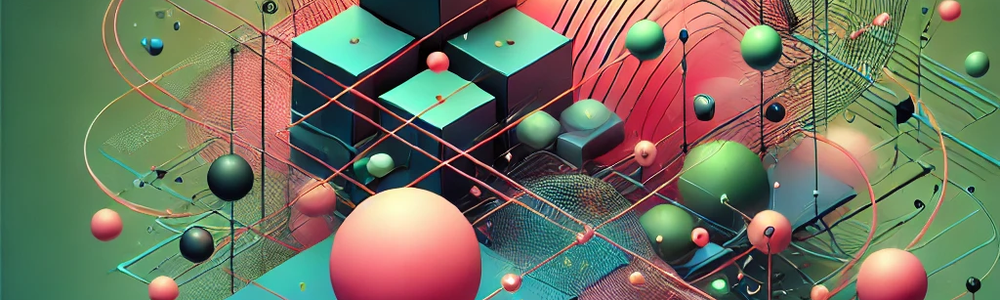
As you start learning Rust and writing some simple programs, you likely hit some issues around ownership, moving, borrowing, and references. This post takes a deep dive into ownership and these associated topics.
If you’re just getting started with Rust, these posts earlier in this series on Rust programming may be helpful:
Setting Up Your Rust Development Environment
Mutability, Copying, and Why Some Things Just Vanish
Functions in Rust – The Building Blocks of Reusable Code
Introduction
One important feature of Rust that sets it apart from other systems languages such as C and C++ is the promise of memory safety without a garbage collector.
Most modern programming languages use a garbage collector to manage memory. The runtime is constantly watching what you’re doing, cleaning up unused memory in the background.
Rust takes a very different approach. It doesn’t have a garbage collector, but it still guarantees memory safety.
To do this Rust performs compile-time checks using a system of ownership and lifetimes. The compiler enforces rules that prevent:
- Dangling pointers (accessing memory that’s been freed)
- Data races (multiple threads modifying data simultaneously)
- Double frees (freeing memory more than once)
- Use-after-free bugs (using memory after it’s been released)
And yet you don’t have to manually manage memory as you do in C or C++. Rust ensures these safety rules are followed before your code even runs, at compile-time.
This leads to:
- Faster programs (no garbage collector pauses)
- Predictable performance
- Fewer runtime crashes
Rust gives you the control of a low-level language with the safety of a high-level one — and does it through its unique approach to memory management.
This post covers ownership, moves, borrowing, references, and how data lives in stack vs heap.
Memory Layout: Stack vs Heap
As your Rust program runs, data is stored in memory on the stack and the heap. Let’s compare the stack and the heap.
Stack
The stack is often described as a stack of dishes. You add data to the top of the stack just like you’d place a dish on top of others.
- Dishes are removed from the top of the stack, and data works the same way.
- This is known as LIFO — Last In, First Out — meaning the most recently added data is removed first.
- Because of this orderly behavior, the stack is fast for both adding and removing data.
- The stack stores data of known, fixed size — like integers, floats, and booleans.
- Stack data is automatically managed. When a variable goes out of scope, its memory is popped off the stack — no manual cleanup needed.
Heap
The heap is more like your garage or a warehouse.
- If you need to store something, you find an open space — it’s not as orderly as the stack.
- This flexibility allows you to store data of unknown size at compile time — like growable strings (String) or vectors (Vec).
- Because the heap is less structured, accessing or modifying heap data is generally slower than working with the stack.
- Data on the heap doesn’t automatically clean itself up — something needs to track it and free it. In Rust, the owner of the data is responsible for that.
Why This Matters
Rust gives you control over whether data lives on the stack or heap — and that affects:
- Performance
- Memory safety
- Whether a variable can be moved, borrowed, or cloned
We’ll explore all of this step-by-step. But first, let’s look at a visual depiction of how stack and heap memory are laid out in a running Rust program.
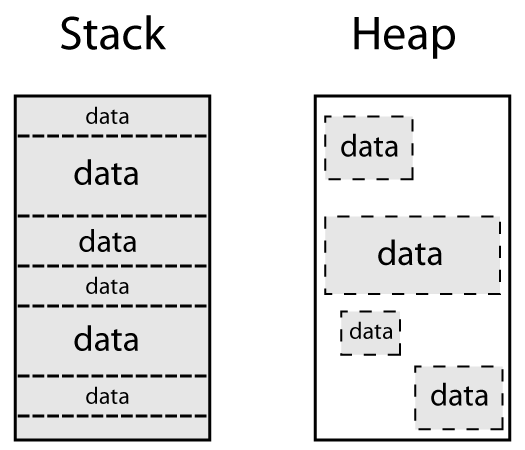
We see that stack data is like that stack of dishes, with the data most recently added on the top.
Contrast that with heap data, which is stored here and there in memory. The data in the bottom right of this heap depiction may have been stored most recently.
What is Ownership?
Ownership is what allows Rust to provide superior memory safety compared with other programming languages.
Rust’s three ownership rules:
- Each value in Rust has a single owner. Usually this is a variable, but ownership can also belong to function parameters, struct fields, or return values.
- A value can only have one owner at a time. Ownership can be moved to another variable, but two variables cannot own the same value simultaneously. Other variables can borrow the value, but that doesn’t transfer ownership.
- When the owner goes out of scope, the value is dropped. Rust automatically calls drop() to clean up the value — freeing memory, closing files, etc.
Ownership in Action
Let’s write some code as we examine ownership, moves, borrowing, copying, references, etc.
Let’s get started writing some code. Open a shell window (Terminal in Mac/Linux, Cmd or PowerShell in Windows), and CD into the directory where you store Rust packages
for this blog and execute this command:
cargo new ownership_borrowing
Now CD into the ownership_borrowing directory and open that directory in VS Code or your favorite IDE.
Note: using VS Code will make it easier to follow along with our posts. And installing the Rust Analyzer extension is also beneficial.
Also, opening the directory containing your Rust package
allows the Rust Analyzer VS Code extension to work better. If you open a parent folder in VS Code the Rust Analyzer extension might not work at all. So open the ownership_borrowing directory in VS Code.
Open the file src/main.rs and replace its contents entirely with this code:
fn main() {
let s1 = String::from("apple");
let s2 = s1;
println!("{s1}"); // s1 no longer valid, its value moved to s2
}
We define variable s1 whose value is a String containing the text “apple”. Then we define variable s2, assigning s1 to it. Then we attempt to print out the value in variable s1.
Now execute this in the shell window:
cargo run
This results in the following error. You can also see this error in VS Code if you have the Rust Analyzer extension installed, by hovering over the red squiggly line under s1.
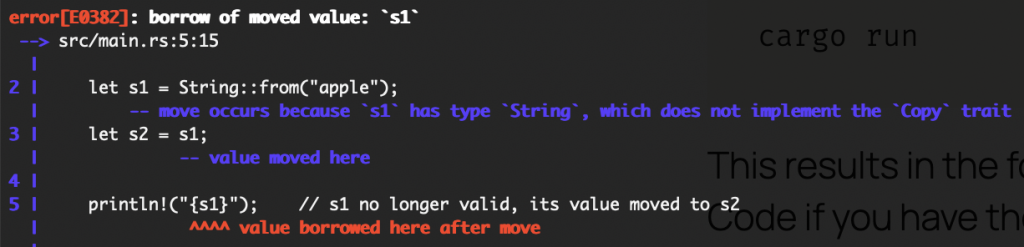
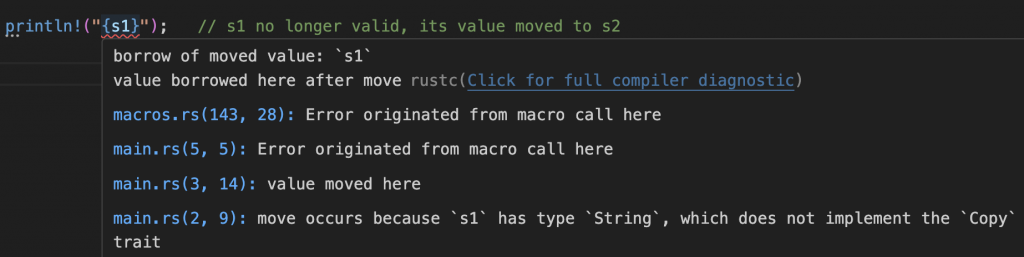
The error tells us we’re trying to use a value after it’s been moved, which is not allowed.
This error occurs because when we assigned s1 to s2, the value in variable s1 was moved to variable s2.
Remember, one of the Rust ownership rules is that each value can have only one owner. So when we assigned s1 to s2, ownership of the String “apple” was transferred (moved) to s2.
This makes variable s1 invalid, so when we try to use it in the println!() statement, we get a compile error. The compiler is protecting us, preventing us from using invalid data.
Note that if the value in s1 were a primitive data type, such as an i32 integer, we wouldn’t get an error:
fn main() {
let s1 = 10;
let s2 = s1;
println!("{s1}");
}
This is because primitive data types implement the Copy trait, so their default behavior in this situation is to have their values copied, not moved. The Copy trait allows values to be duplicated automatically, without moving ownership.
We’ll cover traits in a post some time in the future.
But data types such as String, Vec, Box, etc. do not implement the Copy trait, and their default behavior in this situation is to move ownership, not to copy the value.
We’ll see how to fix this issue with a reference in a bit, but first let’s see how this String data is represented in the stack and in the heap.
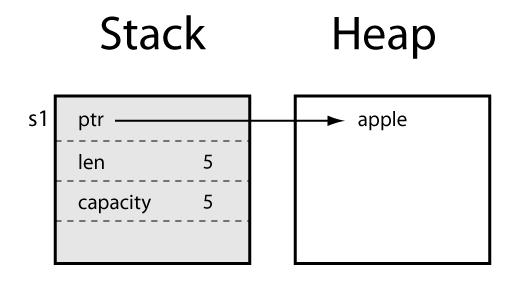
When we define a variable s1 whose value is the String “apple”, three pieces of data are stored on the stack:
A pointer to the heap, where the actual string data “apple” is stored
The length of the string (how many bytes are currently used)
The capacity of the string (how many bytes are allocated on the heap)
In this case, both the length and capacity are 5, and the pointer points to the memory address on the heap where the characters “a”, “p”, “p”, “l”, and “e” are stored.
The variable itself (e.g., s1) is stored on the stack, but the actual string data lives on the heap. This is how Rust handles growable, dynamically sized data while keeping stack allocations small and predictable.
Now let’s see what happens in the stack and the heap when we assign s1 to s2:
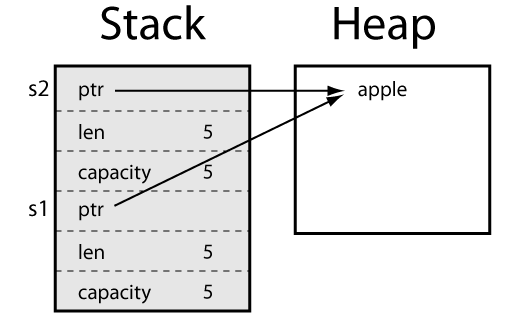
When s1 is assigned to s2, a copy of the stack data from s1 — including the pointer, length, and capacity — is placed on the stack for s2. It is added at the top of the stack.
The pointer fields for both s1 and s2 point to the same location on the heap, where the actual string “apple” is stored.
The ptr, len, and capacity fields for s1 are not removed from the stack immediately when ownership is moved to s2.
At compile-time, s1
is considered invalid and you can’t use it. But at runtime, the memory may still be present on the stack until it’s cleaned up.
However, Rust’s compiler treats s1 as invalid after the move, meaning you cannot use s1 again in your code — it will cause a compile-time error.
At runtime, the memory location for s1 may still exist on the stack until the scope ends (e.g., when main() finishes), but it’s not safe or legal to access it anymore — the compiler ensures that.
Fixing the Error
One way to fix the ownership error is to clone the data instead of assigning s1 directly to s2. Execute cargo run again and there is no error, and apple is printed to the shell window.
fn main() {
let s1 = String::from("apple");
let s2 = s1.clone();
// s1 still valid, its value was cloned, not moved
println!("{s1}");
}
Let’s look at the stack and heap after cloning.
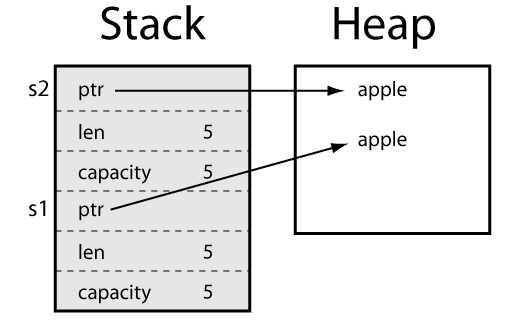
- A new heap allocation is made for s2.
- The characters “apple” are copied into it.
- On the stack, s2 gets its own pointer, length, and capacity — separate from s1.
This works and avoids the move error, but cloning creates a duplicate on the heap, which isn’t always efficient.
A better solution is often to borrow the data using references, which we’ll cover next.
A Better Solution – References
We’re not actually making use of variable s2, but I still want to show you how to fix the ownership error with a reference. Then I’ll show you a more practical example with the same error, and also fixing the error using a reference.
Replace the code in main.rs with the following:
fn main() {
let s1 = String::from("apple");
let s2 = &s1;
// s1 still valid, its value was borrowed, not moved
println!("{s1}");
}
In this version, instead of assigning s1 to s2, we assign an immutable reference to s1:
let s2 = &s1;
This means that s2 doesn’t take ownership of the string — it simply borrows it. As a result, s1 remains valid and usable, because nothing has been moved.
A reference is created by placing an ampersand before the variable name: &s1
We’ll discuss immutable and mutable references soon.
What is a Reference?
When we assign an immutable reference to s2:
let s2 = &s1;
The value of variable s2 is a reference to the data structure representing variable s1 on the stack, a pointer to the data for s1 on the stack:
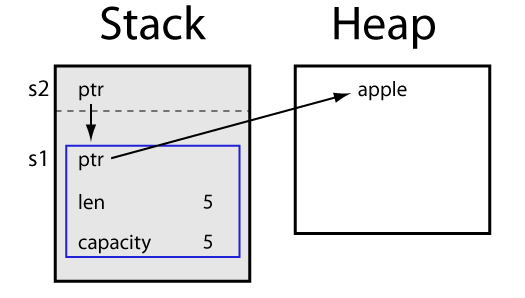
This is how s2 borrows the value of s1 rather than taking ownership via a move.
A More Practical Example
Our current code uses a reference to avoid the ownership move error. But in that example, we didn’t actually use the reference — we just created it and printed s1.
Let’s look at a more practical example involving a function we define. To start, we won’t use a reference and instead pass a String directly to a function:
fn main() {
let s1 = String::from("apple");
// We pass s1 by value — this is a move
display_a_fruit(s1);
// ❌ Error: s1 is now invalid, because its ownership was moved
println!("{s1}");
}
fn display_a_fruit(s: String) {
// s takes ownership of the String
println!("The fruit: {}", s);
}
This code defines a variable s1 as a String containing “apple”.
Then we define a function display_a_fruit that takes ownership of a String parameter. This happens because String
does not implement the Copy
trait, and it’s passed by value.
When we call the function and pass s1, its ownership is moved into the function — which means s1 is no longer valid in main() after the function call. That’s why we get a compile-time error when trying to use s1 again.
This is similar to the earlier example where we assigned s1 to s2, causing a move and invalidating s1.
In Rust, function parameters of types that do not implement the Copy trait (like String) are moved by default when passed by value.
Once again, we can fix the ownership issue by using an immutable reference.
Change the code so we pass an immutable reference to s1 as the function parameter:
fn main() {
let s1 = String::from("apple");
// We borrow s1 immutably, so ownership is not moved
display_a_fruit(&s1);
// s1 is still valid here, because we only borrowed it
println!("{s1}");
}
fn display_a_fruit(s: &String) {
// We're borrowing the String immutably.
// That means we can look at it, but we can't change it.
println!("The fruit: {}", s);
}
Save the file and execute cargo run in the shell window. You should get no errors and see this output:
The fruit: apple
apple
We pass an immutable reference to variable s1 to the function:
&s1
The function signature specifies that it takes a single, immutable reference to a String:
fn display_a_fruit(s: &String)
We use the reference inside the function body to print the passed in String. Because we passed a reference, ownership was not moved into the function. Instead, the function borrows the variable s1, and ownership is not transferred. So variable s1 is still valid back in main() after the function call.
Immutable and Mutable References
So far we’ve used immutable references, but what does that mean? An immutable reference results in a borrow that allows us to view the underlying data, but we can’t change the data.
A mutable reference is also a borrow, but we can actually change the data the reference points to, in addition to viewing the data.
Note that the variable used to create the reference must also be mutable. If you’re new to Rust and new to mutability, this ByteMagma post on mutability might be a good starting point, then you can come back:
Mutability, Copying, and Why Some Things Just Vanish
Replace the code in main.rs with the following code:
fn main() {
// message is mutable, so we can change it
let mut message = String::from("Hello");
// We borrow message mutably, so ownership is not moved
create_message(&mut message);
// message is still valid here, because we only borrowed it
println!("{message}");
}
// we take a mutable reference to a string
fn create_message(msg: &mut String) {
// We're borrowing the String mutably.
// That means we can look at it, and we can change it.
msg.push_str(" world");
}
Execute cargo run. There are no errors, and this is the output:
Hello world
We define a String variable message with the text “Hello”. The variable is made mutable by placing the mut keyword before the message variable identifier, meaning we can change it.
We also define a function create message that takes a mutable reference to a String.
fn create_message(msg: &mut String)
The reference is mutable because we placed the mut keyword after the ampersand.
Inside the function, we mutate the String pointed to by the mutable reference, adding a space and the word “world” to the string.
Back in main()
, we print the message
variable, confirming that the mutation occurred.
Wrapping Up…
In this post, we’ve seen situations in which ownership of data was moved to another variable, making the original variable invalid and unusable.
We then saw how we could avoid this using an immutable reference, which resulted in a borrow of the original variable data, with no transfer (move) of ownership.
We extended this concept to function parameters, first seeing the move error case, in which we passed a variable to the function. The function took ownership with a move, and the original variable back in main() was rendered invalid and unusable.
Finally, we saw how passing an immutable reference to the function solved the move error, instead resulting in a borrow. And we saw using a mutable reference to a mutable variable to modify the data inside the function, verifying the changed data back in main().
Ownership, move, borrowing, copying, and immutable and mutable references are concepts frequently seen in Rust code. We’re just getting started with these important concepts, and will expand on them in upcoming posts.
Thank you so much for stopping by, and for including ByteMagma in your journey to mastering Rust programming!
Leave a Reply