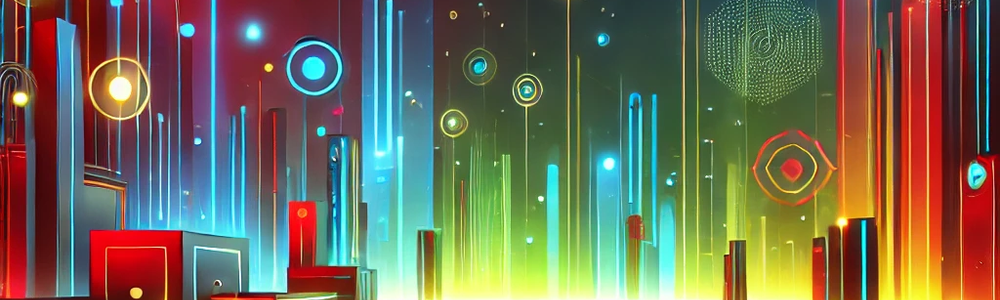
Enums in Rust are closely related to structs. They let you define a type by listing possible variants—and you can even attach data to those variants. That might sound a little mysterious, but in this post, we’ll break it down and show you how enums, like structs, are among the most powerful and commonly used features in Rust.
If you’re just getting started with Rust, these posts earlier in this series on Rust programming may be helpful:
Setting Up Your Rust Development Environment
Mutability, Copying, and Why Some Things Just Vanish
Functions in Rust – The Building Blocks of Reusable Code
Ownership, Moving, and Borrowing in Rust
Structs in Rust: Modeling Real-World Data
Introduction
Rust structs let you group related data fields together and define behavior using methods and associated functions.
Rust enums, on the other hand, let you define a type that can be one of several possible variants. For example, we can define an enum Shape with variants like Rectangle, Circle, and Triangle.
Just like structs, enums can have methods and associated functions—and in this post, we’ll look at examples of both.
We’ll also explore two powerful enums from Rust’s standard library: Option and Result. As you gain experience with Rust, you’ll find yourself using these two all the time.
Traffic Light Scenario
Let’s consider a scenario where we want to represent the possible values of a traffic light using an enum. A traffic light can be green, yellow, or red — exactly one of these three states at any given time — making them perfect candidates for enum variants.
We’ll design our enum so that each variant can hold a value: the number of seconds the light should remain active.
This allows our enum to be flexible enough for use at different intersections — some may require longer green lights to accommodate heavier traffic, while others may need extended yellow lights due to faster-moving vehicles.
Later in the post, we’ll introduce a struct that tracks the current state of the traffic light — whether it’s green, yellow, or red.
The struct will include methods and associated functions to control and display the state of the light over time.
Defining Enums in Rust
Let’s revisit our scenario and see why an enum is a good fit. A traffic light can be red, yellow, or green. Since these are the only valid states for a traffic light, we can enumerate all possible variants in our code.
At any given time, a traffic light is exactly one of these colors — it can’t be two at once. This makes it a perfect use case for a Rust enum, which can only hold one variant at a time. Even though the values are different (red, yellow, green), they all represent the same kind of thing: the state of the traffic light. As such, they should be treated as a single type.
In addition, each traffic light can be configured to display a color for a specific number of seconds. This allows us to adjust timing for different intersections — for example, a longer green light on a busy road, or a longer yellow light on a faster road.
We can express this idea in code by defining an enum with three variants, each carrying a u8 value representing the duration in seconds:
enum TrafficLight {
Red(u8),
Yellow(u8),
Green(u8),
}
Enum names in Rust use PascalCase, where each word is capitalized with no underscores (e.g., Direction, ResultType, Status, LogLevel).
Enum variant names also use PascalCase (e.g., North, South, Success, Failure, Active, Pending).
Names of enum methods and associated functions use snake_case, which means all lowercase letters with underscores between words (e.g., default_red, describe, can_go).
We begin an enum definition with the enum keyword, followed by the enum name, and then curly braces enclosing the enum body.
Inside the body we define the enum variants. Our TrafficLight enum has three variants, each holding a u8 value for the number of seconds the traffic light should be that color.
Note that enum variants do not need to hold the same type of data. Although our TrafficLight enum variants all hold u8 values, one could hold a String, another a u8, even a struct instance!
We call the data an enum variant holds its associated data.
Notice the comma after the final variant Green. This is idiomatic in Rust and applies to many situations:
- enum variants
- struct definitions
- function arguments
- match arms
- array literals
Reasons to use a trailing comma:
- Cleaner version control diffs (only the new line changes)
- Avoids syntax errors when adding new items
- Automatically added by rustfmt, Rust’s formatting tool
Creating Enum Variant Instances
Let’s get started writing some code.
Open a shell window (Terminal on Mac/Linux, Command Prompt or PowerShell on Windows). Then navigate to the directory where you store Rust packages
for this blog series, and run the following command:
cargo new enums
Next, change into the newly created enums directory and open it in VS Code (or your favorite IDE).
Note: Using VS Code is highly recommended for following along with this blog series. Be sure to install the Rust Analyzer extension — it offers powerful features like code completion, inline type hints, and quick fixes.
Also, make sure you’re opening the enums directory itself in VS Code. If you open a parent folder instead, the Rust Analyzer extension might not work properly — or at all.
Now, open the file src/main.rs and replace its contents entirely with the following code:
#[derive(Debug)]
enum TrafficLight {
Red(u8),
Yellow(u8),
Green(u8),
}
fn main() {
let red_light = TrafficLight::Red(30);
let yellow_light = TrafficLight::Yellow(5);
let green_light = TrafficLight::Green(20);
println!("{:?}", red_light);
println!("{:?}", yellow_light);
println!("{:?}", green_light);
}
Notice how we use the :: path separator to create an instance of an enum variant: TrafficLight::Red(30)
⚠️ Important: Enum definitions must be written outside all functions. They can appear above or below the main()
function, but not inside it.
We define our TrafficLight enum with three variants: Red, Yellow, and Green. Each one holds a u8 value, representing the number of seconds that color should be shown.
In main(), we construct an instance of each variant and print them:
Red(30)
Yellow(5)
Green(20)
Rust provides two useful formatting placeholders for printing custom types like enums and structs:
- {:?} — standard debug output
- {:#?} — pretty-printed debug output (more readable for complex data)
For example, printing a struct like this:
#[derive(Debug)]
struct Customer {
first_name: String,
last_name: String,
email: String,
phone: String,
}
Would look like this with {:?}
:
Customer { first_name: "Alice", last_name: "Anderson", email: "alice@example.com", phone: "555-1234" }
And like this with {:#?}
:
Customer {
first_name: "Alice",
last_name: "Anderson",
email: "alice@example.com",
phone: "555-1234",
}
To enable this debug-style printing for our TrafficLight enum, we use:
#[derive(Debug)]
This tells Rust to automatically generate the code needed to implement the Debug
trait — something you could write by hand, but is well outside the scope of this post.
Rust uses the word “derive” because it’s automatically generating (or deriving) code you’d otherwise have to implement manually.
Enum Variants Instances are of the Same Type
Note that our enum variant instances are of the same type, TrafficLight. The variants of the enum are namespaced under its identifier (TrafficLight), and we use a double colon to separate the two.
let red_light = TrafficLight::Red(30);
let yellow_light = TrafficLight::Yellow(5);
let green_light = TrafficLight::Green(20);
This is useful because elsewhere in our code we might have a function that takes a TrafficLight as a parameter, and then the function takes some action based on which variant was passed.
fn change_light(current: &TrafficLight) -> TrafficLight {
match current {
TrafficLight::Red(_) => TrafficLight::Green(20),
TrafficLight::Green(_) => TrafficLight::Yellow(5),
TrafficLight::Yellow(_) => TrafficLight::Red(30),
}
}
We can call this function with any of the variants:
let next = change_light(TrafficLight::Red(30));
let next = change_light(TrafficLight::Green(30));
let next = change_light(TrafficLight::Yellow(30));
Matching on Enums
The example function change_light() above takes a reference to a TrafficLight, checks the variant, and returns a different TrafficLight based on the parameter variant.
The variant check is performed with a Rust match construct. The match is used extensively in Rust. Let’s analyze what it’s doing in the change_light() function.
enum TrafficLight {
Red(u8),
Green(u8),
Yellow(u8),
}
fn change_light(current: &TrafficLight) -> TrafficLight {
match current {
TrafficLight::Red(_) => TrafficLight::Green(20),
TrafficLight::Green(_) => TrafficLight::Yellow(5),
TrafficLight::Yellow(_) => TrafficLight::Red(30),
}
}
The current function parameter is a reference to a TrafficLight variant instance.
We pass a reference to the function because we only need to read the value—we don’t need to take ownership of it. By borrowing it (using &TrafficLight), we avoid invalidating the original instance, allowing it to be used after the function call.
We match on the TrafficLight instance named current. Here is a simplified description of what the match construct does:
match VALUE {
PATTERN1 => RESULT1,
PATTERN2 => RESULT2,
PATTERN3 => RESULT3,
}
We match on a value. In our case it is an enum variant, but it could be any valid Rust value, even an expression that evaluates to a value.
Then we have curly braces. Inside the curly braces there are “match arms”. Each arm has a pattern to match against, a fat arrow ( => ), and a result. If a pattern matches, the match evaluates to the result. The result can be a value, an expression that evaluates to a value, even curly braces with a block of code to execute for that arm.
Here is a simple example:
let direction = "North";
let degrees = match direction {
"North" => 0,
"East" => 90,
"South" => 180,
"West" => 270,
_ => 0,
};
We have a variable direction set to a string literal. We match on the direction. If the “North” pattern matches, the match expression evaluates to 0, and that will be the value of degrees.
In Rust, match is an expression, not just a control-flow statement like in some other languages. This means it evaluates to a value, which we can assign directly to a variable, like we did with degrees.
The match value will be different for the other match arms.
Match expressions in Rust must be exhaustive, meaning all possible input values must be handled by some match arm.
For enums like our TrafficLight, the compiler knows all possible variants, so we only need to cover those. However, for types like &str, which can hold arbitrary strings, we often use _ to catch all unmatched cases.
In the match arms, we write TrafficLight::Red(_)
to match any Red
variant, regardless of its internal value. The underscore _
tells Rust to ignore that value. Our enum variants hold a value, but for the match all we are about is that the variant is Red, Green, or Yellow, the value it is holding is irrelevant to the match.
One useful technique with match arms is to capture the value we are matching on, and use it in the result code for the arm. Here we do want to make use of the value the enum variant is holding and print it out.
match current {
TrafficLight::Red(duration) => println!("{} secs", duration),
...
}
Enum Methods and Associated Functions
As with structs, you can define enum methods and associated functions in an impl (implementation) block. Add this code to your main.rs. Ensure you add this code outside the main() function.
impl TrafficLight {
// Associated function 1: default red light
fn default_red() -> Self {
TrafficLight::Red(30)
}
// Associated function 2: create light from &str (case-insensitive)
fn from_str(name: &str) -> Option<Self> {
match name.to_lowercase().as_str() {
"red" => Some(TrafficLight::Red(30)),
"yellow" => Some(TrafficLight::Yellow(5)),
"green" => Some(TrafficLight::Green(20)),
_ => None,
}
}
fn describe(&self) -> String {
match self {
TrafficLight::Red(secs) => format!("Red: stop for {}s", secs),
TrafficLight::Yellow(secs) => format!("Yellow: caution for {}s", secs),
TrafficLight::Green(secs) => format!("Green: go for {}s", secs),
}
}
fn can_go(&self) -> bool {
matches!(self, TrafficLight::Green(_))
}
}
We first define an associated function default_red() that takes no parameters and returns a Red variant instance with a value of 30 (seconds).
We then define an associated function from_str() that takes a string slice ( &str), matches on the lowercase version of it, and returns an Option Some() variant whose value is a TrafficLight enum variant corresponding to the passed in value.
If the value passed in is anything other than red, yellow or green, the catch all placeholder _ ensures the function returns an Option enum None variant.
We’ll discuss the Rust Option enum in a later section of this post.
We then define a method describe() that takes &self and matches on it to return a String describing the variant &self represents.
Remember that methods defined on an enum are executed in the context of an enum variant instance. So &self passed in automatically to the method will be one of these:
TrafficLight::Red(u8)
TrafficLight::Green(u8)
TrafficLight::Yellow(u8)
Notice that the match arms make use of the u8 value the variant holds in the resulting formatted string:
TrafficLight::Red(secs) => format!("Red: stop for {}s", secs)
Also notice that the method does not need a match arm with the catch all _ because the three match arms handle all possible values that can be passed to the method.
Finally, the can_go() method takes &self and uses the matches! macro to check if self is the Green variant.
The matches! Rust macro is shorthand for a simple pattern match that returns true or false.
The underscore means the value the variant is holding can be ignored.
matches!(self, TrafficLight::Green(_))
🔎 In cases where we only care about a single variant, like checking for a green light, Rust offers a convenient syntax called if let
. We’ll dive deeper into this in a future post, but here’s a quick peek:
let light = TrafficLight::Green(25);
// Use if let to check and extract the value from the Green variant
if let TrafficLight::Green(seconds) = light {
println!("Green light for {} seconds", seconds);
} else {
println!("The light is not green");
}
We create an instance of the Green variant, and then we use the if – let construct rather than a more verbose match because we only care about the Green variant.
if-let
is a shorthand for matching one variant and ignoring others. However it does have limitations, it is not exhaustive, can’t handle multiple patterns unless nested.
🧠 What this does:
if let TrafficLight::Green(seconds) = light checks if light is the Green variant.
If it is, it extracts the seconds value and runs the println!.
If not, it falls to the else block.
Comprehensive Example with a struct
Let’s wrap up the TrafficLight example with a struct making use of the enum. Replace the entire main.rs file contents with the following. Note #[derive(Clone)]
is useful if you want to reuse or copy enum instances without moving them.
#[derive(Debug, Clone)]
enum TrafficLight {
Red(u8),
Yellow(u8),
Green(u8),
}
impl TrafficLight {
// Associated function 1: default red light
fn default_red() -> Self {
TrafficLight::Red(30)
}
// Associated function 2: create light from &str (case-insensitive)
fn from_str(name: &str) -> Option<Self> {
match name.to_lowercase().as_str() {
"red" => Some(TrafficLight::Red(30)),
"yellow" => Some(TrafficLight::Yellow(5)),
"green" => Some(TrafficLight::Green(20)),
_ => None,
}
}
fn describe(&self) -> String {
match self {
TrafficLight::Red(secs) => format!("Red: stop for {}s", secs),
TrafficLight::Yellow(secs) => format!("Yellow: caution for {}s", secs),
TrafficLight::Green(secs) => format!("Green: go for {}s", secs),
}
}
fn can_go(&self) -> bool {
matches!(self, TrafficLight::Green(_))
}
}
struct TrafficLightState {
current: TrafficLight,
}
impl TrafficLightState {
fn new() -> Self {
TrafficLightState {
current: TrafficLight::default_red(),
}
}
fn next_light(current: &TrafficLight) -> TrafficLight {
match current {
TrafficLight::Red(_) => TrafficLight::Green(20),
TrafficLight::Green(_) => TrafficLight::Yellow(5),
TrafficLight::Yellow(_) => TrafficLight::Red(30),
}
}
fn advance(&mut self) {
self.current = TrafficLightState::next_light(&self.current);
}
fn show(&self) {
println!("Current light: {} | Can go? {}", self.current.describe(), self.current.can_go());
}
}
fn main() {
let mut state = TrafficLightState::new();
for _ in 0..3 {
state.show();
state.advance();
}
// Using the new from_str() associated function
if let Some(custom_light) = TrafficLight::from_str("Green") {
println!("Custom light from string: {} | Can go? {}", custom_light.describe(), custom_light.can_go());
}
}
Here we’re adding a TrafficLightState struct with a single field of type TrafficLight. The struct implementation block includes a constructor associated function that calls the TrafficLight default_red() associated function to default the TrafficLightState current field value to the Red variant.
The an associated function next_light() is defined, taking a reference to a TrafficLight. The match ensures the next logical variant is returned. So if Red then return Green, if Green then return Yellow, if Yellow then return Red.
This function is used by the method advance() which takes self and calls next_light(), passing the TrafficLightState current value. The return value of next_light() ensures the TrafficLightState current field value advances as expected:
- Red -> Green
- Green -> Yellow
- Yellow -> Red
Finally, a show() method makes use of the TrafficLight describe() and can_go() methods to print a message reflecting the current state of the TrafficLight.
The main() function creates an instance of the TrafficLightState struct, and then uses a for loop to show the state and advance the state of the TrafficLight several times.
At the end of main() we see an example of using the if – let construct to check a single enum variant.
The Option enum
The Option
enum is part of the Rust standard library. It has two variants, Some and None. The Some variant represents the presence of a value and the None variant represents the absence of a value.
Try to get the first item from a non-empty collection—you get something. Try the same with an empty list—you get nothing.
Many programming languages have the concept of null, and a variable can have a value that is null or non-null.
Rust avoids using null
to represent the absence of a value. Instead, it uses the Option
enum, which has two variants: Some(T)
, meaning a value is present, and None
, meaning no value is present. Here’s how it’s defined in the standard library:
enum Option<T> {
None,
Some(T),
}
The Option enum is used so much in Rust that it is included in the prelude. The prelude in Rust includes features that you can use in any program, without importing them.
The T
is a generic type parameter, allowing Option
to wrap values of any type. So Option<i32>
can either be Some(i32)
or None
. Generics is a large topic that will be covered in a post some time in the future.
So if you define a variable like this:
let int_option: Option<i32>;
Then you’re saying the data type of this variable will be an Option enum instance that will hold an i32 value.
The value will be held in the Some<i32> variant:
fn main() {
let int_option: Option<i32>;
int_option = Some(25);
println!("{:?}", int_option);
}
In the above code we declare a variable of type Option<i32> but we don’t assign it a value. Then we assign it the Some(25)
variant of the Option
enum. Then we print out the variable:
Some(25)
Go ahead and replace the code in main.rs with the above code so you can follow along.
At this point, the variable holds a Some
variant. If we want to print the actual value that the variant holds, we need to unwrap the variant:
println!("{:?}", int_option.unwrap());
25 // output in shell window
Note that .unwrap()
is generally discouraged in real-world code unless you’re absolutely sure the value isn’t None. Safer methods like match
, if-let
, .unwrap_or()
, .expect()
and the ?
operator exist.
Now replace the code in main.rs with this:
fn main() {
let int_option: Option<i32> = None;
println!("{:?}", int_option);
}
None // output in shell window
Here we define the variable to be of type Option<i32> but we immediately assign it a value of None. That’s fine, it just means the variable has no value. Perhaps we’ll update the value later to an i32, but for now there is no value, the value is the Option None variant.
If you try to unwrap() the variable:
println!("{:?}", int_option.unwrap());
You’ll get an error in the shell window:
thread 'main' panicked at src/main.rs:3:33:
called `Option::unwrap()` on a `None` value
panic
means the Rust program crashed and terminated.
This is an example of the Rust safety feature. If the variable doesn’t have a value, then accessing it doesn’t make sense, and in some other programming languages it could be unsafe. So Rust doesn’t allow it. The compiler knows the variable is currently an instance of the None variant of Option, so it doesn’t let you unwrap it.
So why doesn’t Rust have a null value? Why does it instead have Option<T>
?
Well, null values are unsafe and can cause unpredictable behavior at runtime. The compiler won’t let you use a variable that has been declared but not initialized with a value.
Option<T>
and its variants Some()
and None
are a Rust construct that allows you to represent situations where there might be a value, or a value might be absent.
Here’s when Option<T>
makes sense:
- Values that might not be set yet (e.g., lazy initialization)
- Function return types that might fail (e.g.,
find
,get
, etc.) - Nullable fields (e.g., database fields)
- Handling user input that might not be provided
Pattern Matching with Option
We often do pattern matching on values that are of type Option<T>:
match x {
Some(val) => println!("Value is {}", val),
None => println!("No value"),
}
We check if we have a value, and if so we proceed. If we have no value we do something else.
The Option
enum has many useful methods:
is_some()
,is_none()
unwrap()
,expect()
(with caveats)unwrap_or()
,unwrap_or_else()
map()
,and_then()
(monadic chaining)ok_or()
,ok_or_else()
(converting toResult
)
You’ll see these methods used in future posts, including a future post dedicated to examining the Option
enum in-depth.
So use Option<T>
when a value might or might not exist, but don’t overuse it. As you gain experience with Rust you’ll see Option<T>
returned from many built-in Rust methods. Use match
or one of the built-in Rust methods and avoid unwrap()
in most cases.
Now let’s turn our attention to another enum included in the Rust prelude
, the Result
enum.
The Result enum
The Result
enum is also in the Rust standard library. It has two variants, Ok()
and Err
.
The Ok()
variant indicates an operation was successful, and it contains the value generated by the operation. The Err
variant indicates the operation failed, and contains information about what went wrong, such as errors.
Like the Option
enum, the Result
enum has methods defined on it, such as the .expect()
method. If the instance of Result
is an Err
, then expect()
will crash the program and will display the error message you passed to expect()
.
If the instance of Result
is Ok()
, expect()
will return the actual value that Ok()
is holding.
Some operations, like reading a line from a file, return a Result
with the line that was read or an Err
. The Rust compiler will give a warning if you fail to properly handle the Result
, including an Err
if one occurred.
Here is the definition of the Result
enum in the standard library:
enum Result<T, E> {
Ok(T),
Err(E),
}
The T
and E
are also generics. T
is the type of value that will be returned in a success case in the Ok
variant, and E
is the type of error that will be returned in the Err
variant on failure.
Replace the code in main.rs with the following code:
use std::fs::File;
fn main() {
let greeting_file_result = File::open("hello.txt");
let greeting_file = match greeting_file_result {
Ok(file) => file,
Err(error) => panic!("Problem opening the file: {error:?}"),
};
}
This code imports the standard library File
module, located in the fs
module. We’ll discuss the Rust module system in a future post, but for now we’ll discuss modules we need to import as necessary.
In this code we call the open()
associated function, to open a file named hello.txt.
If you run the program using cargo run
, Rust will look for file hello.txt in the current working directory, which is the parent directory of the src directory. If you run the program with the generated executable, then the working directory is the folder you are in when you run the program in the shell window.
The return type of File::open
is a Result<T, E>
. T will be a std::fs::File
, which is a file handle to the opened file on success. E will be a std::io::Error
on failure.
The open operation might fail because the file doesn’t exist, or because we don’t have permissions to open the file.
This is the purpose of the Result<T, E>
enum. It informs us if an operation succeeded or failed.
We use a match
on the result of the operation, with the two arms handling the Ok(file)
success case and the Err(error)
failure case. On success, variable greeting_file will be set to the file handle returned by the match
, on failure, we use the panic!
macro to terminate the program with the specified error message.
Execute the program with cargo run. If file hello.txt does not exist you should get this error:
thread 'main' panicked at src/main.rs:8:23:
Problem opening the file: Os { code: 2, kind: NotFound, message: "No such file or directory" }
If file hello.txt does exist you should get no errors, though we’re currently not doing anything with the returned file handle.
Nested Match Expressions
Our current code will panic!
regardless of the type of error causing the failure. Replace it with this code, which responds differently depending on the error:
use std::fs::File;
use std::io::ErrorKind;
fn main() {
let greeting_file_result = File::open("hello.txt");
let greeting_file = match greeting_file_result {
Ok(file) => file,
Err(error) => match error.kind() {
ErrorKind::NotFound => match File::create("hello.txt") {
Ok(fc) => fc,
Err(e) => panic!("Problem creating the file: {e:?}"),
},
other_error => {
panic!("Problem opening the file: {other_error:?}");
}
},
};
}
This code makes use of two nested match
expressions, to match on the error with the kind()
method. If the error is of type ErrorKind::NotFound
then we call File::create()
to create the file. We match again on the result of create()
.
Nested match
expressions are another important tool as you move toward Rust mastery.
Although we won’t go into detail, the following is a more concise way to handle the above situation without nested match
expressions. It uses the unwrap_or_else()
method:
use std::fs::File;
use std::io::ErrorKind;
fn main() {
let greeting_file = File::open("hello.txt").unwrap_or_else(|error| {
if error.kind() == ErrorKind::NotFound {
File::create("hello.txt").unwrap_or_else(|error| {
panic!("Problem creating the file: {error:?}");
})
} else {
panic!("Problem opening the file: {error:?}");
}
});
}
Similar to Option
, Result
also has the unwrap()
and expect()
methods, with Rustaceans generally preferring the expect()
method.
Propagating Errors to the Caller
Sometimes rather than handle an error as it occurs you want to return the error to the calling scope, and let it handle the error:
use std::fs::File;
use std::io::{self, Read};
fn read_username_from_file() -> Result<String, io::Error> {
let username_file_result = File::open("hello.txt");
let mut username_file = match username_file_result {
Ok(file) => file,
Err(e) => return Err(e),
};
let mut username = String::new();
match username_file.read_to_string(&mut username) {
Ok(_) => Ok(username),
Err(e) => Err(e),
}
}
Here the function returns errors that occur, rather than handling them as they occur.
? as a Shortcut for Propagating Errors
use std::fs::File;
use std::io::{self, Read};
fn read_username_from_file() -> Result<String, io::Error> {
let mut username_file = File::open("hello.txt")?;
let mut username = String::new();
username_file.read_to_string(&mut username)?;
Ok(username)
}
This code uses the ?
operator, which works similar to a match
expression. If the Result
is success then ?
will return the Ok()
value. On failure ?
will return from the whole function with the error as if we had a used the return
keyword.
You can use the ?
operator in functions that return a Result
or Option
type.
Wrapping up…
That wraps up our look at enums in Rust, and the Option
and Result
enums from the Rust standard library.
You’ll see Option
and Result
used in future posts, including future deep dive posts on Option
and Result
.
Thanks for stopping by, and for letting ByteMagma be a part of your journey toward Rust mastery!
Leave a Reply