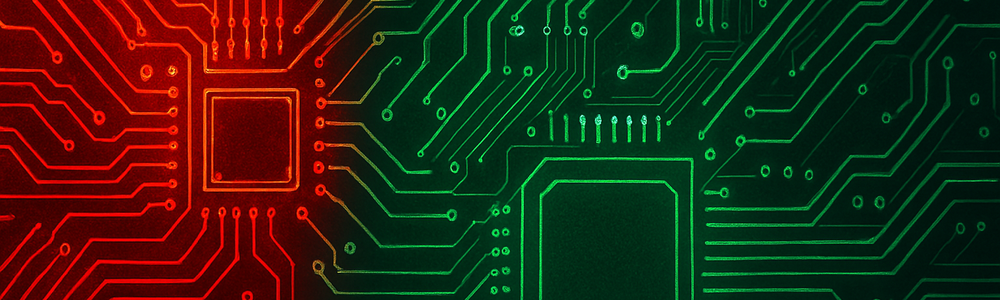
Every programming language has ways to deal with unexpected situations. It could be a missing file, a division by zero error, or some other problem. Rust is known for its safety and performance, and offers a unique and powerful approach to error handling through panics, and the Result
and Option
enums.
In this post we’ll learn about each of these concepts and more, and we’ll see concrete examples that demonstrate how to deal with errors in Rust. If you’re new to Rust enums or the Option
enum, check out this ByteMagma blog post: Rust Enums: Close Cousins of Structs
Introduction
Rust takes a deliberate and structured approach to error handling, distinguishing between recoverable and unrecoverable errors. Recoverable errors—such as a file not being found or a network timeout—are expected in real-world applications and are handled using the Result
type. These allow the program to respond gracefully and continue running.
Unrecoverable errors, on the other hand, represent bugs or logic errors in the program—like indexing past the end of an array. These trigger a panic!
and typically crash the program, because there’s no sensible way to continue execution.
This clear separation reflects Rust’s philosophy: make error handling explicit and safe, avoiding silent failures and encouraging developers to think through how things can go wrong—without sacrificing performance or reliability.
Panic – When Things Go Very Wrong
Let’s start with unrecoverable errors. Panic is Rust’s mechanism when things go so wrong that the program cannot be expected to recover, and the program must terminate.
When a panic occurs, the program immediately stops execution, unwinds the stack, and cleans up resources before terminating. By default, Rust unwinds the stack on panic to run destructors (i.e., Drop
implementations), but in release builds you can configure it to abort immediately using panic = "abort"
in Cargo.toml.
Let’s create a new Rust package
and write some code. CD into the directory where you store Rust packages
and execute this command:
cargo new error_handling
Now CD into the error_handling directory and open that directory in VS Code or your favorite IDE.
Note: using VS Code will make it easier to follow along with our posts. And installing the Rust Analyzer extension is also beneficial.
Also, opening the directory containing your Rust package
allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the error_handling directory in VS Code.
Open the file src/main.rs and replace its contents entirely with this code:
fn main() {
println!("Before panic...");
panic!("Something went terribly wrong!");
println!("This line will never be reached.");
}
This code shows the easiest way to trigger a panic in Rust, with the panic!()
macro. We first print a message then we call the Rust panic!()
macro, passing a message to display in the shell window. The second message will never be printed because on panic the program terminates.
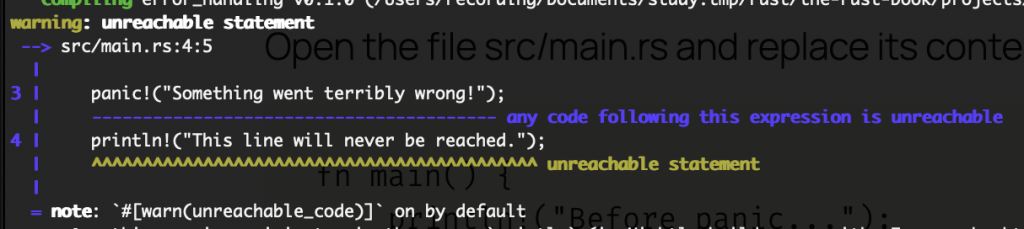
Let’s look at several scenarios where your code might trigger a panic. You can comment out the current code in main.rs with a block comment /* code */ or simply replace it with these code snippets, then execute cargo run to see the result.
Out of Bounds Access
The following code panics because we try to access an element in the vector at index 5. The vector only has three elements, so index 5 is not valid.
fn main() {
let numbers = vec![1, 2, 3];
println!("{}", numbers[5]); // This will panic!
}

There are several ways to improve your code to avoid a panic in this situation. Instead of accessing the vector element directly by index you could use the vector get()
method. get()
returns an Option<T>
and numbers.get(5) returns the Option None
variant because index 5 is out of bounds.
fn main() {
let numbers = vec![1, 2, 3];
match numbers.get(5) {
Some(value) => println!("Value: {}", value),
None => println!("Index out of bounds!"),
}
}
You could also address this situation with an if-let
construct:
fn main() {
let numbers = vec![1, 2, 3];
if let Some(value) = numbers.get(5) {
println!("Value: {}", value);
} else {
println!("Index out of bounds!");
}
}
This is basically an if-else
construct with the condition that numbers.get(5) produces a Some(value)
.
Unwrapping a None or Err
In this code, we call the unwrap()
method on an Option
enum None
variant instance. The Option
enum has two variants, Some()
and None
. The unwrap()
method is used to get the actual value held by an Option Some()
variant. It will panic if called on a None
, so it’s best used only when you’re certain the Option
is Some
.
fn main() {
let option: Option<i32> = None;
// Panics with "called `Option::unwrap()` on a `None` value"
println!("{}", option.unwrap());
}

We can also use an if-let
construct to handle this situation:
fn main() {
let option: Option<i32> = None;
if let Some(value) = option {
println!("Value: {}", value);
} else {
println!("No value present");
}
}
If you prefer to handle each case more explicitly you can use a match
construct:
fn main() {
let option: Option<i32> = None;
match option {
Some(value) => println!("Value: {}", value),
None => println!("No value present"),
}
}
An even more concise way would be to use the Option
unwrap_or()
method to provide a fallback default value.
fn main() {
let option: Option<i32> = None;
println!("Value: {}", option.unwrap_or(0)); // prints 0 if None
}
There are many ways to do things in Rust. Here are two more possibilities.
.unwrap_or_else()
fn main() {
let option: Option<i32> = None;
println!("Value: {}", option.unwrap_or_else(|| {
// Some expensive computation or logging
println!("Generating default...");
42
}));
}
.map_or()
fn main() {
let option: Option<i32> = None;
let doubled = option.map_or(0, |v| v * 2);
println!("Doubled value: {}", doubled);
}
Integer Overflow (in debug mode)
Here we define a variable x of type u8
and initialize it to a value of 255. The maximum value a u8
can hold is 255, so when we increment x by 1 the program panics (in debug mode).
fn main() {
let mut x: u8 = 255;
// Panics in debug mode, but wraps around in release mode.
x += 1;
}
Rust has two primary compilation modes: debug mode and release mode. These modes affect how your code is compiled and optimized.
- Debug mode is the default when you run
cargo build
orcargo run
. It compiles quickly and includes debug symbols, making it ideal for development and testing. However, it doesn’t perform many optimizations, so the resulting executable is slower and larger. - Release mode is enabled with
cargo build --release
. It enables powerful compiler optimizations that make your program run significantly faster and use less memory. This is the mode you’d use when building software for production or benchmarking.
These modes also affect behavior around things like overflow checks. For example, in debug mode, integer overflows cause a panic. But in release mode, they wrap around silently (i.e., they use modular arithmetic) unless you explicitly check for overflows.
So in debug mode you get a panic, alerting you to improve your code to avoid the problem.

To write panic-free and intentional code, here are several ways to safely handle integer overflow in Rust.
.wrapping_add()
– will add 1 to x, but if it would cause an overflow it instead wraps to 0.
fn main() {
let mut x: u8 = 255;
x = x.wrapping_add(1); // x becomes 0
println!("{}", x);
}
Note that wrapping refers to going to the first possible value for a type, so for u8
, which can hold values from 0 – 255, wrapping results in a value of zero.
.checked_add()
– returns None on overflow
fn main() {
let mut x: u8 = 255;
match x.checked_add(1) {
Some(result) => println!("Result: {}", result),
None => println!("Overflow detected!"),
}
}
.saturating_add()
caps the value at the max for the type (255 for u8)
fn main() {
let mut x: u8 = 255;
x = x.saturating_add(1); // x stays at 255
println!("{}", x);
}
.overflowing_add()
returns a tuple (result, did_overflow)
fn main() {
let mut x: u8 = 255;
let (result, overflowed) = x.overflowing_add(1);
println!("Result: {}, Overflow occurred: {}", result, overflowed);
}
This is a good example of how Rust provides multiple ways to handle potential error situations in code. The solution you choose often depends on the coding scenario, and also on your preferences and which methods you are most comfortable with.
When to Panic
Panic should be used only when something unexpected and unrecoverable happens—such as a bug or invalid state that you cannot handle. For errors that you expect and can recover from, prefer Result
or Option
.
The Result Enum: Recoverable Errors with Success or Failure
Now that we’ve looked at how to handle errors that can lead to program termination on panic
if not handled properly, let’s learn more about the Result
enum.
The Rust Result
enum is used for operations that might succeed or fail. Here is the definition:
enum Result<T, E> {
Ok(T), // Represents success and contains a value
Err(E), // Represents an error and contains an error value
}
The Rust Result
enum is a generic that specifies the success value type T
and the error type E
. A future post will discuss generics in detail, in this post we’ll see examples of using Result and specifying the T and E generic types.
We’ll see examples of code that checks to see if an operation produced a Result
enum Ok()
variant holding a value, or if instead the operation produced a Result
enum Err()
variant with an error.
Reading a File
Let’s say we’re trying to read the contents of a file. This is an operation that can fail if the file is missing or if there are insufficient permissions.
use std::fs::File;
use std::io::Read;
fn main() {
match read_file("data.txt") {
Ok(content) => println!("File content: {}", content),
Err(e) => println!("Error reading file: {}", e),
}
}
fn read_file(filename: &str) -> Result<String, std::io::Error> {
let mut file = File::open(filename)?; // Propagate errors with ?
let mut content = String::new();
file.read_to_string(&mut content)?;
Ok(content)
}
In this code we define a function read_file() that takes a single parameter, the name of the file to read from. The function is defined to return a Result
enum. We’ve specified the success and failure generic types as String and std::io::Error:
Result<String, std::io::Error>
So if this file read operation succeeds this function reads the contents of the file and returns it as a String wrapped in the Result Ok()
variant. On failure the function returns an error wrapped in the Result Err()
variant, specifically the std::io::Error from the Rust standard library.
Notice the question mark ( ? ) in this line:
let mut file = File::open(filename)?;
The ?
is the Rust “try operator”. It’s syntactic sugar used for error propagation when working with functions that return a Result<T, E>
or an Option<T>
. Syntactic sugar means code that is simple and concise, but behind the scenes there is more complex code being executed.
In our code, since File::open()
returns a Result
, the ?
operator helps propagate the error cleanly. The ?
try operator automatically “unwraps” the Result
. On success it gives the actual value from the Result
. On failure it immediately returns the Err
and doesn’t continue with the rest of the code in the read_file() function. Similarly, the ?
operator can be used with Option<T>
as well—it returns early with None
if the value is None
.
Understanding Unwrap
When using Result<T, E>
, the success value will be “wrapped” in the Result Ok()
enum variant, and on failure the error will be “wrapped” in the Result Err()
variant. Rust does this for safety, clarity and control. It forces you to explicitly code to handle both the Ok()
and the Err()
cases. So to get at the actual value of the operation performed, you need to “unwrap” the value or error.
If our code opens the file and gets a file handle, it reads from the file and returns it as a String in the Result Ok()
variant. Back in main() we match
on the return value from the read_file() function, either printing the file contents or printing the error. If you want to review the Rust match construct you can check out this ByteMagma post: Making Decisions in Rust: Conditionals and Match Explained
Let’s look at another example using the Result enum. In this code we define a function divide() that takes two i32
parameters, and returns a Result<i32, String>
.
fn divide(a: i32, b: i32) -> Result<i32, String> {
if b == 0 {
Err(String::from("Cannot divide by zero"))
} else {
Ok(a / b)
}
}
fn main() {
let result = divide(10, 0);
match result {
Ok(value) => println!("Result: {}", value),
Err(e) => println!("Error: {}", e),
}
}
The function immediately checks if parameter b is zero and if so, the function returns the Err()
variant of Result
. If parameter b is not zero then the function returns a divided by b in the Ok()
variant.
Notice that we don’t use the return
keyword to explicitly return a value from the function. In Rust, if we don’t explicitly return a value, then the last expression evaluated will be returned. Because our two if-else branches have expressions, not statements ending in a semicolon, they will be the return value.
Once again, in main() we match on the return value of the divide() function, and either print the result of division or the error.
When to Use Result
- When the error is expected and recoverable, such as reading files, network requests, or parsing user input.
- When the caller needs to handle the error differently based on the context.
The Option Enum: Handling the Absence of a Value
Now that we’ve seen the Result
enum to handle success or failure, let’s look at the Option<T>
enum to handle the presence or absence of a value.
enum Option<T> {
Some(T), // Contains a value
None, // No value present
}
Similar to the Result<T, E>
enum, Option<T>
is also a generic enum. The T generic parameter is the value that will be wrapped in the Some()
variant. So when using Option
, you either have a value wrapped in an instance of the Some()
variant or you have an instance of the None
variant.
By design Rust does not have the null value commonly found in other languages. The null value in those languages can give rise to unexpected behavior and other unsafe conditions. Rust offers strong safety and thus uses the Option None
variant to express the absence of a value.
Let’s look at some examples.
This first example defines a User struct and a function to find a user within an array of users. The function returns a reference to the found user. Note: <'a>
declares a lifetime parameter, and 'a
is used to annotate that references to users and the return value share the same lifetime. This ensures memory safety, preventing dangling references.
A future post will explore Rust lifetimes in detail, for now just understand that lifetime parameters contribute to Rust’s memory safety by explicitly specifying how long references are valid.
struct User {
name: String,
age: u32,
}
fn find_user<'a>(name: &'a str, users: &'a [User]) -> Option<&'a User> {
for user in users {
if user.name == name {
return Some(user);
}
}
None
}
fn main() {
let users = vec![
User { name: String::from("Alice"), age: 30 },
User { name: String::from("Bob"), age: 25 },
];
match find_user("Alice", &users) {
Some(user) => println!("Found user: {} (Age: {})", user.name, user.age),
None => println!("User not found."),
}
}
The find_user() function iterates over the users array. If it finds a user with the provided name, it returns the user wrapped in the Some()
variant of the Option
enum. If the “for” loop doesn’t find a user and returns, then the None
variant is returned implicitly, because as mentioned above it is the last expression evaluated in the function. It is an expression because it does not end in a semicolon, otherwise it would be a statement.
Back in main() we define a vector of users, and then match
on the return value of the find_user() function. The return value will either be a user wrapped in an Option
Some()
variant or a None
variant. The match arms check this and print appropriate messages.
In this Rust code, the match
arm automatically unwraps the Some
variant, binding the inner value to user
, which we can use in our println!().
Combining Result and Option
Sometimes, you’ll need to combine Result
and Option
when dealing with operations that may fail and return either success, failure, or no value.
In this code we define a function get_config_file() that should return a File handle. Or we could get a file not found error, or an error due to insufficient permissions. But this code relies on the presence of an environment variable CONFIG_PATH being set on the system where this program is executed. If that environment variable is not set, then None would be returned.
use std::fs::File;
use std::env;
fn get_config_file() -> Option<Result<File, std::io::Error>> {
// Try to get CONFIG_PATH from the environment
let config_path = env::var("CONFIG_PATH").ok();
match config_path {
Some(path) => Some(File::open(path)), // Could be Ok or Err
None => None, // No config path specified
}
}
fn main() {
match get_config_file() {
Some(Ok(file)) => println!("Config file opened successfully: {:?}", file),
Some(Err(e)) => println!("Failed to open config file: {}", e),
None => println!("No config path provided in environment."),
}
}
The return type of this function is Option<Result<File, std::io::Error>>. You’ll see this kind of return type a lot in Rust code. The return type is the Option
enum, where the Some()
value will be a Result
enum. The Result
enum will have a File
handle for the Ok()
variant or a std::io::Error
for the Err()
variant.
If the CONFIG_PATH environment variable is set on the system then config_path will contain its value. We match for this because if the environment variable is not set then config_path will be None
.
env::var(“CONFIG_PATH”) will return an Ok(String)
with the environment variable value or Err(VarError)
. The .ok()
method converts a Result
into an Option
. Ok(value)
becomes Some(value)
and Err()
becomes None
. VarError is the error type returned from env::var when it fails to read an environment variable.
When to Use Option
- When a value might be missing or optional, such as querying a database or searching a list.
- To avoid using
null
pointers, which can lead to undefined behavior.
Best Practices for Error Handling in Rust
- Use
Result
for recoverable errors.- Provide detailed error messages to aid in debugging.
- Use
Option
when a value might be absent.- Avoid unwrapping unless absolutely certain.
- Use
panic!
only for unrecoverable errors.- Be cautious when writing libraries—avoid panicking unless the error is catastrophic.
Rust’s approach to error handling encourages developers to think critically about failure and success scenarios. By effectively using panic!
, Result
, and Option
, you can build robust applications that gracefully handle errors and maintain stability.
You’ll see Result and Option a lot in Rust code and you’ll see them returned from many Rust methods, often used together.
Thank you so much for visiting, and for including ByteMagma in your Rust mastery journey!
Leave a Reply