifiable for User {
fn id(&self) -> u32 {
self.id
}
}
impl Loggable for User {}
Step 4: Use It!
fn main() {
let user = User {
id: 101,
name: "Greg".into(),
};
user.log(); // Logging item with ID: 101
}
Note that when we implement Loggable for struct User, we have an empty implementation block. Because we make use of the default implementation of the log() method it’s enough just to have:
impl Loggable for User {}
Real-World Example: Display + Printable
Rust’s Display
trait is often used as a super trait, especially when formatting is needed.
use std::fmt::Display;
trait PrettyPrint: Display {
fn pretty_print(&self) {
println!("--- {} ---", self);
}
}
struct Report {
title: String,
}
impl Display for Report {
fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result {
write!(f, "{}", self.title)
}
}
impl PrettyPrint for Report {}
fn main() {
let report = Report {
title: "Quarterly Earnings".into(),
};
report.pretty_print(); // Output: --- Quarterly Earnings ---
}
This is a clean way to extend formatting logic while guaranteeing that the base formatting (Display
) is available.
Using Super Traits with Generics
When working with generic functions or structs, super traits let you constrain type parameters elegantly.
fn print_twice<T: Printable>(item: &T) {
item.print();
item.print();
}
Under the hood, this ensures that T: Display
too, because Printable
requires it. You don’t have to specify both.
Here’s another example: imagine a logging utility that requires both Debug
and Identifiable
.
trait Debuggable: std::fmt::Debug + Identifiable {
fn debug_log(&self) {
println!("Debug: {:?}, ID: {}", self, self.id());
}
}
Super traits scale nicely when you want composability and type safety.
You’ll often see super traits used in the Rust standard library — for example, the Iterator
trait sometimes includes Sized
as a super trait when certain methods (like .collect()
) are provided only for sized iterators. Super traits are a powerful way to layer and extend behavior safely.
Why Super Traits Matter
- Powerful trait bounds: Super traits make your APIs more expressive while ensuring correct usage.
- Composable abstractions: You can break large behaviors into smaller traits and combine them as needed.
- Avoid repetition: Default methods in child traits can use super trait methods directly.
Advanced trait features
Rust’s trait system is one of its most powerful features, offering capabilities far beyond what most mainstream languages provide. Once you’re comfortable with basic traits and trait bounds, it’s time to dive into some advanced features that make Rust’s trait system truly shine.
In this section, we’ll cover:
- Blanket Implementations
- Conditional Methods
- Coherence and the Orphan Rule
Blanket Implementations
Blanket implementations allow you to provide trait implementations for any type that satisfies certain bounds. This is a powerful way to write generic and reusable code.
Example: Implementing a Custom Trait for All Display
Types
use std::fmt::Display;
trait Printable {
fn print(&self);
}
// Blanket implementation: any T that implements Display now also implements Printable
impl<T: Display> Printable for T {
fn print(&self) {
println!("{}", self);
}
}
fn main() {
let x = 42;
let s = "Hello, traits!";
x.print(); // Works because i32 implements Display
s.print(); // Works because &str implements Display
}
Rust’s coherence rules ensure safe and non-conflicting trait implementations. In particular, the orphan rule says you can only use blanket implementations if either the trait or the type is defined in your crate. This prevents multiple crates from implementing the same trait for the same type differently — a potential source of bugs in other languages.
With just one impl
, we enabled all Display
types to gain a new method, print
. This pattern is used heavily in the standard library and crates like serde
.
Note: Because of Rust’s coherence rules (more on that later), blanket impls are only allowed if either the trait or the type is local to your crate.
Conditional Methods with Trait Bounds
Sometimes, you want to make a method available only if the type also implements another trait. Rust lets you do this using trait bounds on impl
blocks or even specific methods.
Example: Conditionally Implementing a Method
struct Container<T> {
value: T,
}
impl<T> Container<T> {
fn new(value: T) -> Self {
Container { value }
}
// Always available
fn get(&self) -> &T {
&self.value
}
}
// This method is only available if T: Display
impl<T: Display> Container<T> {
fn display(&self) {
println!("The value is: {}", self.value);
}
}
fn main() {
let a = Container::new(123);
a.display(); // i32 implements Display, so this works
let b = Container::new(vec![1, 2, 3]);
// b.display(); // Compile error: Vec<i32> does not implement Display
}
This approach is especially useful in generic types and libraries, where you want to provide richer functionality only when appropriate.
Coherence and the Orphan Rule
Rust enforces strict rules around trait implementations to preserve type safety and prevent conflicting impls. These are known as the coherence rules, and the most important one is the orphan rule.
The Orphan Rule
You can only implement a trait for a type if at least one of the trait or the type is defined in your crate.
You cannot implement foreign traits for foreign types.
A foreign trait is a trait defined in another crate. A foreign type is a type defined in another crate.
Example: Legal and Illegal Implementations
Suppose you want to implement Display
(a foreign trait) for Vec<T>
(a foreign type). You can’t:
use std::fmt;
impl<T> fmt::Display for Vec<T> {
// ERROR: both Vec and Display are defined outside your crate
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
write!(f, "Custom display for vec")
}
}
But if you define your own trait or your own type, you’re free to implement them:
// Your own trait
trait MyTrait {
fn hello(&self);
}
// Legal: You own the trait
impl<T> MyTrait for Vec<T> {
fn hello(&self) {
println!("Hello from MyTrait!");
}
}
// Your own type
struct MyType;
impl fmt::Display for MyType {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
write!(f, "This is MyType!")
}
}
Why the Rule Exists
The orphan rule prevents conflicting implementations across crates. Without it, two crates could implement the same trait for the same type differently, leading to ambiguity or inconsistency.
Rust’s trait system is more than just interfaces—it’s a powerful abstraction mechanism with fine-grained control. Here’s a quick recap of the advanced features we covered:
- Blanket impls let you implement a trait for many types at once, based on trait bounds.
- Conditional methods allow you to restrict methods to types that meet specific trait constraints.
- Coherence and the orphan rule keep the trait system predictable and safe by preventing conflicting impls.
Understanding and leveraging these advanced features allows you to write highly reusable, flexible, and safe Rust code—especially in library and framework development.
Traits play an important role in Rust. This post discussed traits in detail, diving into a wide range of trait topics you’ll want to be familiar with as you program in Rust.
Thanks for reading, and for letting ByteMagma be part of your journey to Rust mastery!
Leave a Reply