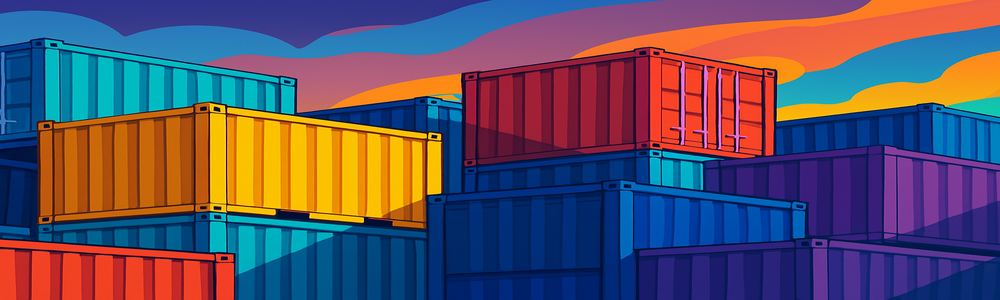
Introduction
Collections are the building blocks of real-world data manipulation. You might be aggregating user input, tracking scores in a game, or managing configuration options. Collections make it possible to store and organize multiple values efficiently. In Rust, collections are more than just containers—they ensure memory and thread safety, and performance without the need for a garbage collector.
Rust doesn’t rely on runtime memory management. It enforces strict rules at compile time, helping you avoid common bugs like null references, dangling pointers, and data races. Rust’s collection types integrate with its powerful ownership and borrowing system, making them safe and efficient by default.
In this post, we’ll explore the most commonly used collection types in Rust:
Vec<T>
: a growable, heap-allocated list, perfect for storing a sequence of values.String
: a UTF-8 encoded, heap-allocated text type that builds onVec<u8>
.HashMap<K, V>
: a key-value store that’s ideal for fast lookups and flexible associations.
We’ll also briefly touch on other collection types available in the standard library, such as HashSet
, BTreeMap
, and LinkedList
, and explain when they might be appropriate.
By the end, you’ll not only understand how to use these collections but also how Rust’s design choices help you write fast, reliable, and expressive code.
Vec<T> The Rust Workhorse
The first Rust collection type we’ll look at is the Vec<T>
type, commonly known as vector. The Rust vector is a generic type, meaning we specify the type of elements the vector will store using the generic type parameter <T>
. For example if we want to store i32 data in a vector, its type will be Vec<i32>
. Generics in Rust will be covered in detail in a future post, but in this post we’ll see several examples of generics in action.
Similar to Rust arrays, the elements in a Rust vector must be of the same type.
Unlike arrays, which are fixed-size and allocated on the stack, Vec<T>
is a growable, heap-allocated collection. The vector struct itself is stored on the stack and contains a pointer to the heap buffer, the current length, and the capacity. The actual elements live on the heap. Rust vectors manage their memory through ownership, and cleanup is handled automatically when the vector goes out of scope.
Vectors have a capacity()
method, and Rust often over-allocates space to avoid reallocating every time push()
is called.
Let’s create a new Rust package
and write some code. CD into the directory where you store Rust packages
and execute this command:
cargo new collections
Now CD into the collections directory and open that directory in VS Code or your favorite IDE.
Note: using VS Code will make it easier to follow along with our posts. And installing the Rust Analyzer extension is also beneficial.
Also, opening the directory containing your Rust package
allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the collections directory in VS Code.
Open the file src/main.rs and replace its contents entirely with this code, then execute cargo run:
fn main() {
let mut todos = Vec::new();
todos.push("Buy milk");
todos.push("Call Alice");
todos.push("Write blog post");
for (i, task) in todos.iter().enumerate() {
println!("{}: {}", i + 1, task);
}
}
Output:
1: Buy milk
2: Call Alice
3: Write blog post
First we define a mutable variable named todos and initialize it to an empty vector with the Vec builtin new() associated function. All variables are immutable (cannot change) by default in Rust so we add the mut
keyword to make our variable mutable, as we want to add elements to it.
If you need a refresher on mutability you can refer to this ByteMagma post: Mutability, Copying, and Why Some Things Just Vanish. Haven’t learned about Rust functions? Refer to this ByteMagma post: Functions in Rust – The Building Blocks of Reusable Code.
Then we call the vector push() method to add several string literals (string slices). We then iterate over the vector to print out the index of the element in the vector and the element at each index.
Our for
loop illustrates one way to iterate over a vector. Calling the iter()
method on a vector creates an iterator over references to the elements in the vector. We want references rather than the actual data because by default Rust moves the elements into the for loop, making the vector invalid and unusable. Getting references with iter()
allows our code to instead borrow the elements.
For more on references, ownership, moving and borrowing, this ByteMagma post may be useful: Ownership, Moving, and Borrowing in Rust.
The enumerate()
method allows us to also get the index of the elements in the vector. It turns a plain iterator into one that yields (index, item) tuples.
The Vec type in Rust has many useful methods. Here are a few of the most commonly used methods.
push() | pop() | len() | is_empty() | get(index) |
remove(index) | insert(index, value) | clear() | contains(&value) | iter() |
iter_mut() | into_iter() | sort() | reverse() | dedup() |
Note that we didn’t specify the type of elements stored in this vector:
let mut todos = Vec::new();
We are adding string slices to the vector so the compiler can infer the type of the elements. We could have specified the element type explicitly:
let mut todos = Vec::<&str>::new();
or
let mut todos: Vec<&str> = Vec::new();
Summing Scores
In this example we have a vector of grades and we sum them with the iterator sum() method:
let grades = vec![85, 92, 78, 90];
let sum: i32 = grades.iter().sum();
let average = sum as f32 / grades.len() as f32;
We create the grades vector using the vec![]
macro, a convenience macro for creating a vector with an initial set of elements. Then we sum the grades and get the average grade.
Note how we cast sum and grades.len() to f32
because the division could result in a floating point number, and sum and the length of the vector are integers.
Filtering and Collecting
This next example allows us to filter emails to only those that include the @ symbol.
let emails = vec!["a@example.com", "invalid", "b@example.com"];
let valid: Vec<&str> =
emails
.into_iter()
.filter(|e| e.contains('@'))
.collect();
This is a good example of method chaining, where we call a method and then call another method on the return value.
We have a vector of emails but some might have “invalid” instead of a valid email. We call several methods on our emails vector, and assign the result to a new vector named valid.
into_iter()
consumes the emails collection and turns it into an iterator over its elements. Earlier we saw using iter()
to create an iterator that borrows the vector elements. In this example we use into_iter()
which doesn’t borrow the elements, it moves the elements, consuming them. After this code runs, the emails vector will be invalid and unusable.
We chain a call to the iterator filter()
method. It takes a closure as a parameter. Closures will be covered in detail in a future post, for now just understand that a closure is an anonymous function (function without a name). The | e | represents each element of the email vector being passed into this closure. The body of the closure checks if the email contains the “@” symbol.
Finally we chain a call to the collect()
method, which creates a new collection from the iterator. Because the valid vector type is Vec<&str>
, collect()
will produce a new Vec<&str>
collection.
Note that we could also use the retains()
method to perform filtering.
let mut nums = vec![1, 2, 3, 4, 5];
nums.retain(|&x| x % 2 == 0);
// nums is now [2, 4]
String and &str – Strings and String Slices
String
is the Rust data type for a group of characters. &str
is a string slice, representing part of a String.
String and &str are not collections, but they behave like collections. They’re not part of std::collections
, but both String
and &str
implement iterator traits, allowing collection-like behaviors such as mapping, filtering, and collecting.
String behaves like a collection of characters. It owns a sequence of UTF-8 bytes, and you can iterate over its characters or bytes.
let mut s = String::from("hello");
for c in s.chars() {
println!("{}", c); // prints h e l l o
}
&str
is a view into a collection of bytes/chars. Often string slices are created from string literals: “world”
let s = "world"; // type: &str
for b in s.bytes() {
println!("{}", b); // prints byte values
}
This next example shows creating a String using its from() method, and then chaining methods on it to create a String that has only letters, no numbers or special characters.
let raw = String::from("User123!@#");
let letters_only: String = raw.chars().filter(|c| c.is_alphabetic()).collect();
println!("{}", letters_only); // Output: "User"
Next we check to see if a &str contains the text “error”.
let input = "error: file not found";
if input.contains("error") {
println!("Log contains an error");
}
We can also chain methods on a &str, in this case getting the characters of a &str, then calling map() which operates on each character. Here we convert the character to uppercase.
let word = "Greg";
let uppercased: String = word.chars().map(|c| c.to_ascii_uppercase()).collect();
println!("{}", uppercased); // Output: "GREG"
We can also split a string slice and specify the delimiter. Here we use a comma as the delimiter to split a string slice into words.
let csv = "apples,bananas,grapes";
let fruits: Vec<&str> = csv.split(',').collect();
for fruit in fruits {
println!("{}", fruit);
}
apples
bananas
grapes
We can also add to a string, similar to adding to a vector:
let mut story = String::from("Once upon a time");
story.push_str(", there was a dev who learned Rust.");
println!("{}", story);
Once upon a time, there was a dev who learned Rust.
We can convert a string slice into a collection:
let name: &str = "Greg";
let chars: Vec<char> = name.chars().collect();
println!("{:?}", chars); // Output: ['G', 'r', 'e', 'g']
So even though String
and &str
aren’t officially collection types in std::collections
, they behave just like collections of characters (or bytes). And Rust gives you powerful iterators and adapters to treat them that way—cleanly and efficiently.
HashMap: Organizing Data with Key-Value Pairs
HashMap
is a Rust collection storing data as key-value pairs. HashMap
enables fast retrieval of a value by its corresponding key, using a hashing algorithm under the hood.
- Keys must be unique.
- Both keys and values can be any type that implements the
Eq
andHash
traits (most primitives and strings do). - HashMap is part of Rust’s standard library under
std::collections::HashMap
.
HashMap
s are great for situations where you want:
- Fast lookups (e.g. config settings, user sessions, caching).
- Counting or grouping items (e.g. word frequencies).
- Storing relationships between keys and values (e.g. product ID to product name).
Determine Word Frequency
Let’s look at an example of using a HashMap to determine word frequency in text.
use std::collections::HashMap;
fn main() {
let text = "hello world wonderful world";
let mut word_count = HashMap::new();
for word in text.split_whitespace() {
// Increment count or insert with 1
let count = word_count.entry(word).or_insert(0);
*count += 1;
}
// Display the word frequencies
for (word, count) in &word_count {
println!("'{}': {}", word, count);
}
}
Output:
'world': 2
'wonderful': 1
'hello': 1
We create a new, initially empty HashMap with its new() associated function.
We then split the text with the builtin split_whitespace()
method that parses text and breaks it up using whitespace (spaces, tabs, etc.) as the delimiter.
These two lines are interesting and require a bit of explanation:
let count = word_count.entry(word).or_insert(0);
*count += 1;
word_count.entry(word)
attempts to find an element in our word_count HashMap for the key that is the current word. We chain .or_insert(0)
and the result is:
- if the word doesn’t exist in our HashMap, we insert it and set its value to 0, and we set count to that element
- if the word does exist, we simply set count to that element
Then we increment the count variable. We do this with the dereference operator because count is actually a reference to the HashMap element.
Note that the following code also uses the and_modify() method as a more concise version of the above code.
word_count.entry(word).and_modify(|c| *c += 1).or_insert(1);
When our first for loop has completed, our word_count HashMap
keys are the words in the text (no duplicates), and the value for each key (word) is the frequency the word occurs in the text.
Tracking Product Inventory
Our next example is for tracking product inventory. When an item is sold we update the inventory counts.
use std::collections::HashMap;
fn main() {
let mut inventory = HashMap::new();
// Add products
inventory.insert("Apple", 50);
inventory.insert("Banana", 30);
inventory.insert("Orange", 20);
// A sale is made
let sold = "Apple";
if let Some(stock) = inventory.get_mut(sold) {
*stock -= 1;
println!("Sold one {}. Remaining: {}", sold, stock);
}
// Display full inventory
for (product, qty) in &inventory {
println!("{}: {}", product, qty);
}
}
Output:
Sold one Apple. Remaining: 49
Apple: 49
Banana: 30
Orange: 20
We create a new Hashmap
called inventory and insert data for several products. The keys are the product names (fruits) and the values are the inventory count for that fruit.
We use the get_mut() method to get a mutable reference the HashMap element whose key is Apple, and if we find it we decrease the quantity for that fruit and print a message indicating a sale was made.
Finally we have another for loop where we print out all the product inventory data.
We use a mutable reference to the HashMap element because we don’t want to take ownership (move) of the data, we just want to borrow it so we can update the inventory count.
Other Rust Collections
Here’s a brief overview of some other commonly used collections in Rust beyond Vec
and HashMap
:
VecDeque<T>
- A double-ended queue implemented with a growable ring buffer.
- Supports efficient push/pop operations from both front and back (
push_front
,pop_back
, etc.). - Useful when you need queue or deque behavior with performance similar to
Vec
.
LinkedList<T>
- A doubly-linked list.
- Efficient insertion and removal at arbitrary positions.
- Less cache-friendly and usually slower than
Vec
orVecDeque
for most use cases. - Rarely needed unless you have specific needs like frequent mid-list insertions.
HashSet<T>
- A collection of unique values, backed by a
HashMap<T, ()>
. - Fast membership testing, insertion, and removal.
- Great when you want to track presence/absence of items without associated values.
BTreeMap<K, V>
- A sorted key-value map, implemented as a B-tree.
- Keys are ordered, so you can iterate in sorted order.
- Slower than
HashMap
for basic lookups, but offers range queries and ordered iteration.
In this post we examined the most common Rust collections. We also looked at String and string slices (&str) which are not collections but behave very similar. We saw a number of code examples illustrating how versatile Rust collections are, and how useful they are for storing and manipulating data.
Thank you so much for allowing ByteMagma to be a part of your Rust mastery journey!
Leave a Reply